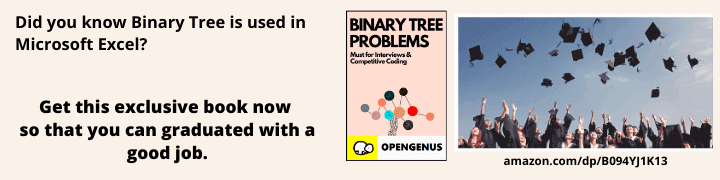
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Polarity and subjectivity are two important concepts in natural language processing (NLP) that help machines understand the sentiment and emotions conveyed in human language. In this article, we'll explore these two concepts in depth and how they are used in NLP.
Table Of Content
- Polarity
- Subjectivity
- Importance of Polarity and Subjectivity in NLP
- Math Logic and Formula Behind Polarity and Subjectivity
- Code Implementation
- Pros and Cons
- Applications of Polarity and Subjectivity Analysis
Polarity in NLP
Polarity refers to the degree of positivity or negativity in a given text. In NLP, polarity analysis is used to determine the sentiment of a text, whether it is positive, negative, or neutral. This can be done using a variety of techniques such as rule-based methods, machine learning algorithms, or deep learning models.
Rule-based methods typically involve defining a set of rules or patterns that identify certain words or phrases that carry a positive or negative connotation. For example, words like "happy," "great," and "awesome" would be considered positive, while words like "sad," "terrible," and "awful" would be considered negative.
Machine learning algorithms and deep learning models, on the other hand, are trained on large datasets of labeled texts to identify patterns and relationships between words and sentiment. These models can be highly accurate in identifying the sentiment of a given text, but they require a large amount of data and computational resources to train.
Subjectivity in NLP
Subjectivity refers to the degree of personal opinion or bias in a given text. In NLP, subjectivity analysis is used to determine whether a text is objective or subjective. This can be important in applications such as news and social media analysis, where it is important to identify whether a particular article or post is providing factual information or expressing a personal opinion.
Subjectivity analysis can be done using a variety of techniques such as lexicon-based approaches, machine learning algorithms, or deep learning models. Lexicon-based approaches involve using a pre-defined set of words and phrases that are commonly associated with subjective language. Machine learning and deep learning models, on the other hand, are trained on large datasets of labeled texts to identify patterns and relationships between words and subjectivity.
Importance of Polarity and Subjectivity in NLP
Polarity refers to the positive, negative, or neutral sentiment expressed in a text. It is used to determine whether a piece of text is expressing a positive or negative sentiment towards a particular entity or topic. In NLP, polarity analysis is often used for sentiment analysis, which is the process of identifying and categorizing opinions expressed in a piece of text.
Subjectivity, on the other hand, refers to how subjective or objective a piece of text is. It is used to determine whether a statement is a fact or an opinion. In NLP, subjectivity analysis can be used to identify subjective language and to differentiate it from objective language. Subjectivity analysis can also be used to identify the degree of objectivity or subjectivity in a text.
Polarity and subjectivity analysis are important because they enable the automatic processing of large amounts of text data. This can be useful in many applications, including social media monitoring, customer feedback analysis, and market research. By analyzing the polarity and subjectivity of text data, NLP systems can extract valuable insights and trends that can be used to inform business decisions or to understand public opinion.
Math Logic and Formula Behind Polarity
polarity is typically represented as a numerical value that indicates the degree of positive, negative, or neutral sentiment expressed in a text. This value is usually assigned using a mathematical formula based on the frequency of certain words or phrases that are associated with positive or negative sentiment. There are several mathematical formulas used in natural language processing (NLP) to calculate the polarity of a piece of text. One common formula for polarity analysis is the following:
Polarity = (Positive score - Negative score) / (Positive score + Negative score)
In this formula, the Positive score and Negative score represent the frequency or intensity of positive and negative sentiment in the text. These scores can be calculated using a sentiment lexicon or other machine learning algorithms that analyze the text data. Each word in the lexicon is assigned a polarity score based on its association with positive or negative sentiment. For example, words like "love" and "happy" might be assigned a high positive polarity score, while words like "hate" and "sad" might be assigned a high negative polarity score.
To calculate the overall polarity of a piece of text, the polarity scores of all the words in the text are added together and then normalized to a scale of -1 to +1, where -1 indicates extremely negative sentiment, +1 indicates extremely positive sentiment, and 0 indicates neutral sentiment. This normalization is typically done by dividing the sum of the polarity scores by the total number of words in the text.
In addition to the sentiment lexicon approach, there are other mathematical models and machine learning algorithms that can be used to calculate the polarity of text data, such as neural networks and support vector machines. These models use a combination of mathematical techniques and statistical analysis to identify patterns in the text data and assign polarity values based on those patterns.
Overall, the math and logic behind polarity analysis in NLP involve using a combination of mathematical formulas, sentiment lexicons, and machine learning algorithms to analyze the frequency and context of words in a text and to assign a numerical polarity value that represents the sentiment expressed in that text.
Math logic Behind Subjectivity
The concept of subjectivity is not strictly tied to math logic, as it deals with personal perspectives and experiences that cannot always be measured or quantified. However, math logic can be used to analyze and understand certain aspects of subjectivity.
One way math logic can be used in relation to subjectivity is through the study of formal logic systems. Formal logic is concerned with the study of reasoning and inference using mathematical symbols and rules. These systems are used to determine whether arguments are valid or not based on the rules of the system.
