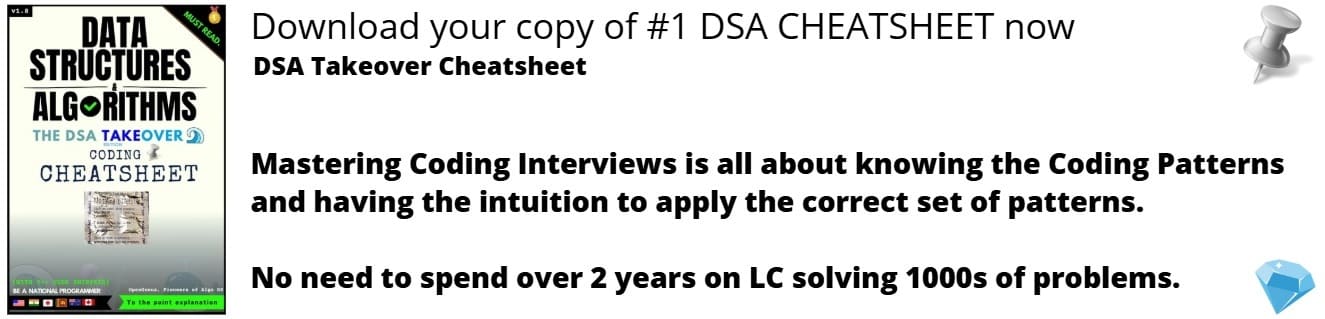
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
OOPs, or Object-Oriented Programming is a programming model or paradigm which revolves around the concept of "objects" that mimic real-life entities. It is widely used and is a concept adapted by most of the popular programming languages.
Questions about OOP concepts are asked in every organization during interviews, be it product-based or service based. This article covers the most important questions you need to crack any technical round.
Table of Content
- Multiple Choice Questions
- Descriptive Questions
- Practical Questions
Multiple Choice Questions
1. Which is not an oops concept?
a. Inheritance
b. Compilation
c. Polymorphism
d. Encapsulation
Ans: Option b. Compilation
Explanation: Compilation does not come under the 4 core concepts of OOP - Abstraction, Polymorphism, Encapsulation, Inheritance.
2. Why is Java considered a partial OOP language?
a. Code can be written outside classes
b. It supports primitive data types
c. It does not support pointers
d. It does not support all types of inheritance
Ans: Option b. It supports primitive data types
Explanation: Java is considered a partial implementation of OOP since it supports primitive datatypes instead of using objects of these types.
3. Which feature of OOP indicates code reusability?
a. Abstraction
b. Polymorphism
c. Encapsulation
d. Inheritance
Ans: Option d. Inheritance
Explanation: In Inheritance, the subclass (or child class) inherits the properties of its superclass (or parent class). This indicates reusability as the same code can be used in subclasses of a superclass.
4. Which of the following was the first purely object oriented programming language developed?
a. Kotlin
b. SmallTalk
c. Java
d. C++
Ans: Option b. SmallTalk
Explanation: The first entirely object-oriented programming language was called SmallTalk, created by Alan Kay in the 1970s.
5. The feature by which one object can interact with another object is
a. Message reading
b. Message Passing
c. Data transfer
d. Data Binding
Ans: Option b. Message Passing
Explanation: All communication between processes or objects is done through message passing.
6. Which of the following is the correct syntax of constructor?
a. –Classname()
b. Classname()
c. ()Classname
d. ~Classname()
Ans: Option b. Classname()
Explanation: The constructors only contains the class name. The class name is followed by the parenthesis - with or without arguments. Constructor does not return any value.
7. Which of the following type of members can not be accessed in a subclass?
a. Protected
b. Private
c. Public
d. All can be accessed
Ans: Option b. Private
Explanation: Private members of a class can only be accessed within the class in which it is declared.
8. Where is the memory allocated for the objects?
a. Cache
b. ROM
c. HDD
d. RAM
Ans: Option d. RAM
Explanation: The allocation of memory for the objects or any other data occurs initially in RAM.
9. If data members are private, what can we do to access them from the class object?
a. It can never be accessed from outside the class
b. It can be accessed by creating public member functions
c. It can be accessed by creating private member functions
d. It can be accessed by creating protected member functions
Ans: Option b. It can be accessed by creating public member functions
Explanation: To get access to the private data members and obtain their values for usage or modification, we can build public member functions. They cannot be accessed directly, however member functions can be used to access them. To prevent unintentional modification of the confidential data, this is done.
