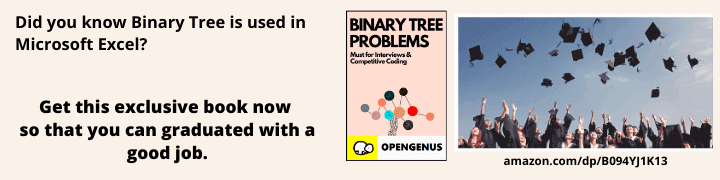
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Exploring the Architecture of the Core CPU Concept provides an in-depth look at the design and functionality of the core CPU concept. This article delves into the technical aspects of the architecture, including key terminology and diagrams, to help readers develop a thorough understanding of how the core CPU operates. Through detailed explanations of the concept's complexity, performance considerations, and real-life applications, readers will gain practical insights into CPU architecture. The article also offers interactive elements and community discussions to enhance the learning experience. Overall, this article is a comprehensive guide for those seeking to explore and understand the intricacies of the architecture of the core CPU concept.
Table of Contents
- Introduction
- Understanding CPU Architecture
- Visualizing CPU Architecture
- Practical Implementation
- Complexity
- Applications
- Questions
- Discussion
- Conclusion
Introduction
The central processing unit (CPU) is the brain of any computer system, responsible for executing the majority of its processing tasks. Within the CPU, the core is the component that performs the actual arithmetic and logical operations. Understanding the architecture of the core CPU concept is essential for anyone who wants to have a deep understanding of how computers work and how they process information. In this article, we will explore the intricacies of the architecture of the core CPU concept and highlight its importance in the field of computer science. We will examine its basic components, its complexity, and its performance considerations. Additionally, we will discuss some of the real-life applications of this concept, including the use of CPUs in gaming, AI, and scientific research. By the end of this article, readers will have a comprehensive understanding of the architecture of the core CPU concept and how it contributes to the overall functionality of a computer system.
Understanding CPU Architecture
CPU architecture refers to the design and implementation of the central processing unit (CPU) of a computer system. The CPU is responsible for executing instructions and performing calculations. Understanding the architecture of the CPU is crucial for anyone interested in computer science, as it provides insight into how a computer processes data.
To understand CPU architecture, it is essential to familiarize yourself with some key terminology. Some of the most important terms to know include:
Instruction Set: The set of instructions that the CPU can execute.
Registers: Small, high-speed memory locations within the CPU used to store data and instructions.
Cache: A small, high-speed memory used to temporarily store frequently accessed data and instructions.
Clock Speed: The speed at which the CPU can execute instructions, measured in hertz (Hz).
Pipeline: A technique used by CPUs to speed up instruction execution by breaking down instructions into smaller parts.
There are two main types of CPU architecture: RISC (Reduced Instruction Set Computing) and CISC (Complex Instruction Set Computing). RISC CPUs have a smaller instruction set and simpler instructions, allowing for faster execution. CISC CPUs have a larger instruction set with more complex instructions, which can lead to slower execution times but more powerful operations.
In the following sections, we will delve deeper into the intricacies of CPU architecture and explore the components that make up a CPU. By the end of this article, you will have a comprehensive understanding of CPU architecture and its importance in the field of computer science.
Visualizing CPU Architecture
Visual aids are an essential part of any educational material, and CPU architecture is no exception. Diagrams and images can help make abstract concepts more tangible and easier to understand. In this section, we will provide diagrams of CPU architecture to aid in your understanding.
One of the most crucial components of CPU architecture is the control unit. It directs the flow of data and instructions between the various components of the CPU, such as the arithmetic logic unit (ALU) and registers. A diagram of the control unit can be seen below:
Another critical component of CPU architecture is the pipeline. As mentioned earlier, the pipeline allows for faster execution of instructions by breaking them down into smaller parts. A visual representation of the pipeline can be seen below:
In addition to these diagrams, we will also provide images to help visualize other essential concepts in CPU architecture, such as cache memory and the instruction set.
An Instruction Set Architecture (ISA) is part of the abstract model of a computer that defines how the CPU is controlled by the software. The ISA acts as an interface between the hardware and the software, specifying both what the processor is capable of doing as well as how it gets done.
By incorporating these images into your understanding of CPU architecture, you will be able to better grasp the intricacies of how a computer processes data.
Practical Implementation
Understanding CPU architecture is essential for developing custom applications that can take full advantage of a computer's processing power. In this section, we will provide examples of code that implement key concepts in CPU architecture.
