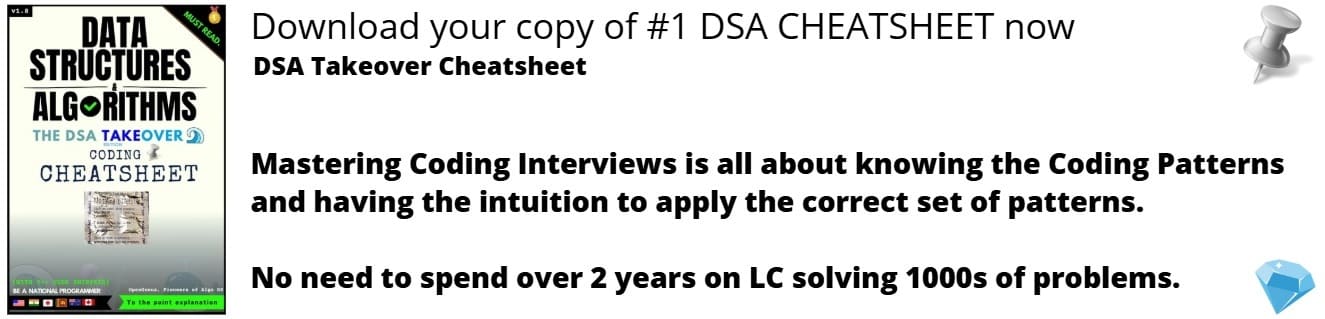
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explored the concept of MOVZX assembly instruction in depth with code examples.
Table of contents:
- MOVZX
- C code converted to MOVZX in Assembly
- C code to run MOVZX
- Intrinsic for MOVZX
MOVZX
MOVZX stands for "Move with Zero Extend". MOVZX is an assembly instruction. It is available since the 80386 processor and is supported with all SIMD instruction sets like SSE2, AVX2, AVX512 and others.
MOVZX is used to copy the content of a byte or a source operand to a larger sized destination operand and fills the extra bits of the destination operand with zeroes.
It is used as follows:
MOVZX EAX, AL
- AL is a 8 bit register
- EAX is a 32 bit register
- Value of AL to copied to EAX; the rest of the 24 bits in EAX is filled with 0.
So, if AL=01101011 (8 bits)
then after the operation, EAX = 00000000000000000000000001101011 (note the last 8 bits are same as AL and all other bits are 0).
MOVZX is mainly used for the following cases:
- Loading data from memory to a register as register size is larger than the data in most cases
- Moving data between two variables or array of different datatype/ size.
C code converted to MOVZX in Assembly
Consider the following C code:
#include <stdio.h>
int main() {
unsigned char c = 0xFF;
unsigned int i = c;
printf("%u\n", i);
return 0;
}
Consider these 2 lines:
unsigned char c = 0xFF;
unsigned int i = c;
Note:
- Size of c (char datatype) is 8 bits
- Size of i (int datatype) is 32 bits
- So, we are moving a smaller data to a larger data
- Hence, MOVZX assembly instruction will be used in this case.
Following is the corresponding Assembly code that is usign MOVZX:
section .data
message db "%u", 10, 0
section .text
global main
main:
; Initialize variables
xor eax, eax
mov al, 0xFF ; unsigned char c = 0xFF;
movzx eax, al ; unsigned int i = c;
; Print unsigned int i
push eax
push message
call printf
add esp, 8
; Return 0
xor eax, eax
ret
C code to run MOVZX
In C Programming Language, we can run an assembly instruction like MOVZX as follows:
unsigned int i;
__asm__ ("movzx %%al, %0;" : "=r" (i) : "a" (c));
Intrinsic for MOVZX
The intrinsic for MOVZX is _mm_cvtepu8_epi32
. Following is the complete C code to demonstrate this:
#include <immintrin.h>
int main() {
unsigned char c = 0xFF;
__m128i i = _mm_cvtepu8_epi32(_mm_set1_epi8(c));
// do something with i
return 0;
}
With this article at OpenGenus, you must have the complete idea of MOVZX.