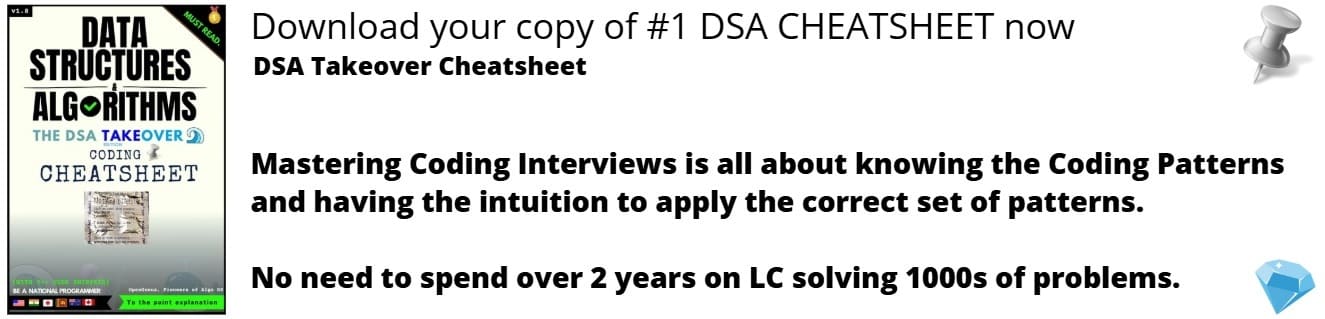
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
CPU core technology is a fundamental concept in modern computer architecture. At the heart of every central processing unit (CPU) lies a CPU core that executes instructions and performs calculations. With the rise of multi-core processors, understanding how to effectively utilize CPU cores has become increasingly important for optimizing software performance. This article provides an overview of CPU core technology, processor architecture, and techniques for optimizing code for multi-core processors. Additionally, real-life applications of multi-core processors and future developments in CPU core technology will also be discussed.
Table of content
- Introduction
- Understanding CPU Core Technology
- Processor Architecture
- Optimizing Software for Multi-Core Processors
- Complexity
- Applications
- Questions and Discussion
- Conclusion
Introduction
CPU Core technology is a critical component of modern computing that has revolutionized the way we interact with our devices. In this section, we will provide a brief overview of the concept of CPU Core, explain why understanding CPU Core technology is important, and clarify the differences between CPU Core, Processor, and CPU.
A CPU Core is a part of a processor that handles instructions and tasks, and multi-core processors have multiple CPU Cores that can work simultaneously to increase performance. Understanding CPU Core technology is important as it enables us to optimize code for multi-core processors and choose the right computer or device for specific tasks. The use of multi-core processors has revolutionized computing by providing faster processing speeds, improved multitasking capabilities, and enhanced overall performance.
It is important to clarify the differences between CPU Core, Processor, and CPU, as these terms can sometimes be used interchangeably, leading to confusion. CPU refers to the entire processing unit in a computer, while Processor refers to a chip that performs the majority of the processing tasks. CPU Core is a component within a Processor that carries out instructions and tasks.
To better illustrate the differences between CPU Core, Processor, and CPU, we will create a custom HTML table that outlines the key features and differences of each. This table will be a useful reference point throughout the article and will be included in the Introduction section.
CPU Core | Processor | CPU | |
---|---|---|---|
Definition | A component within a processor that carries out instructions and tasks | A chip that performs the majority of processing tasks | The entire processing unit in a computer |
Function | Handles instructions and tasks | Performs the majority of processing tasks | Processes data and instructions, communicates with other hardware components |
Examples | Intel Core i7, AMD Ryzen 5 | Intel Core i5, AMD Ryzen 3 | Intel Pentium, AMD Athlon |
The table clearly outlines the definitions and functions of CPU Core, Processor, and CPU, and provides examples of each component. This will serve as a useful reference point throughout the article.
Understanding CPU Core Technology
A CPU core is a processing unit responsible for executing instructions within a computer's central processing unit (CPU). It contains an arithmetic logic unit (ALU) that performs arithmetic and logical operations, as well as a control unit that manages data flow between the CPU, memory, and other components.
Over time, CPU core technology has become increasingly powerful and efficient. Initially, CPUs only had one core, limiting them to executing one instruction at a time. However, with the introduction of multi-core technology, CPUs can now execute multiple instructions simultaneously.
