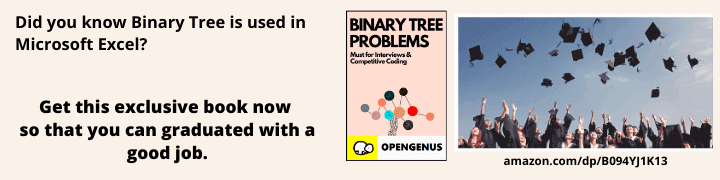
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this OpenGenus article, we will write a program in C to verify the legitimacy of a credit card and determine the corresponding company using the Luhn algorithm. We will provide you with a generic template that can be customized to suit your needs.
Table of Content:
- Checking credit card legitimacy using C / raw code
- Checking Card Type
- Checking Card Legitimacy with Luhn Algorithm
- Sample Input/Output Code
- Understanding credit card legitimacy and types
- The Luhn algorithm
- Limitations of the code and conclusion.
Let's write a program in C to check legitimacy and company of a credit card.
Checking credit card legitimacy using C
#include <stdio.h>
int main() {
char cardNumber[20];
int sum = 0, digit, i;
printf("Enter your credit card number: ");
scanf("%s", cardNumber);
// Check for card type
if (cardNumber[0] == '4') {
printf("Visa\n");
} else if (cardNumber[0] == '5') {
printf("Mastercard\n");
} else if (cardNumber[0] == '3' && cardNumber[1] == '4' || cardNumber[1] == '7') {
printf("American Express\n");
} else {
printf("Unknown card type\n");
}
// Check for card legitimacy using Luhn algorithm
for (i = 0; cardNumber[i] != '\0'; i++) {
digit = cardNumber[i] - '0';
if (i % 2 == 0) {
digit *= 2;
if (digit > 9) {
digit = digit % 10 + digit / 10;
}
}
sum += digit;
}
if (sum % 10 == 0) {
printf("Valid credit card number\n");
} else {
printf("Invalid credit card number\n");
}
return 0;
}
Sample input, ouput code.
Understanding credit card legitimacy and types
In this code, we start by declaring a character array cardNumber to store the user's credit card number. We then prompt the user to enter their credit card number using scanf.
Next, we check the first digit of the credit card number to determine its type. Visa credit cards start with the digit 4, Mastercard credit cards start with the digit 5, and American Express credit cards start with the digits 34 or 37. If the first digit doesn't match any of these, we print "Unknown card type".
The Luhn algorithm
After checking the card type, we use the Luhn algorithm to check the legitimacy of the credit card number. The Luhn algorithm works by doubling every other digit in the credit card number, starting with the second-to-last digit, and adding up all of the digits. If the sum is evenly divisible by 10, the credit card number is considered valid.
In the code, we loop through the credit card number string, convert each character to a digit, and apply the Luhn algorithm to calculate the sum. We then check whether the sum is evenly divisible by 10 and print "Valid credit card number" or "Invalid credit card number" accordingly.
Limitations of the code
This is not all the checkpoints we can put to check the credit card and it does not check all the companies. It depends on what purpose you are trying to build the credit card checker. If you want to check all the possible companies then you have to check their starting number and different combinations if they have same starting numbers. you can use a .json file which contains all the companies data of credit to check their credentials and check against this programm. All credit card has to follow a pattern to start with and one programme will be enough to check their legitimacy but to check their companies you have to check their company preference how their card starts or ends as a pattern.
Try to improve this code to check against a lot more companies, or try other algorithms, Trial and error is the best way to learn how to code.