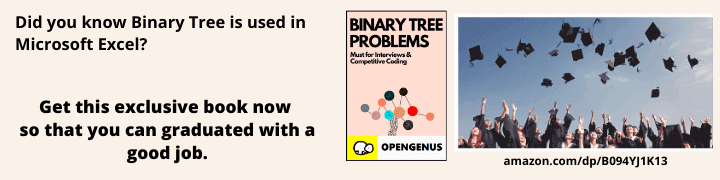
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Spring is a Java based framework. It was developed by Rod Johnson. The first version of spring framework was released by Rod Johnson along with his book Expert One-on-one J2EE Design and Development in October 2002. The framework was first released under the Apache 2.0 license in June 2003. In this article, we have covered 70 Spring Interview Questions and Answers.
1. What is Spring?
Spring is a Java based framework that is used to develop modern java-based enterprise applications. Spring framework can used to develop any kind of java application including standalone and web applications. It provides support for developing microservices with spring boot. Spring framework provides modules that makes the development of data driven and web-based applications easier. It follows the POJO-based programming model.
2. What are the features of Spring Framework?
Spring framework is long lived and famous in market because of the features and ease of development it provides to the java developers. Following are some of the features of spring framework:
- Lightweight: Spring is a light weight framework in terms of size and transparency. The size of the basic version of spring framework is just about 2MB.
- Inversion of Control: Spring framework provides IOC with the help of dependency injection. Inversion of Control helps in building a scalable in loosely coupled software application.
- Aspect-Oriented Programming: Spring supports aspect-oriented programming that helps in separating the application's business logic and cross-cutting concerns.
- Transaction Management: Transaction management is an important part of RDBMS-oriented enterprise application to ensure data integrity and consistency. Spring has a support for robust transaction management. Spring provides transaction management interfaces that can scale down to local transaction and scale up to global transaction. Spring provides two types of transaction management- programmatic and declarative.
- Exception Handling: Spring provides a convenient API to translate technology-specific exceptions (thrown by JDBC, Hibernate, or JDO) into consistent, unchecked exceptions.
3. What is Spring Bean?
In Spring Framework, a bean is an object that is instantiated, assembled, and otherwise managed by a Spring IoC container. The objects that form the backbone of your application and that are managed by the Spring IoC container are called beans. Spring ApplicationContext
is used to get the Spring Bean instance and spring IoC container manages the life cycle of Spring Bean.
4. Explain Spring Bean lifecycle.
As lifecycle of anything spring bean lifecycle includes instantiation, use and destruction of it. Before the bean can used we must ensure that it has been instantiated and once there's no longer need of the been in the system, it should be removed from the system to free-up the memory.
We can understand the lifecycle of beans with following steps:
- The bean lifecycle starts with the Spring IoC container instantiating the bean from the bean’s definition present in the XML file
- Spring populates all of the properties as specified in the bean definition (DI).
- If the bean implements
BeanNameAware
interface, spring passes the bean’s id tosetBeanName()
method. - If Bean implements
BeanFactoryAware
interface, spring passes thebeanfactory
tosetBeanFactory()
method. - If there are any bean
BeanPostProcessors
associated with the bean, Spring callspostProcesserBeforeInitialization()
method. - If the bean implements
IntializingBean
, itsafterPropertySet()
method is called. If the bean has init method declaration, the specified initialization method is called. - If there are any BeanPostProcessors associated with the bean, their postProcessAfterInitialization() methods will be called.
- If the bean implements
DisposableBean
, it will call thedestroy()
method.
5. Are Singleton beans thread-safe in Spring Framework?
No, singleton beans are not thread-safe in Spring Framework.
6. What are the different options in Spring to inject Java Collection?
Spring Framework provides following types of java collection configurations elements:
- The
<list>
type is used for the purpose of injecting a list of values with duplicates allowed. - The
<set>
type is used for the purpose of injecting a collection of values but with not duplicates in the collection is allowed. - The
<map>
type is used for the purpose of injecting a collection of key-value pairs where key and value can be any type. - The
<props>
type is used for the purpose of injecting a collection of key-value pairs where key and value both are of type String.
7. Can you inject null and empty string values in Spring?
Yes, you can.
8. What is IOC and Dependency Injection in Spring?
IoC stands for Inversion of Control. It simply means delegating the responsibility of creating an object to a container or framework. Now the programmer do not have to create an object of class using new
keyword. There are many ways to achieve IoC. Some of these are, Strategy Design pattern, Service Locator pattern, Factory pattern and Dependency Injection.