One such concept is parallelism, which allows for the execution of multiple instructions at once. One way to achieve parallelism is through the use of threads. The following code snippet shows how to create and manage threads in C++:
#include <iostream>
#include <thread>
using namespace std;
void worker_thread(int id) {
cout << "Worker thread " << id << " is running" << endl;
}
int main() {
const int num_threads = 4;
thread threads[num_threads];
// Launch a group of threads
for (int i = 0; i < num_threads; i++) {
threads[i] = thread(worker_thread, i);
}
cout << "Main thread is running" << endl;
// Join the threads with the main thread
for (int i = 0; i < num_threads; i++) {
threads[i].join();
}
cout << "All worker threads have completed" << endl;
return 0;
}
This code creates a group of worker threads and launches them to execute the worker_thread function. The main thread waits for all worker threads to complete using the join function. When all threads have completed, the main thread prints a message to indicate that the program has finished.
Another key concept in CPU architecture is pipelining. Pipelining involves breaking down instructions into smaller parts and executing them concurrently to improve performance. The following code snippet shows an example of pipelining in C++:
#include <iostream>
#include <vector>
using namespace std;
int main() {
// create an array of instructions
vector<string> instructions = {"add", "sub", "mul", "div", "mod"};
// initialize pipeline registers
string fetch = "";
string decode = "";
string execute = "";
// iterate over the instructions and simulate pipelining
for (int i = 0; i < instructions.size(); i++) {
// fetch instruction
fetch = instructions[i];
cout << "Fetch: " << fetch << endl;
// decode instruction
decode = fetch;
cout << "Decode: " << decode << endl;
// execute instruction
execute = decode;
cout << "Execute: " << execute << endl;
// remove instruction from pipeline
fetch = "";
decode = "";
execute = "";
}
return 0;
}
In this example, we simulate a pipeline with three stages:
fetch
,decode
, andexecute
. The instructions are represented as strings in an array, and we iterate over the array to simulate the pipelining process.
At each iteration, we fetch the instruction from the array and store it in the fetch register. Then, we decode the instruction by copying the fetch register to the decode register. Finally, we execute the instruction by copying the decode register to the execute register.
Once an instruction has been executed, we remove it from the pipeline by resetting all the registers to empty strings. This allows us to simulate the pipelining of multiple instructions, with each instruction going through the pipeline one stage at a time.
By incorporating these code examples into your understanding of CPU architecture, you will be better equipped to develop custom applications that can take full advantage of a computer's processing power.
Additionally, understanding CPU architecture is crucial for optimizing the performance of existing applications. By knowing how the CPU processes data and instructions, you can design algorithms and data structures that are more efficient and take advantage of the CPU's strengths.
Overall, the code examples provided in this section will help you better understand the practical implications of CPU architecture and how to apply it in real-world scenarios.
Complexity
When it comes to CPU architecture, one of the most critical considerations is the complexity of the system. The complexity of a CPU design can have a significant impact on performance and efficiency.
In general, a more complex CPU architecture may offer more features and capabilities, but it may also be more difficult to design and manufacture. This complexity can result in higher costs and longer development times, which can ultimately lead to higher prices for consumers.
However, complexity is not necessarily a bad thing in all cases. In fact, some CPU architectures are intentionally designed to be highly complex in order to achieve certain performance or efficiency goals.
One example of this is the use of pipelining in modern CPUs. Pipelining involves breaking down CPU instructions into smaller, more manageable chunks and processing them in parallel. While this technique can result in significantly faster processing times, it also adds a significant amount of complexity to the CPU architecture.
Another example is the use of multiple cores in modern CPUs. By using multiple processing cores, CPUs can perform multiple tasks simultaneously, which can result in significantly faster processing times for certain types of applications. However, this also requires a significant amount of additional complexity in the CPU architecture.
Overall, understanding the complexity of CPU architecture is critical for both designers and consumers. By carefully considering the trade-offs between complexity, performance, and efficiency, designers can create CPUs that meet the needs of a wide range of applications. Meanwhile, consumers can make more informed purchasing decisions based on their specific performance and efficiency requirements.
Applications
CPU architecture is a critical component of modern computing systems, and its design has a significant impact on the performance, efficiency, and capabilities of these systems. Here are some real-life examples of CPU architecture and their practical applications:
ARM Architecture: ARM (Advanced RISC Machines) is a popular CPU architecture used in a wide range of devices, including smartphones, tablets, and embedded systems. Its design is focused on low power consumption and high efficiency, making it ideal for battery-powered devices that require long battery life.
x86 Architecture: The x86 architecture is used in many personal computers and servers. It is known for its high performance and ability to execute complex instructions quickly. It is widely used in applications that require high processing power, such as video editing, 3D rendering, and gaming.
