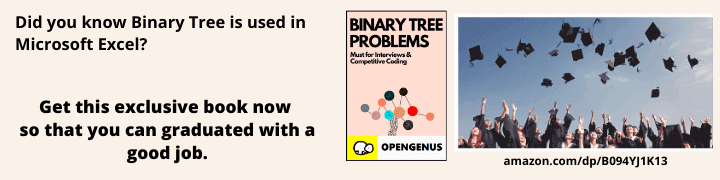
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explained how to implement Matrix Multiplication in Assembly. We have provided the complete assembly code to multiply 2 3x3 matrices as well.
Table of contents:
- Explanation: Matrix Multiplication in Assembly
- Code for Matrix Multiplication in Assembly
Explanation: Matrix Multiplication in Assembly
The main components of Matrix Multiplication in Assembly are:
- m, n and p are constants set to 3 representing the size of the input matrices (3x3 and 3x3)
- The multiplication is performed by MUL op which multiplies AL register with an operand and stores the result in AX register.
- The ADD op is used to add the results of the dot product of a row and column and save it in the output matrix.
- INT op is used to write the output matrix to the standard output and exit the program.
Code: Matrix Multiplication in Assembly
Following is the complete assembly code to perform Matrix Multiplication (with descriptive comment for each line of code):
; Declare variables and constants
section .data
; Input matrices
matrix1: times 3 db 1, 2, 3, 4, 5, 6, 7, 8, 9
matrix2: times 3 db 1, 0, 0, 0, 1, 0, 0, 0, 1
; Output matrix
result: times 9 db 0
; Constants
m equ 3 ; Number of rows in matrices
n equ 3 ; Number of columns in matrices
p equ 3 ; Number of columns in matrix2
section .text
global _start
_start:
; Load address of matrices into registers
mov esi, matrix1 ; Load address of matrix1 into esi
mov edi, matrix2 ; Load address of matrix2 into edi
mov ebx, result ; Load address of result matrix into ebx
; Initialize loop counters
mov ecx, m ; Outer loop counter
mov edx, n ; Middle loop counter
xor ebp, ebp ; Inner loop counter
; Perform matrix multiplication
outer_loop:
; Initialize sum to zero
xor eax, eax
middle_loop:
; Load elements of matrices
mov al, [esi + ebp] ; Load element from matrix1 into al
mov dl, [edi + ebp * p] ; Load element from matrix2 into dl
; Multiply and accumulate sum
mul dl ; Multiply al and dl
add [ebx + ebp], al ; Add result to corresponding element in result matrix
; Increment inner loop counter
inc ebp
; Check if inner loop counter has reached its limit
cmp ebp, edx
jl middle_loop ; Jump to middle_loop if ebp < edx
; Increment outer loop counters
add esi, n ; Move to next row in matrix1
add ebx, p ; Move to next row in result matrix
; Check if outer loop counter has reached its limit
dec ecx
jg outer_loop ; Jump to outer_loop if ecx > 0
; Print result matrix
mov eax, 4 ; System call for write
mov ebx, 1 ; File descriptor for standard output
mov ecx, result ; Address of result matrix
mov edx, m * p ; Number of bytes to write
int 0x80 ; Call kernel to write to standard output
; Exit program
mov eax, 1 ; System call for exit
xor ebx, ebx ; Exit status
int 0x80 ; Call kernel to exit program
With this article at OpenGenus, you must have the complete idea of how to do Matrix Multiplication in Assembly.