Multi-core processors provide several advantages over single-core processors. Firstly, they can perform more tasks simultaneously, improving system performance and responsiveness. Secondly, they can improve the efficiency of parallel processing tasks, such as rendering graphics or video encoding. Finally, they can reduce power consumption by allowing individual cores to be turned off when not in use.
However, multi-core processors are not without their disadvantages. Some tasks cannot be parallelized, limiting the benefits of additional cores for certain applications. Additionally, multi-core processors can be more expensive to produce, leading to higher costs for consumers.
In summary, CPU core technology has evolved significantly since its inception, with multi-core processors greatly improving the performance and efficiency of modern computers. While there are some downsides to using multi-core processors, their benefits generally outweigh the disadvantages, making them a popular choice for high-performance computing applications.
Processor Architecture
Processor architecture refers to the internal structure of a processor, which includes the CPU cores and how they are designed to process data. Diagrams and illustrations can be useful tools in understanding the inner workings of a CPU.
A diagram of a CPU core typically includes labels for the Arithmetic Logic Unit (ALU), Control Unit, Registers, and other components that work together to execute instructions and perform mathematical calculations. Meanwhile, an illustration of a multi-core processor shows how multiple CPU cores are integrated onto a single chip, along with the interconnects that allow the cores to communicate with each other and share data. This provides a visual representation of how multi-core processors are able to perform multiple tasks simultaneously.
In addition to diagrams and illustrations, comparing single-core and multi-core processors can help illustrate the differences between CPU architectures. Single-core processors can only execute one instruction at a time and are typically slower and less efficient than multi-core processors. On the other hand, multi-core processors can perform multiple tasks simultaneously, which can significantly improve system performance.
However, multi-core processors can also be more complex and expensive to produce and may not be fully utilized by all software applications. Thus, the choice of CPU architecture depends on the specific needs of the application or system in question.
Overall, understanding CPU architecture is essential in selecting the appropriate processor for a given system or application.
Optimizing Software for Multi-Core Processors
To fully leverage the processing power of multi-core processors, software applications must be optimized for parallel processing. This involves dividing tasks into smaller, independent parts that can be executed simultaneously on different CPU cores.
Optimizing software for multi-core processors involves various techniques and strategies that can be used to parallelize code and make it more efficient. Some of these techniques include:
-
Using Parallel Libraries: Parallel libraries such as OpenMP, TBB, and Cilk can quickly and easily parallelize code without requiring major changes to the underlying algorithms. These libraries enable developers to distribute workloads across multiple CPU cores and take advantage of multi-core processors.
-
Multithreading: Multithreading involves creating multiple threads within a single process, each of which can run on a different CPU core. This technique is particularly useful for tasks that can be easily divided into independent sub-tasks, such as image processing or data analysis.
-
SIMD (Single Instruction Multiple Data): SIMD instructions allow a single instruction to operate on multiple data elements simultaneously. This technique can speed up certain types of calculations, such as image and audio processing.
-
Load Balancing: Load balancing involves distributing the workload evenly across all CPU cores to ensure that each core is fully utilized. This technique can improve overall system performance and prevent bottlenecking on a single core.
In summary, optimizing software for multi-core processors requires using various techniques to parallelize code and distribute workloads across multiple CPU cores. Utilizing parallel libraries, multithreading, SIMD instructions, and load balancing can all improve the performance of software on multi-core processors.
Here's an example of a code snippet for a parallelized matrix multiplication using OpenMP in C++:
#include <omp.h>
void matrix_multiplication(int *A, int *B, int *C, int size) {
#pragma omp parallel for
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
int sum = 0;
for (int k = 0; k < size; k++) {
sum += A[i * size + k] * B[k * size + j];
}
C[i * size + j] = sum;
}
}
}
In this code snippet, the matrix multiplication algorithm has been parallelized using OpenMP in C++. The #pragma omp
parallel for directive instructs the compiler to distribute the iterations of the outer loop across multiple threads. This allows the work to be distributed across multiple CPU cores, improving performance on multi-core processors.
Complexity
In this section, we'll explore the complexity of CPU core architecture and how it impacts performance. Factors such as clock speed, cache size, and the number of cores all affect a CPU's ability to handle workloads. Understanding these factors is essential for optimizing code and fully utilizing the potential of multi-core processors.