Simply put, Dependency Injection is a pattern we can use to achieve the Inversion of Control.
9. What are the different bean scopes in Spring?
Following are the types of scope defined by the latest version of Spring framework:
- Singleton
- Prototype
- Request
- Session
- Application
- Websocket
Last four scopes mentioned are available only in web-aware applications.
10. What are the different ways to configure Spring Applications?
Following are the three ways to define the configuration of a Spring Application
- XML based configuration
- Java based configuration
- Annotation based configuration
11. Explain various ways of Dependency Injection in Spring.
There are three ways of dependency injection in Spring:
- Constructor based Dependency Injection
- Setter based Dependency Injection
- Field or Property-based Dependency Injection
12. What is the difference between Constructor Injection and Setter Injection?
Constructor Injection
- It uses constructor to inject the dependency
- Fields are accessed through index
- It initializes a class properly
- It has the benefit of testability
Setter Injection
- It uses setter methods to inject the dependency
- It is more readable in XML configuration file
- While using setter injection complete dependency injection is not guaranteed
- It is less secure compared to constructor injection
13. Explain the use cases of Singleton and Prototype Scopes?
Singleton is used when only one instance of the bean definitions get instantiated in the spring container. A new request can only be made if the container has no other pending requests.
Prototype scope allows one to make new instances for each request that gets passed. There is no need to wait for clearing of the container. Depending on what your requirement it is, you would pick one over the other. The injections can be mentioned in the bean configuration file.
14. How many types of IOC containers are available in Spring?
There are two types of IoC contains in Spring
BeanFactory
ApplicationContext
15. Describe important Spring modules.
Some of the important Spring modules are following:
- Spring AOP
- Spring MVC
- Spring Data JPA
- Spring Data JDBC
16 Explain @Autowired
annotation.
@Autowired
annotation is used to define the automatic dependency injection in spring. Spring container has the capability to autowire the relationships between the collaborating beans. This is called spring bean autowiring.
17. What are the different types of spring bean auto-wiring?
Following are the different modes of auto-wiring in spring:
No
- No autowiring at all. This is the default mode.byName
- This option enables the dependency injection based on bean names.byType
- This option enables the dependency injection based on bean types.constructor
- Autowiring byconstructor
is similar tobyType
, but applies to constructor arguments.
18. What are the different types of transaction management?
There are support for two types of transaction management in Spring Framework:
- Programmatic Transaction Management
- Declarative Transaction Management
19. What are the benefits of using IoC?
The primary benefits of IoC include –
- Minimizes the number of codes in any application
- Makes a platform/application easy to test, as it doesn’t need singletons or JNDI lookup mechanisms
- Promotes loose coupling with fewer efforts and intrusive mechanism
- Facilitates Eager Instantiation
20. What are the advantages of Reactive Programming?
The following are some of the advantages of Reactive Programming:
- Helps to increase the utilization of computing resources, such as multicore and multi-CPU hardware.
- Complex threading becomes very easy
- Helps to increase the performance by a reduction in the serialization process.
- Easy to implement back-pressure
21.What are the various ways of using Spring Framework?
You can use Spring Framework:
- for writing web applications
- for exposing RESTful services
- to secure your web applications
- for communicating with databases
- for handling long running jobs
- to handle external resources or systems you have to work with
- for testing purposes
- for standalone java projects
- to convert your application into an executable
- to integrate Social Media into your applications
22. What is the advantage of NamedParameterJDBC
Template?
Instead of traditional placeholders arguments, the ‘NamedParameterJDBCTemplate
’ enables you to use parameters instead. It is easier to maintain and helps with readability.
23. Differentiate Spring vs Spring MVC vs Spring Boot?
- Spring: the most important feature of Spring is Dependency Injection or Inversion of Control.
- Spring MVC: is a complete HTTP oriented MVC framework managed by the Spring Framework and based in Servlets. It would be equivalent to JSF in the JavaEE stack.
- Spring Boot: is a utility for setting up applications quickly, offering an out of the box configuration in order to build Spring powered applications.
24. Why is the Spring bean configuration file necessary?
Ans. Spring Bean configuration file can be used to define all the beans initialized by Spring Context. Spring Bean configuration file reads and initializes the Spring bean XML file when the instance of Spring ApplicationContext
is created. These files can then be used to get different bean instances once the context is initialized.