10. Which among the following is not a necessary condition for constructors?
a. The name of the constructor must be same as that of its class
b. The constructor must not return any value
c. It must have a definition body
d. It must contain arguments as parameters
Ans: Option c. It must have a definition body
Explanation: Even if not explicitly defined, constructors are nonetheless predefined implicitly. It is not required for a constructor to have any definitions.
11. Instance of which type of class can not be created?
a. Parent class
b. Abstract class
c. Anonymous class
d. Nested class
Ans: Option b. Abstract class
Explanation: Java does not allow us to instantiate abstract classes since they are not concrete and therefore can not be used. Abstract class can only be inherited.
12. Assume that class B inherits from class A and class C is a subclass of class B. If class C is instantiated, which of the following will be the sequence in which the destructors will be called?
a. ~A(), ~B(), ~C()
b. ~C(), ~A(), ~B()
c. ~C(), ~B(), ~A()
d. ~B(), ~C(), ~A()
Ans: Option c. ~C(), ~B(), ~A()
Explanation: The order in which the destructors are called is the reverse of how the constructors have been called. Since class A constructor would have been called first, class A destructor will be called at the end.
13. What is the number of parameters that a default constructor requires?
a. 0
b. 2
c. 1
d. 5
Ans: Option a. 0
Explanation: Default constructor does not require any parameter.
14. Which of the following functions can be inherited from the base class?
a. Constructor
b. Static
c. Destructor
d. None
Ans: Option d. None
Explanation: None of the functions can be inherited from the base class.
15. Identify the feature, which is used to reduce the use of nested classes.
a. Binding
b. Inheritance
c. Encapsulation
d. Abstraction
Ans: Option b. Inheritance
Explanation: Through inheritance, the parent class's members are accessible to the subclass.
16. What are the total number of catch blocks that can be used using a single Try block?
a. 1
b. According to need
c. 5
d. at most 256
Ans: Option b. According to need
Explanation: Any number of catch blocks can be used for a single try block.
17. Which of following does not have a body definition?
a. An Interface
b. A Class
c. An Abstract Method
d. none of above
Ans: c. An Abstract Method
Explanation: Methods classified as abstract don't need to be implemented in order to be declared.
18. How to find out the size of a class?
a. Total size of all inherited variables and variables belonging to the same class.
b. It is the largest variable of the same class.
c. Classes not have any size.
d. The aggregation of the size of all the variables in the class.
Ans: Option c. Classes not have any size
Explanation: The class size is determined by the object size.
19. A protected member of a class is visible to
a. subclasses in other packages
b. classes in the same package
c. within the same class
d. All of the above
Ans: Option d. All of the above
Explanation: The protected methods or data members are accessible within the classes of the same package and subclasses in different packages.
20. How can the concept of encapsulation be achieved in the program?
a. By using the Access specifiers
b. By using the concept of Abstraction
c. By using only private members
d. By using the concept of Inheritance
Ans: Option a. By using the Access specifiers
Explanation: By including access specifiers, users can obtain the principle of encapsulation. The user is not required to use only private members.
Descriptive Questions
1. What is Object Oriented Programming?
Object oriented Programming (OOP) is a programming paradigm. Using this method, data and operations using the data are combined to create an object that can be used by the application. Concepts from object-oriented programming aid in translating complex real-world systems into controllable software solutions.
2. Name some languages that use OOP.
-
Popular pure OOP languages include:
Ruby
Scala
JADE
Emerald -
Programming languages designed primarily for OOP include:
Java
Python
C++ -
Other programming languages that pair with OOP include:
Visual Basic .NET
PHP
JavaScript
3. Define class.
A class is a blueprint or a set of guidelines for creating an object. It is a fundamental idea in object-oriented programming that centres on real life entities. Data and methods using the data are both contained in a class. For example, Car is a class that contains some data and operations that are common for all type of cars. All cars have attributes such as horsepower, color, price and capacity and perform basic functions such as break and accelerate.
4. Define Object.
An Object is an instance of a class. It is a real world entity that has some attributes and functions with values just specific to it. In the above example, if there is a class of Cars with the mentioned properties, then an object will be a particular model of a car. Hyundai Venue, Kia Sonet and Tata Nexon are instances of the class Car, each having data and operations of its own.
5. What are the advantages of using Object Oriented Programming languages?
- Instead of having to start writing the code from scratch, we can build programs from pre-existing working modules that communicate with one another. This increases productivity and reduces development time.
