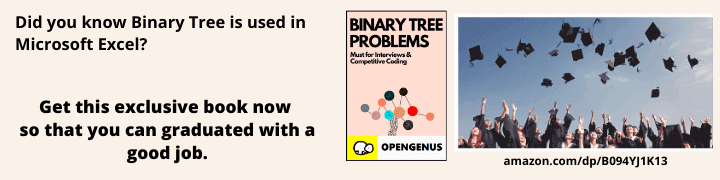
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explained the concept of LEA assembly instruction which is used in x86 processors.
Table of contents:
- X86 LEA - Load Effective Address
- How LEA is used?
- Intrinsic for LEA + C code using LEA
- LEA vs ADD
X86 LEA - Load Effective Address
LEA stands for "Load Effective Address". LEA is an assembly instruction that is used to store the effective memory address from an operand to a register.
The syntax of using LEA is as follows:
LEA destination, source
destination register will store the effective memory address of the source operand.
LEA instruction was supported since 8086 microprocessor which was released in 1978 by Intel. It is supported in all x86 and x86-64 processors. LEA instructions require 1 clock cycle in execution.
How LEA is used?
LEA can be used in the following ways:
- Calculating the offset of an array element
- Performing pointer arithmetic
- Calculating the address of a function
- Performing arithmetic operations on register values without changing the flags
For example, consider the following:
LEA eax, [array + 2*4]
In this, array is the base address of an array and 2 * 4 is the offset of an element at index 2 with each element being 4 bits in size. The effective address is stored in EAX register.
Similarly, LEA can be used in combination with other instructions to perform complex calculations and memory operations. LEA is frequently used to optimize code and reduce memory accesses.
Intrinsic for LEA + C code using LEA
Intrinsic for LEA (Load Effective Address) in x86 assembly language is _mm256_set_epi32()
which is available in the AVX2 instruction set.
Following is a C program which is directly using LEA instruction (using __asm__
) to perform a memory calculation:
#include <stdio.h>
#include <stdint.h>
#include <immintrin.h>
int main() {
uint32_t base = 0x1000;
uint32_t index = 0x200;
uint32_t scale = 4;
uint32_t displacement = 0x30;
uint32_t result;
__asm__ (
"lea %[result], [%[base] + %[index]*%[scale] + %[displacement]]"
: [result] "=r" (result)
: [base] "r" (base), [index] "r" (index), [scale] "r" (scale), [displacement] "r" (displacement)
);
printf("LEA result: 0x%X\n", result);
return 0;
}
LEA vs ADD
Both LEA and ADD instructions are used to perform arithmetic operations. The main differences are:
- LEA does not modify the flags while ADD do modify the flags.
- LEA is faster than ADD as LEA does not modify status flags.
- LEA is mainly used to perform address calculations while ADD is mainly used for arithmetic operations.
- LEA involve 1 clock cycle while ADD involve 1 to 4 clock cycles depending on the operands involved.
With this article at OpenGenus, you must have the complete idea of how to use LEA x86 instruction.