In the case of subjectivity, formal logic can be used to analyze arguments and determine their validity. However, this analysis is limited to the logical structure of the argument itself and cannot account for subjective factors that may influence one's beliefs or perspectives.
Another way math logic can be used to understand subjectivity is through the study of probability theory. Probability theory is used to quantify the likelihood of an event occurring based on a set of assumptions or prior knowledge. This can be useful in understanding subjective judgments or assessments, such as the likelihood that someone will enjoy a particular movie or book.
However, even with probability theory, there is still an element of subjectivity involved in the selection of assumptions or prior knowledge used in the analysis. Ultimately, while math logic can be a useful tool for analyzing certain aspects of subjectivity, it cannot fully account for the personal experiences and perspectives that shape our subjective beliefs and judgments.
Code Implementation
Polarity
from textblob import TextBlob
# Sample text
text = "I love this product! It works perfectly and has exceeded my expectations."
# Create TextBlob object
blob = TextBlob(text)
# Get polarity score (-1 to 1)
polarity = blob.sentiment.polarity
# Print polarity score
print("Polarity score:", polarity)
# Print sentiment label
if polarity > 0:
print("Sentiment: Positive")
elif polarity < 0:
print("Sentiment: Negative")
else:
print("Sentiment: Neutral")
Output:
Polarity score: 0.625
Sentiment: Positive
In this example, we first import the TextBlob library and create a TextBlob object from a sample text. We then use the sentiment.polarity method to calculate the polarity score of the text, which ranges from -1 (extremely negative) to +1 (extremely positive). Finally, we print the polarity score and a sentiment label based on the polarity value.
Note that this is a basic example and there are many other libraries and tools available for more advanced polarity analysis, including the NLTK library and various machine learning frameworks.
Subjectivity
# import required libraries
from textblob import TextBlob
# sample text to analyze
text = "The movie was great, but the ending was disappointing."
# create a TextBlob object
blob = TextBlob(text)
# get the polarity score of the text
polarity = blob.sentiment.polarity
# determine if the text is subjective or objective
if polarity == 0:
print("The text is objective.")
elif polarity > 0:
print("The text is subjective and positive.")
else:
print("The text is subjective and negative.")
This code takes a sample text as input, creates a TextBlob object, and calculates the polarity score of the text using the TextBlob sentiment method. It then uses the polarity score to determine if the text is subjective or objective, and if it is subjective, whether it is positive or negative.
Note that this is a very basic implementation and can be improved upon by using more advanced techniques and algorithms, as well as by training the model on a larger and more diverse dataset.
Pros and Cons
Polority
Pros of implementing polarity analysis in NLP:
- Can provide insights into the overall sentiment of a piece of text, which can be useful in understanding customer feedback, social media posts, and other forms of text data
- Can be used to identify patterns and trends in sentiment over time or across different groups or demographics
- Can be automated and scaled up to analyze large volumes of text data
- Can be used in conjunction with other NLP techniques like subjectivity analysis and entity recognition to gain a more comprehensive understanding of text data
Cons of implementing polarity analysis in NLP:
- Can be challenging to accurately classify the sentiment of text, especially when dealing with complex and nuanced language
- Can be prone to errors and inaccuracies, especially when dealing with informal language and misspellings
- Can be biased if the training data is not diverse and representative of different groups and perspectives
- May not be suitable for certain types of text data, such as technical manuals or legal documents, where sentiment is not relevant.
Subjectivity
Pros of implementing subjectivity analysis in NLP:
- Can provide insights into the subjective opinions and sentiments of users
- Can be used to analyze customer feedback, social media posts, and other forms of text data to identify trends and patterns
- Can be used in conjunction with other NLP techniques like topic modeling and entity recognition to gain a more comprehensive understanding of text data
- Can be automated and scaled up to analyze large volumes of text data
Cons of implementing subjectivity analysis in NLP:
- Can be challenging to accurately classify text as subjective or objective, especially when dealing with subtle nuances and sarcasm
- Can be prone to errors and inaccuracies, especially when dealing with informal language and misspellings
- Can be biased if the training data is not diverse and representative of different groups and perspectives
- May not be suitable for certain types of text data, such as legal documents or technical manuals, where subjectivity is not relevant.
Applications of Polarity and Subjectivity Analysis
Polarity and subjectivity analysis have a wide range of applications in various industries. Here are some examples:
Applications of Polarity Analysis:
- Social Media Monitoring: Polarity analysis can be used to monitor social media sentiment about a brand or product and identify trends and patterns in customer feedback.
- Customer Service: Polarity analysis can be used to automatically classify customer feedback as positive, negative, or neutral and prioritize responses accordingly.
- Market Research: Polarity analysis can be used to analyze customer feedback and identify emerging trends and changes in consumer sentiment over time.
- Political Analysis: Polarity analysis can be used to analyze political speeches, tweets, and other forms of communication to identify the sentiment of the audience towards specific issues and candidates.
Applications of Subjectivity Analysis:
- Content Curation: Subjectivity analysis can be used to automatically categorize news articles, blog posts, and other content as objective or subjective and prioritize content that is relevant to the user's interests.
- Advertising: Subjectivity analysis can be used to analyze user-generated content and identify influencers who are likely to have a positive impact on a brand's reputation.
- Customer Feedback: Subjectivity analysis can be used to analyze customer feedback and identify specific areas where customers are expressing strong opinions or emotions.
- Market Research: Subjectivity analysis can be used to identify emerging trends and changes in consumer attitudes and opinions over time.
With this article at OpenGenus, you must have the complete idea of Polarity and Subjectivity in NLP.