- OOP language also enables us to divide a program's problems into smaller, more manageable chunks (one object at a time).
- With the new technology, programmers should produce more work with higher-quality software at lower maintenance costs.
- OOP systems are easy to scale.
- It is feasible for different instances of the same object to coexist peacefully.
- The division of work within a project based on objects is relatively simple.
- The objects in the problem domain can be mapped to those in the program.
- The data hiding principle enables programmers to create safe programs that are impervious to code from other areas of the program. This enhances security.
- We may remove unnecessary code and increase the functionality of existing classes by using inheritance.
- Since message passing techniques are utilised for inter-object communication, it is considerably easier to describe interfaces with external systems.
6. Why is Java not considered a pure OOP language?
Java does not qualify as a pure object-oriented language because it makes use of primitive data types like (int, float, char, etc.). Although the Java developers could have created these primitive data types as objects (like String), but primitive data types are faster than objects.
The second reason is the 'static' keyword. To access methods and data, objects are utilised in object-oriented programming languages. However, static variables and methods in Java can be accessed without the use of objects.
7. What are the four pillars of OOP?
The four pillars of object oriented programming are:
- Inheritance: sub-classes derives data and methods from super-class.
- Encapsulation: wrapping data and methods in a single entity.
- Abstraction: revealing only relevant information.
- Polymorphism: methods can have many forms.
8. Define Encapsulation.
Encapsulation is the binding of data and the functions that manipulate them. It also lead to data hiding or abstraction. Through encapsulation, the data and functions are wrapped together in a single entity called a class.
9. What are the advantages of encapsulation?
- Encapsulation adds flexibility to programming. It implies that you do not need to constantly alter and update code in accordance with changing requirements.
- It provides a way to restrict access to data members, thus improving security.
- You can achieve loose coupling through encapsulation.
- Debugging and testing a program is simple and straightforward with encapsulation.
- It is also possible to change and update your codebase without impairing your program's normal operation. It improves reusability.
10. Define Polymorphism.
Polymorphism is derived from two words - Poly, which means many and morphism which means forms. Polymorphism in OOPs refers to the way that some code, data, methods, or objects act differently depending on the situation or context. A real life example would be how a person plays different roles - a mother, sister, daughter, etc. There are two types of polymorphism:
-
Compile time polymorphism: also known as Static Polymorphism, is the type of Polymorphism that occurs during compilation of the program. The compiler decides what behavior has to be taken by the object.
-
Runtime polymorphism: or Dynamic Polymorphism, is the type of Polymorphism that occurs during run time. The behavior of the entity is decided at the time of execution and can't be decided by the compiler.
11. What is Method Overloading?
A compile-time polymorphism feature called overloading allows an entity to have numerous implementations of the same name. Overloading is done in two ways - Method Overloading and Operator Overloading.
In Method overloading, several methods can share the same name but have distinct signatures, where the signature might vary based on the quantity, nature, or combination of input arguments. It is also referred to as Early Binding, Static Polymorphism, or Compile-time polymorphism.
Example:
class HelloWorld {
public static void addition(int a, int b){
System.out.println("Function 1");
System.out.println(a + b);
}
public static void addition(int a, int b, int c){
System.out.println("Function 2");
System.out.println(a + b + c);
}
public static void addition(double a, double b){
System.out.println("Function 3");
System.out.println(a + b);
}
public static void main(String[] args) {
addition(1, 2); // int a , int b
addition(1.0, 2.0); // double a, double b
addition(1, 2, 3); // int a, int b, int c
}
}
Output:
Function 1
3
Function 3
3.0
Function 2
6
The function addition has many implementations. The function to be called depends on the argument passed. The decision is made during compile time.
12. What is Method Overriding?
Method Overriding is a way to achieve run-time polymorphism. Through overriding, a subclass can provide a customized implementation of a method it has inherited from its super class. If a method in the subclass has the same name, parameters or signature and return type as that of method in its parent class, the method in the parent class is said to be overridden by the method in the child class.
The method that will be invoked is decided by the object that calls the method. When a method is called from an object of a parent class, the method's parent class version is executed; however, when a method is called from an object of a subclass, the child class version is executed.