25. Name Some of the Design Patterns Used in the Spring Framework?
- Singleton Pattern – singleton-scoped beans
- Factory Pattern – Bean Factory classes
- Prototype Pattern – prototype-scoped beans
- Adapter Pattern – Spring Web and Spring MVC
- Proxy Pattern – Spring Aspect-Oriented Programming support
- Template Method Pattern – JdbcTemplate, HibernateTemplate, etc.
- Front Controller – Spring MVC DispatcherServlet
- Data Access Object – Spring DAO support
- Model View Controller – Spring MVC
26. Explain the working of Scope Prototype?
Scope prototype means that every time we call for an instance of the Bean, Spring will create a new instance and return it. This differs from the default singleton scope, where a single object instance is instantiated once per Spring IoC container.
27. What are the different ways of controlling the life cycle events of a bean?
There are 2 ways for controlling the life cycle events of a bean –
- InitializingBean and DisposableBean interfaces
- Init-method and destroy-method
28. What is the difference between Tight Coupling and Loose Coupling?
Tight Coupling: Tight coupling is when a group of classes are highly dependent on one another.
Loose Coupling: Loose coupling is achieved by means of a design that promotes single-responsibility and separation of concerns.
29. What Is Spring DAO?
Spring Data Access Object (DAO) is Spring's support provided to work with data access technologies like JDBC, Hibernate and JPA in a consistent and easy way.
30. What does a Spring Bean definition contain?
A Spring Bean definition contains all configuration metadata which is needed for the container to know how to create a bean, its lifecycle details and its dependencies.
31. What are Singleton Scopes used with?
Singleton Scopes are used with Stateless session bean.
32. Name some AOP concepts.
Different types of AOP concepts are –
- Interceptor
- Joinpoint
- Aspect
- Target object
- Weaving
- Pointcut
- Advice
- AOP proxy
33. What are the benefits of the Spring Framework’s transaction management?
- It provides a consistent programming model across different transaction APIs such as JTA, JDBC, Hibernate, JPA, and JDO.
- It provides a simpler API for programmatic transaction management than a number of complex transaction APIs such as JTA.
- It supports declarative transaction management.
- It integrates very well with Spring’s various data access abstractions.
34. Explain AOP.
Aspect-oriented programming, or AOP, is a programming technique that allows programmers to modularize crosscutting concerns, or behavior that cuts across the typical divisions of responsibility, such as logging and transaction management.
35. What are the advantages of spring Aspect Oriented Programming?
Following are the advantages of spring AOP:
- It is not invasive
- Can be used to implement java (which is pure)
- Use of container system for dependency injections
- Implements cross cutting concern with no calls made to it directly
- Helps in regulation of cross cutters
- Can use XML configuration and @AspectJ annotation
36. What are the different types of Advice?
Following are the types of advice in Spring AOP:
- Before
- After
- After-running
- After-throwing
- Around
37. What are the different types of events in Spring framework?
Here is a list of events in spring framework
- A custom event
- Application
- Publisher
- Listener
- Asynchronous event
- Existing framework event
- Annotation driven event
- Generic support
- Generic application event
- Listener
- Publisher
- Transaction bound event
38. What are the limitations with auto wiring?
Here is the list of disadvantages and limitations of auto wiring in spring while running web applications.
- Autowiring is not completely precise. It can cause ambiguity which is not handled by spring with ease.
- The documentations of spring may not have access to autowired information
- Multiple beans with respect to their containers may get attached to the wrong constructor.
- Primitive classes and strings can not be autowired
- There are high chances of overriding with auto wiring in spring
- The definition of primitive data needs to be called out for auto wiring
- The high dependencies in the program can cause a lot of chaos and confusion
39. What is weaving?
Weaving is a process of applying aspects to the target object or other application types to create an advised object or a new proxy object.
40. On what points can you apply weaving?
We can apply weaving on the following points –
- Compile Time
- Class load Time
- Runtime
41. Explain JoinPoint.
JoinPoint showcases a point in an application where we can plug in an abstract-oriented programming aspect. This is the actual place where actions will be taken with the help of the Spring AOP framework.
42. Explain Aspect.
The core construct of AOP is the aspect, which encapsulates behaviors affecting multiple classes into reusable modules. It is a module which has a set of APIs providing cross-cutting requirements. For example, a logging module would be called AOP aspect for logging. An application can have any number of aspects depending on the requirement. In Spring AOP, aspects are implemented using regular classes annotated with the @Aspect
annotation (@AspectJ
style).