Power Architecture: The Power architecture is used in high-performance computing (HPC) systems, such as supercomputers. Its design is focused on parallel processing, making it ideal for applications that require a large number of processing cores working in tandem.
SPARC Architecture: SPARC (Scalable Processor Architecture) is another CPU architecture used in high-performance computing systems. It is known for its ability to handle large datasets and complex workloads, making it ideal for scientific and engineering applications.
MIPS Architecture: MIPS (Microprocessor without Interlocked Pipeline Stages) is a CPU architecture used in many embedded systems, such as routers, modems, and gaming consoles. Its design is focused on low power consumption and high efficiency, making it ideal for devices that require long battery life and efficient operation.
IBM Z Architecture: IBM Z architecture is used in mainframe computers and is known for its high reliability, security, and scalability. It is widely used in applications that require high availability, such as financial systems and transaction processing.
In summary, CPU architecture plays a critical role in modern computing systems, and its design can have a significant impact on the performance, efficiency, and capabilities of these systems. By understanding the real-life examples of CPU architecture and their practical applications, we can better appreciate the importance of this technology in our daily lives.
Questions
Interactive elements can be a great way to enhance learning when it comes to CPU architecture. These interactive elements can include quizzes, games, simulations, and other tools that allow users to engage with the material in a more dynamic and engaging way.
Here are some potential questions that could be used to test knowledge of CPU architecture:
-
What is the purpose of pipelining in CPU architecture, and how does it impact performance?
-
What are some common features of ARM architecture, and in what types of devices is it commonly used?
-
How does the x86 architecture differ from other CPU architectures, and in what types of applications is it commonly used?
-
What is the role of cache memory in CPU architecture, and how does it impact performance?
-
What are some key features of Power architecture, and in what types of applications is it commonly used?
-
What is the role of clock speed in CPU performance, and how does it relate to the overall design of the CPU?
-
What are some key factors to consider when designing a CPU architecture for a specific application or use case?
-
How does the design of a CPU impact power consumption, and what are some strategies for improving energy efficiency in CPU design?
-
What are some common techniques used in modern CPUs to improve performance and efficiency, and how do they work?
-
What are some potential challenges and trade-offs that designers must consider when developing a new CPU architecture?
Discussion
The OpenGenus community is a platform that allows for collaborative learning and sharing of knowledge related to computer science and technology. As part of this community, members can engage in discussions on a variety of topics related to CPU architecture, including its design, performance, and applications.
One potential topic of discussion is the trade-offs that must be considered when designing a CPU architecture for a specific application or use case. This could include considerations such as power consumption, performance, scalability, and reliability, among others. Members of the community could share their insights and experiences related to these trade-offs, as well as discuss the latest trends and developments in CPU architecture design.
Another potential topic of discussion is the impact of CPU architecture on overall system performance and efficiency. Members could discuss the role of CPU architecture in tasks such as data processing, graphics rendering, and machine learning, and share insights on how different CPU architectures can impact performance in these areas.
The OpenGenus community could also provide a platform for sharing best practices and strategies for optimizing CPU performance and efficiency. This could include discussions on techniques such as pipelining, caching, and parallel processing, as well as discussions on how to balance performance and efficiency in different types of applications.
Overall, the OpenGenus community provides a valuable platform for collaborative learning and sharing of knowledge related to CPU architecture and other topics in computer science and technology. By engaging in discussions and sharing their insights and experiences, members can help to advance our understanding of CPU architecture and its role in modern computing systems.
Conclusion
CPU architecture is a core concept in modern computing systems, and its design has a significant impact on performance, efficiency, and capabilities. We have discussed several key concepts related to CPU architecture, including pipelining, cache memory, clock speed, and parallel processing, among others.
We also looked at several examples of CPU architecture and their practical applications, including ARM, x86, Power, SPARC, MIPS, and IBM Z. These architectures are used in a wide range of devices, from smartphones and tablets to supercomputers and mainframe computers, and each architecture has its unique strengths and weaknesses.
Looking to the future, we can expect continued advancements in CPU architecture design, with a focus on improving performance, efficiency, and scalability. These advancements will be driven by the increasing demand for high-performance computing systems for applications such as data analytics, machine learning, and scientific computing.
In addition, we can expect to see continued innovation in areas such as parallel processing, cache management, and power management, as well as increased use of specialized hardware such as graphics processing units (GPUs) and field-programmable gate arrays (FPGAs) to accelerate specific types of workloads.
Overall, the future of CPU architecture is bright, and we can expect to see continued advancements that will enable new and exciting applications in a wide range of fields. By staying up-to-date with the latest developments and trends in CPU architecture, we can better appreciate the critical role that this technology plays in our daily lives.