To measure CPU core performance, we use benchmarking. This involves running tests or programs on a CPU and measuring the time it takes to complete them. Benchmarking provides valuable insights into a CPU's performance and helps us identify areas where we can optimize code to take advantage of multi-core processors.
In summary, understanding the complexity of CPU core architecture and measuring CPU core performance are crucial for optimizing code and fully utilizing the potential of multi-core processors.
Applications
In this section, we'll explore the real-life applications of multi-core processors and how they are used in different industries.
Multi-core processors have become ubiquitous in modern computing and are used in a wide range of applications. They are particularly useful in industries that require high-performance computing, such as finance, engineering, and scientific research.
In the finance industry, multi-core processors are used for high-frequency trading and risk management. These applications require the processing of vast amounts of data in real-time, and multi-core processors can provide the necessary performance to handle this workload.
In the engineering industry, multi-core processors are used for simulations, modeling, and design. These applications require the processing of complex algorithms and equations, and multi-core processors can significantly reduce the time it takes to complete these tasks.
In the scientific research industry, multi-core processors are used for simulations, data analysis, and machine learning. These applications require massive amounts of computational power, and multi-core processors can provide the necessary performance to handle these workloads.
Overall, multi-core processors are a valuable tool in modern computing, thanks to their ability to process large amounts of data quickly and efficiently. As such, they have numerous real-life applications and are used in various industries that require high-performance computing.
Questions
In this section, we'll provide interactive questions to test your knowledge of CPU Core technology and sample practical questions on optimizing code for multi-core processors.
Interactive questions:
- What is a CPU Core, and what is its function?
- What are the advantages and disadvantages of multi-core processors?
- How do multi-core processors differ from single-core processors in terms of performance and functionality?
- What are some real-life applications of multi-core processors?
- How do you measure CPU Core performance?
Sample practical questions:
- Write a C++ code snippet that demonstrates the utilization of multi-core processors.
- What are some techniques for optimizing code for multi-core processors, and how do they work?
- Explain the concept of load balancing in multi-core processors, and how is it achieved?
- What are some common challenges faced when optimizing code for multi-core processors, and how can they be addressed?
- Give an example of an algorithm that can be parallelized to take advantage of multi-core processors, and explain how it can be done.
- These interactive and practical questions are designed to test your understanding of CPU Core technology and multi-core processors and provide practical examples of how to optimize code for multi-core processors.
Discussion
In this section, we can encourage readers to participate in an open discussion on CPU Core technology and its future. This can be done by posing thought-provoking questions, such as "Where do you think the future of CPU Core technology is headed?", "What are the potential advantages and drawbacks of using multi-core processors in various industries?", or "How have you personally benefited from using multi-core processors in your work?", Have you ever encountered a situation where a single-core processor was sufficient for your needs, or where a multi-core processor did not provide a significant performance improvement?" or "What are some new or emerging industries that could benefit from multi-core processors?"
Additionally, readers can be invited to share their experiences on using multi-core processors, such as any challenges they faced in optimizing their code for multi-core processors or any notable improvements in performance they achieved. The goal of this section is to foster a community discussion around CPU Core technology and its practical applications.
Conclusion
In this article, we have explored the key aspects of CPU Core technology, including its definition, function, and evolution. We have also discussed the advantages and disadvantages of multi-core processors and how they compare to single-core processors.
Optimizing code for multi-core processors is critical to achieve maximum performance gains. We have provided sample code snippets and tips for optimizing code for multi-core processors.Without optimization, multi-core processors may not be able to effectively distribute workloads and utilize all available cores
Looking to the future, CPU Core technology is expected to continue advancing rapidly, emerging technologies like quantum computing or specific developments in multi-core processor design. We can expect to see an increasing number of cores and the use of new technologies like machine learning to optimize performance.
In conclusion of this article at OpenGenus, CPU Core technology is an essential aspect of modern computing, and it plays a critical role in shaping the way we interact with technology. Understanding CPU Core technology is necessary for developers and computer enthusiasts alike, A deeper understanding of CPU Core technology allows developers to optimize code for specific hardware and improve performance, while also providing computer enthusiasts with a greater appreciation for the underlying technology that powers modern computing and we hope this article has provided valuable insights into this crucial aspect of computing.