Example:
class Parent{
void print(){
System.out.println("Parent Print");
}
}
class Child extends Parent{
void print(){
System.out.println("Child Print");
}
}
class Demo {
public static void main(String[] args) {
Parent p = new Parent();
p.print();
Parent p2 = new Child();
p2.print();
}
}
Output:
Parent Print
Child Print
13. What is Operator Overloading?
Operator overloading is an implementation of compile-time polymorphism. It is the concept of modifying an existing C++ operator without altering its original meaning. We can adapt operators in C++ to work with user-defined classes. This means that C++ has the ability to give the operators a special meaning for a particular data type. This is known as Operator Overloading. For instance, by overloading the "+" operator in a class like String, we can concatenate two strings with merely the "+" symbol.
14. What is the difference between Method Overloading and Overriding?
Method Overloading | Method Overriding |
---|---|
It is a compile-time polymorphism. | It is a run-time polymorphism. |
It helps to make the program easier to read. | It is utilized to offer a particular implementation of a method that is already available in its parent class or superclass. |
Method overloading occurs within a single class with multiple functions with the same name. | It is carried out by two classes that are related by inheritance. |
Methods must have different parameters or signatures but the same name when overloading. | Methods must share the same name and signature in different classes. |
Private and final methods can be overloaded. | Private and final methods can not be overridden. |
The return type may or may not be the same. However, the parameters must be different | Return type must be same or covariant. The parameters must be the same. |
15. Define Inheritance.
A process used to acquire a class's attributes and behaviours by another class called inheritance. The class that inherits from the base class is referred to as the derived class, while the class that inherits from the base class is known as the parent class. Derived class is also known as Child class or Sub class, whereas Base class is also known as Parent class or Super class.
Advantages:
- Code reuse is made easier through inheritance. The parent class's code may be used by the child class without having to re-write it. This saves time and effort.
- A simple and understandable model structure is provided by inheritance.
- It helps to achieve less development and maintenance costs.
- With inheritance, we are able to override base class methods so that the derived class can design a useful implementation of the base class method.
16. What are the types of Inheritance?
-
Single Inheritance: A class only derives from one base class in single inheritance. This indicates that there is just one superclass and one subclass.
-
Multi-level Inheritance: A class is derived from another derived class through multilevel inheritance. There can be as many tiers of inheritance as necessary.
-
Multiple Inheritance: In multiple inheritance, a class can derive from multiple classes.
-
Hierarchical Inheritance: In this type of inheritance, multiple classes inherit from a single base class.
-
Hybrid Inheritance: Hybrid inheritance is usually a combination of more than one type of inheritance. In the diagram given below, the hybrid inheritance is a combination of single inheritance and a hierarchical inheritance.
17. Why does Java not support Multiple Inheritance?
The ability to create a single class with many super classes is known as multiple inheritance in Java. Due to the potential for ambiguity when two subclasses share a method with the same name and signature, Java does not support multiple inheritances in classes. Think of a scenario where Class B extends Class A and Class C, and Class A and Class C both have the same method void display(). The display method the subclass should inherit cannot be determined by the Java compiler.
18. What can be done to implement multiple inheritance in Java?
Using multiple interfaces in a class is the only way to implement multiple inheritance. Java allows one class to implement multiple interfaces. Due to the fact that all methods stated in interfaces are implemented in classes, ambiguity is avoided.
interface Interface1
{
public void show();
}
interface Interface2
{
public void show();
}
class SubClass implements Interface1, Interface2
{
public void show()
{
System.out.println("A class can implements more than one interfaces");
}
public static void main(String args[]){
SubClass obj = new SubClass();
obj.show();
}
}
19. What is Abstraction?
Abstraction hides implementation details while revealing required functionality to other objects. To put it another way, it is the process of keeping an application's internal workings hidden from the outside world so that we only need to understand what the code does and not how it does it. A real life example of abstraction would be learning how to drive a car. While learning, a person does not need to know the internal working of the mechanism but only the necessary process to drive a car.
20. How is abstraction achieved in Java?
Interfaces and abstract classes help us achieve Abstraction in Java. Although, abstract classes only provide partial abstraction, interfaces can be used to achieve 100% abstraction. This is because abstract classes can have both abstract methods (methods without a body) and non abstract methods, whereas, interfaces can only have abstract methods.
21. What are abstract classes and abstract methods?
Abstract keyword is used to declare classes and methods as abstract. Variables can not be abstract.
-
Abstract class: In Java, a class that uses the abstract keyword in its declaration is considered abstract. It might use non-abstract as well as abstract methods. It is necessary to declare a class as abstract if it has at least one abstract method. An abstract class cannot have an instance created for it.
