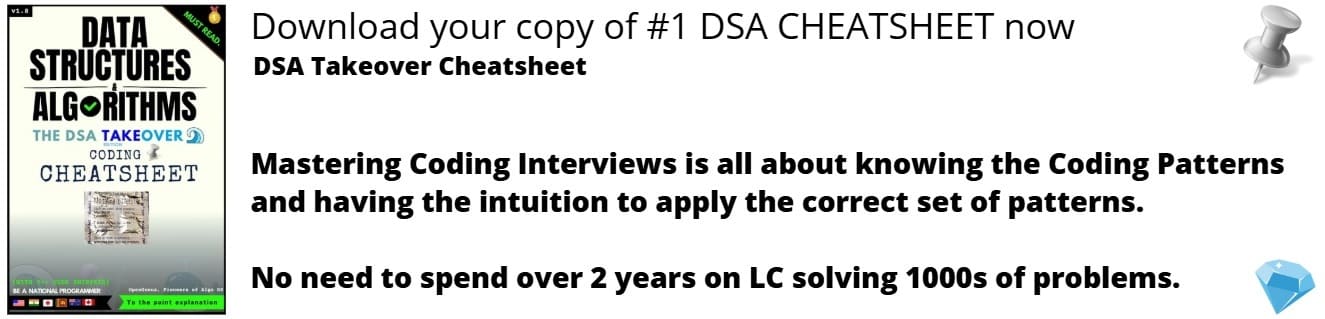
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explained how to implement an Array in x86 Assembly Programming Language. This gives a strong insight on how array function behind the high level programming languages.
Table of contents:
- How to implement Array in Assembly?
- Array in x86 Assembly Language
- Compile and run the code
How to implement Array in Assembly?
We define an array of 5 bytes using DB directive in .data section and initialized the first 5 elements as 10, 20, 30, 40 and 50.
myArray db 10, 20, 30, 40, 50 ;
We can access an element by loading it to a register. For example, in this assembly code snippet, we loaded the second element into a register using MOV:
mov bl, [myArray + 1] ;
We can also, modify an array element using MOV by moving the value of a register to a specific array element.
mov [myArray + 3], dl ;
We used a loop to iteration over the element of the array, loaded each element to a register by incrementing the loop counter. ECX is used as the loop counter which is initialized to 0 and the base address of the array is EBX.
mov ebx, 0
At the end, exit the program using (understand this part using this article):
; Exit the program
mov eax, 1 ; Set EAX to 1 (system call for exit)
xor ebx, ebx ; Set EBX to 0 (exit code)
int 0x80 ; Invoke the system call
Array in x86 Assembly Language
Following is the complete assembly code for implementing an array of 5 elements:
section .data
myArray db 10, 20, 30, 40, 50 ; Define an array of bytes with 5 elements
section .text
global _start
_start:
; Accessing array elements
mov al, [myArray] ; Load the first element of the array into the AL register
mov bl, [myArray + 1] ; Load the second element of the array into the BL register
mov cl, [myArray + 2] ; Load the third element of the array into the CL register
; Modifying array elements
mov [myArray + 3], dl ; Store the contents of DL into the fourth element of the array
; Accessing array elements using a loop
mov ecx, 0 ; Set ECX to 0
mov ebx, 0 ; Set EBX to the starting address of the array
loop_start:
cmp ecx, 5 ; Compare ECX with the length of the array
je loop_end ; If ECX equals 5, jump to loop_end
mov al, [ebx + ecx] ; Load the current element of the array into AL
inc ecx ; Increment ECX
jmp loop_start ; Jump to loop_start
loop_end:
; Rest of the program
; ...
; Exit the program
mov eax, 1 ; Set EAX to 1 (system call for exit)
xor ebx, ebx ; Set EBX to 0 (exit code)
int 0x80 ; Invoke the system call
Compile and run the code
Save the above code in a file named as array.asm.
To compile the above code in NASM, use the following command:
nasm -f elf64 -o array.o array.asm
ld -o array array.o
The executable is named as array. To run the executable, use the following command:
./array
With this article at OpenGenus, you must have the complete idea of how to implement an array in x86 Assembly language.