43. Explain Advice
The advice is the actual action that will be taken either before or after the method execution. This is actual piece of code that is invoked during the program execution by the Spring AOP framework.
44. Explain Pointcut
The pointcut is a set of one or more joinpoints where an advice should be executed. You can specify pointcuts using expressions or patterns.
45. What is Target object?
The target object is an object being advised by one or more aspects. It will always be a proxy object. It is also referred to as the advised object.
46. What is a proxy in Spring Framework?
proxy in the Spring framework is referred to as the creation of an object after applying advice to a particular target object.
47. What Is Spring WebFlux?
Spring WebFlux is Spring's reactive-stack web framework, and it's an alternative to Spring MVC.
In order to achieve this reactive model and be highly scalable, the entire stack is non-blocking.
48. What Is the Use of WebClient and WebTestClient?
WebClient is a component in the new Web Reactive framework that can act as a reactive client for performing non-blocking HTTP requests. Since it's reactive client, it can handle reactive streams with back pressure, and it can take full advantage of Java 8 lambdas. It can also handle both sync and async scenarios.
On the other hand, the WebTestClient is a similar class that we can use in tests. Basically, it's a thin shell around the WebClient. It can connect to any server over an HTTP connection. It can also bind directly to WebFlux applications using mock request and response objects, without the need for an HTTP server.
49. What Are the Disadvantages of Using Reactive Streams?
There are some major disadvantages to using reactive streams:
- Troubleshooting a Reactive application is a bit difficult
- There is limited support for reactive data stores since traditional relational data stores have yet to embrace the reactive paradigm.
- There's an extra learning curve when implementing.
50. Can We Use Both Web MVC and WebFlux in the Same Application?
No, we cannot use both of these together. As of now, Spring Boot will only allow either Spring MVC or Spring WebFlux, as Spring Boot tries to auto-configure the context depending on the dependencies that exist in its classpath.
Also, Spring MVC cannot run on Netty. Moreover, MVC is a blocking paradigm and WebFlux is a non-blocking style. So, we shouldn't be mixing both together because they serve different purposes.
51. What is the role of @SpringBootApplication?
The @SpringBootApplication annotation was introduced in Spring Boot 1.2.0 and it enables the auto-configuration feature.
This annotation encapsulates the working of three different annotations:
- @Configuration: Allows the developers to explicitly register the beans
- @ComponentScan: Enables the component-scanning so that the controller class and other components will be automatically discovered and registered as beans in spring’s application context
- @EnableAutoConfiguration: Enables the auto-configuration feature of spring boot
52. Can we send an Object as the response of Controller handler method?
Yes we can send JSON or XML based response in restful web services, using the @ResponseBody annotation.
53. What does the @RequestParam annotation do?
The @RequestParam annotation in spring binds the parameter values of a query string to the method argument of a controller.
54. What’s the difference between @Component, @Controller, @Repository & @Service annotations in Spring?
-
@Component: This marks a java class as a bean. It is a generic stereotype for any Spring-managed component. The component-scanning mechanism of spring now can pick it up and pull it into the application context.
-
@Controller: This marks a class as a Spring Web MVC controller. Beans marked with it are automatically imported into the Dependency Injection container.
-
@Service: This annotation is a specialization of the component annotation. It doesn’t provide any additional behavior over the @Component annotation. You can use @Service over @Component in service-layer classes as it specifies intent in a better way.
-
@Repository: This annotation is a specialization of the @Component annotation with similar use and functionality. It provides additional benefits specifically for DAOs. It imports the DAOs into the DI container and makes the unchecked exceptions eligible for translation into Spring DataAccessException.
55. What is the role of @SpringBootApplication?
The @SpringBootApplication annotation was introduced in Spring Boot 1.2.0 and it enables the auto-configuration feature.
This annotation encapsulates the working of three different annotations:
- @Configuration: Allows the developers to explicitly register the beans
- @ComponentScan: Enables the component-scanning so that the controller class and other components will be automatically discovered and registered as beans in spring’s application context
- @EnableAutoConfiguration: Enables the auto-configuration feature of spring boot
56. Explain WebApplicationContext
.
The WebApplicationContext
is an extension of the plain ApplicationContext
. It has some extra features that are necessary for web applications. It differs from a normal ApplicationContext
in terms of its capability of resolving themes and in deciding which servlet it is associated with.