Syntax:abstract class ClassName{ //body }
-
Abstract method: Methods that are declared as abstract using the "abstract" keyword do not need to be implemented before being declared. Only the method signature is included in these methods; there is no method body. A class should be defined as an abstract class if it has an abstract method. However, the contrary may not be true, i.e., an abstract class need not have an abstract method. Abstract methods need to be implemented in the subclasses of the abstract class.
Syntax:abstract return_type method_name(parameters);
22. Compare an abstract class and an interface?
Both interfaces and abstract classes are used to achieve abstraction in Java. They need to be inherited in order to be implemented. They contain abstract methods that have no body and need to be overridden in the subclass to be executed.
Abstract Class | Interface |
---|---|
It can contain both abstract and non abstract methods. | It can only contain abstract methods and hence help us achieve 100% abstraction. |
It can have all static, non-static and final, non-final variables. | It can only contain final and static variables. |
It does not support multiple inheritance. | It supports multiple inheritance. |
It is declared using "abstract" keyword and is inherited using "extends" keyword. | It is declared using "interface" keyword and is inherited using "implements" keyword. |
Class members can be private, protected, etc. | Interface has public class members by default. |
23. What are access specifiers? What are the access specifiers used in Java?
The accessibility or scope of a field, function, constructor, or class is specified by the access modifiers in Java. By using the access modifier, we can alter the access level of fields, constructors, methods, and classes.
Java has 4 access specifiers - private, protected, public and default. The default modifier does not have any keyword associated with it.
Private | Protected | Default | Public | |
---|---|---|---|---|
Same class | Y | Y | Y | Y |
Same package subclass | N | Y | Y | Y |
Same package non subclass | N | Y | Y | Y |
Different package subclass | N | N | Y | Y |
Different package non subclass | N | N | N | N |
24. What are constructors?
In object-oriented programming, a constructor is a particular method of a class or structure that initialises a newly formed object of that class. The constructor is always invoked once an object is created.
A constructor has the same name as that of its class and has no return type. It cannot be static, final, abstract, or synchronised. It is usually used to initialise the data members of an object.
25. List the types of constructors.
There are primarily 3 types of constructors in Java:
- Parameterized Constructor: These are the type of constructors that can accept one or more arguments as input while initialzing an object.
- No-Arg Constructor: A constructor is referred to be a no-argument constructor if it does not accept any parameters.
- Default Constructor: In Java, a constructor with no parameters is referred to as a default constructor when no no-arg constructor is explicitly defined in the class. Java compiler automatically builds a constructor for a class when no constructor is declared for that class.
class Main{
Main(int i){
//this is a parameterized constructor
}
Main(){
//this is a no arg constructor
}
}
Constructors can be overloaded the same way methods are overloaded - based on the parameters. A default constructor is called in case there is no explicit no-arg constructor and no parameters are taken while creating an object.
26. Define copy constructors.
A copy constructor is a kind of constructor that copies one object to another. It means that, assuming both objects are of the same class, a copy constructor will copy an object and its values into a different object. It initializes an object using another object of the same class which has been previously created. It takes a reference to another object of the same class as argument.
Syntax:
Classname (const classname &obj) {
// body of constructor
}
27. What is exception? What is exception handling?
An exception is a disruptive occurrence that takes place at run time, or during the execution of a program, which interrupts the regular flow of the program's instructions. The program has the ability to detect and manage exceptions. An object is created when an exception occurs within a method called an exception object. It includes details about the exception, including its name, description, and the program's status at the time the exception occurred.
Efficient ways to deal with runtime failures in Java is through exception handling, which helps to maintain the application's normal flow. Runtime issues such as ClassNotFoundException, IOException, SQLException, RemoteException, etc. can be handled through the Java Exception Handling mechanism.
28. When is finally blocked executed in java?
Whether an exception has occurred or not, a finally block is always executed. It is carried out after the try or catch block has finished running.
Unless there is an anomalous program termination caused by a JVM crash or a request to System.exit(), the finally block is always executed. In situations like w hen the JVM runs out of memory or when the Java process is forcibly killed from the task manager or terminal or when the computer shuts down due to a power outage, or a deadlock, a finally block won't run.
29. What is a destructor?
In C++, destructors are class member functions that destroy an object. They are triggered whenever a class object exits its scope, including at the conclusion of a function, the conclusion of a program, the calling of a delete variable, etc.
Since destructors don't accept arguments and return nothing. Destructors are also named after their classes and are prefixed by a tilde (~).