57. How do you turn on annotation wiring?
Annotation wiring is not turned on in the Spring container by default. In order to use annotation based wiring we must enable it in our Spring configuration file by configuring <context:annotation-config/>
element.
58. Explain @RequestMapping
annotation
The @RequestMapping
annotation is used to map the web request onto a handler class (i.e. Controller) or a handler method and it can be used at the Method Level or the Class Level. If developers use the @RequestMapping annotation at a class level, it will be as a relative path for the method level path.
59. What do you understand by the Spring MVC framework?
The Spring MVC framework is responsible for providing model-view-controller architecture as well as ready-to-use components, used for developing flexible and loosely coupled web apps.
The MVC pattern helps in separating out the various aspects of the application, such as business logic, input logic, and UI logic, in addition to providing a loose coupling amongst these separated elements.
61. What do you mean by Spring DAO support?
The Spring DAO support eases working with data access technologies, such as JDBC, JDO, and Hibernate, in a reliable way. Also, it allows coding without worrying about catching specific-technology exceptions and easily makes a switch amongst persistence technologies.
62. How will you access Hibernate using Spring Framework?
Hibernate can be accessed using Spring Framework in the following two ways:
- Extending HibernateDAOSupport and then applying an AOP Interceptor node
- Inversion of Control with a Hibernate Template and Callback
63. Explain DispatcherServlet
.
The DispatcherServlet
is the essence of Spring Web MVC framework and handles all the HTTP requests as well as responses. Upon receiving the entry of handler mapping from the configuration file, the DispatcherServlet
forwards the request to the controller.
Thereafter, the controller returns an object of Model and View. Afterward, the Dispatcher Servlet checks the configuration file for the entry of view resolver and calls the specified view component.
64. How can you fetch records by spring JdbcTemplate
?
There are two interfaces that can be used to fetch records from the database:
- ResultSetExtractor
- RowMapper
65. What is the difference between JDBC and Spring JDBC?
Spring JDBC value-add provided by the Spring Framework’s on top JDBC layer
- Define connection parameters
- Define connection parameters
- Open the connection
- Specify the statement
- Prepare and execute the statement
- Set up the loop to iterate through the results (if any)
- Do the work for each iteration
- Process any exception
- Handle transactions
- Close the connection
66. ORM’s Spring support
Spring supports the following ORM’s:
- Hibernate
- JPA (Java Persistence API)
- TopLink
- JDO (Java Data Objects)
- OJB
67. What are some of the important Spring annotations?
List of some of the Spring annotations are:
- @Service is used to indicate a class as a Service.
- @Scope is used to configure the scope of the spring bean.
- @Configuration is used to indicate that the class can be used as a source of bean definitions by the Spring IoC container.
- @ComponentScan is used to scan all the classes available under a package.
- @Required used to indicate the affected bean property that must be populated at configuration time, by an explicit property value in a bean definition or with the help of autowiring.
- @ResponseBody is used to send an object as a response, basically used for sending XML or JSON data as a response.
- @PathVariable is used to map dynamic values from the URI to handler method arguments.
- @Autowired is used for autowire bean on the setter method similar to @Required annotation, on the constructor, on a property, or on methods with arbitrary names and/or multiple arguments.
- @Component is used to indicate a class as a component, where all the classes are used for auto-detection and composed as a bean when annotation-based configurations are used.
- @Controller is used for MVC applications and is generally used with @RequestMapping annotation.
- @Repository annotation indicates that a component is used as a mechanism to store/retrieve/search data.
- @Qualifier is used for wiring a single bean with a property at a time when there are multiple beans of similar type with the help of @Autowired annotation
- @Bean is used in java based configurations, where a method annotated with @Bean will return an object that should be registered as a bean in the Spring application context.
68. What are the different ways to create a Spring Boot application using Maven?
The following are some of the ways to create a Spring Boot application using Maven:
- Spring Boot CLI
- Spring Initializr
- Spring Starter Project Wizard
- Spring Maven Project
69. What is @bean spring?
Beans are the objects that are the backbone of the application. These are initiated, assembled as well as managed by Spring IoC container.
70. How to use jetty instead of tomcat in spring-boot-starter-web?
We can use jetty instead of tomcat in spring-boot-starter-web by replacing the existing dependency.
Step 1: Find the following dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Step 2: Replace it with:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
Step 3: Add Jetty:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
With this article at OpenGenus, you must have a good practice of String for Interviews.