#include<iostream>
using namespace std;
class Demo {
public:
int n;
Demo(int i) {
cout<<"Inside Constructor"<<endl;
n = i;
}
void display() {
cout<<"n = "<< n <<endl;
}
~Demo() {
cout<<"Inside Destructor";
}
};
int main() {
Demo obj1(10);
obj1.display();
return 0;
}
Output:
Inside Constructor
n = 10
Inside Destructor
30. What is meant by Garbage Collection?
Programming languages like C# and Java include garbage collection (GC) as a memory recovery mechanism. A programming language that supports garbage collection (GC) contains one or more GC engines that automatically release memory space that has been reserved for objects the application is no longer using. The freed up memory can then be used to further object allocations in that program.
A programmer is in charge of both creating and destroying objects in C/C++. However in Java, the programmer need not deal with objects that are no longer in use. These items are destroyed by the garbage collector. Garbage Collector's primary goal is to release heap memory by eliminating inaccessible objects.
Practical Questions
1. Which feature of OOP is indicated by the following code?
class student
{
int marks;
};
class topper : public student
{
int age;
topper(int a) { age = a; }
};
Encapsulation is indicated by use of classes. It is the mechanism that binds together code and the data it manipulates. Inheritance is shown by inheriting the student class into topper class. It is the mechanism by which one class is allowed to inherit the features(fields and methods) of another class.
2. What will be the output of the following code?
class A
{
int i;
A(){
i=0;
}
};
class HelloWorld {
public static void main(String[] args) {
A obj;
System.out.println(obj.i);
}
}
Output: error: variable obj might not have been initialized
The object of class A - obj - has just been declared and not initialised. Objects are initialised using the new keyword.
3. What is the output of the following code:
class Print {
public static void function(String s) {
try {
System.out.println(s);
return;
} catch (Exception e) {
System.out.println("Error");
} finally {
System.out.println("Finally");
}
}
public static void main(String[] args) {
function("Hello");
}
}
Output:
Hello
Finally
The finally block is always executed in Java regardless of the occurrence of an exception. It will also be executed after the return statement. If System.exit()
is called before a finally block, it will not execute.
Consider the following code and predict the output of the following questions
class A{
A(){
System.out.println("Constructor A");
}
}
class B extends A{
B(){
System.out.println("Constructor B");
}
}
4.B obj = new B();
Output: Constructor A
Constructor B
5. A obj1 = new B();
Output: Constructor A
Constructor B
6. A obj2 = new A();
Output: Constructor A
7. B obj3 = new A();
Output: error: incompatible types: A cannot be converted to B
8. What OOP concepts are reflected in the following code
class A{
public void print(){
System.out.println("Class A");
}
}
class B extends A{
public void print(){
System.out.println("Class B");
}
}
class C extends B{
public void print(){
System.out.println("Class C");
}
}
class Test{
public static void main(String[]args){
C obj = new C();
obj.print();
A obj1 = new C();
obj1.print();
}
}
In the given code snippet, we can see Inheritance and Polymorphism. Class B inherits from class A and class C inherits from class B. This is multilevel polymorphism. The print function is being overridden in the child classes, demonstrating polymorphism.
9. What will be the output of the code in the above question?
Output: Class C
Class C
10. What will be the output of the following code
public class Demo{
public static void main(String[] arr){
System.out.println(“Main1”);
}
public static void main(String arr){
System.out.println(“Main2”);
}
}
Output: Main1
11. What OOP concepts are shown in the following code?
abstract class P{
public abstract void m1();
}
class C extends P{
public void m1(){
System.out.println("abstract method");
}
public void m2(){
System.out.println("Child");
}
}
The code demonstrates Abstraction, Inheritance and Polymorphism. Abstraction is achieved by using abstract class and abstract method. Class C inherits from abstract class. The abstract method m1 has to be overridden in the subclass.
Consider the following code and predict the output of the following questions
class P{
public void m1(){
System.out.println("parent");
}
}
class C extends P{
public void m2(){
System.out.println("Child");
}
}
P p = new P();
p.m1();
p.m2();
Output:
error: cannot find symbol
obj.m2();
^
symbol: method m2()
location: variable obj of type P
1 error
13.
C c=new C();
c.m1();
c.m2();
Output:
parent
Child
14.
P p = new C();
p.m1();
p.m2();
Output:
error: cannot find symbol
p.m2();
^
symbol: method m2()
location: variable p of type P
1 error
15.
C c = new P();
c.m1();
c.m2();
Output:
error: incompatible types: P cannot be converted to C
C c = new P();
^
1 error