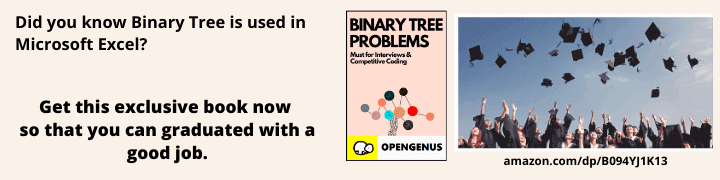
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Natural Language Processing (NLP) is a rapidly growing field in computer science that focuses on making computers understand human language. One of the key tools used in NLP is the N-gram language model.
In this article, we will explore what N-gram models are, how they work, their advantages and disadvantages, and finally, we'll provide an example of how to implement an N-gram model.
What is an N-gram model?
The N-gram model is a statistical language model that estimates the probability of the next word in a sequence based on the previous N-1 words. It works on the assumption that the probability of a word depends only on the preceding N-1 words, which is known as the Markov assumption. In simpler terms, it predicts the likelihood of a word occurring based on its context.
N-grams are essentially sequences of N words or characters. For example, a bigram consists of two words, while a trigram consists of three words. These sequences are then used to calculate the probability of a particular word given its preceding context. In a bigram model, the probability of a word is based on its preceding word only, while in a trigram model, the probability is based on the two preceding words.
How does the N-gram Language Model work?
The N-gram language model works by calculating the frequency of each N-gram in a large corpus of text. The frequency of these N-grams is then used to estimate the probability of a particular word given its context.
For example, let's consider the sentence, "I am going to the grocery store". We can generate bigrams from this sentence by taking pairs of adjacent words, such as "I am", "am going", "going to", "to the", and "the grocery". The frequency of each bigram in a large corpus of text can be calculated, and these frequencies can be used to estimate the probability of the next word given the previous word.
A bigram model is a type of n-gram model where n=2. This means that the model calculates the probability of each word in a sentence based on the previous word.
To calculate the probability of the next word given the previous word(s) in a bigram model, we use the following formula:
P(w_n | w_{n-1}) = count(w_{n-1}, w_n) / count(w_{n-1})
where P(w_n | w_{n-1}) is the probability of the nth word given the previous word, and count(w_{n-1}, w_n) is the count of the bigram (w_{n-1}, w_n) in the text, and count(w_{n-1}) is the count of the previous word in the text.
For example, let's say we have the sentence: "The cat sat on the mat." In a bigram model, we would calculate the probability of each word based on the previous word:
P("The" | Start) = 1 (assuming "Start" is a special token indicating the start of a sentence)
P("cat" | "The") = 1
P("sat" | "cat") = 1
P("on" | "sat") = 1
P("the" | "on") = 1
P("mat" | "the") = 0.5 (assuming "the" appears twice in the sentence)
The probability of each word given the previous word can be used to generate new sentences or to evaluate the likelihood of a given sentence.
A trigram model is similar to a bigram model, but it calculates the probability of each word based on the previous two words. To calculate the probability of the next word given the previous two words in a trigram model, we use the following formula:
P(w_n | w_{n-2}, w_{n-1}) = count(w_{n-2}, w_{n-1}, w_n) / count(w_{n-2}, w_{n-1})
where P(w_n | w_{n-2}, w_{n-1}) is the probability of the nth word given the previous two words, and count(w_{n-2}, w_{n-1}, w_n) is the count of the trigram (w_{n-2}, w_{n-1}, w_n) in the text, and count(w_{n-2}, w_{n-1}) is the count of the previous two words in the text.
For example, let's say we have the sentence: "I love to eat pizza." In a trigram model, we would calculate the probability of each word based on the previous two words:
P("I" | Start, Start) = 1
P("love" | Start, "I") = 0
P("to" | "I", "love") = 1
P("eat" | "love", "to") = 1
P("pizza" | "to", "eat") = 1
Again, the probability of each word given the previous two words can be used to generate new sentences or to evaluate the likelihood of a given sentence. However, trigram models may be less accurate than bigram models because they require more context to calculate the probability of each word.
Implementing the N-gram Language Model
To implement an N-gram language model, we first need to tokenize the input text. Tokenization is the process of breaking down the text into words or characters. Once the text is tokenized, we can generate N-grams by taking sequences of N consecutive tokens.
Here is a basic implementation of an N-gram language model in Python:
Step 1: Preprocessing the Data
Before building an N-gram language model, we need to preprocess the data to clean it and convert it into a suitable format. Here are some common preprocessing steps:
Tokenize the text corpus into individual words or subwords.
Remove punctuation, stop words, and other non-relevant characters.
Convert all words to lowercase to avoid inconsistencies.
Step 2: Building the N-gram Language Model
Once the data is preprocessed, we can build the N-gram language model. Here's an example of how to build a bigram language model:
import nltk
from nltk.util import ngrams
from collections import defaultdict
# Sample text corpus
text = "This is a sample text corpus for N-gram language model implementation."
# Tokenize the text corpus into individual words
tokens = nltk.word_tokenize(text)
# Create a bigram language model
n = 2
bigrams = ngrams(tokens, n, pad_left=True, pad_right=True)
# Initialize a dictionary to store bigram counts
bigram_counts = defaultdict(int)
# Count the occurrences of each bigram in the text corpus
for bigram in bigrams:
bigram_counts[bigram] += 1
# Calculate the probability of each bigram
total_bigrams = len(tokens) - 1
bigram_probs = defaultdict(float)
for bigram in bigram_counts:
bigram_probs[bigram] = bigram_counts[bigram] / total_bigrams
In this example, we use the nltk library to tokenize the text corpus into individual words, and the ngrams function to create bigrams. We then initialize a dictionary to store the bigram counts and count the occurrences of each bigram in the text corpus. Finally, we calculate the probability of each bigram by dividing its count by the total number of bigrams in the text corpus.
Step 3: Using the N-gram Language Model
Once the N-gram language model is built, we can use it to generate text, predict the probability of a sequence of words, and more. Here's an example of how to use the bigram language model to generate text:
import random
# Generate a random sentence using the bigram language model
sentence = ["This"]
while sentence[-1] != ".":
prev_word = sentence[-1]
next_word = max(bigram_probs, key=lambda x: bigram_probs[x] if x[0] == prev_word else 0)
sentence.append(next_word[1])
print(" ".join(sentence))
In this example, we start with the word "This" and repeatedly choose the next word based on the probability of each bigram that starts with the previous word. We stop when we reach a period. Finally, we join the words together to form a sentence.
Note that this is just a basic example of how to implement an N-gram language model. In practice, you may want to use more advanced techniques, such as smoothing, to improve the accuracy of the model.
Applications of N-gram Language Model
The N-gram language model has numerous applications in Natural Language Processing. Some of the popular applications are:
Speech Recognition
In speech recognition, the N-gram language model is used to predict the next word in a spoken sentence. This helps in improving the accuracy of the transcription.
Machine Translation
In machine translation, the N-gram language model is used to predict the most likely translation of a sentence based on its context.
Text Classification
In text classification, the N-gram language model is used to classify a text document into different categories based on its content.
Information Retrieval
In information retrieval, the N-gram language model is used to rank search results based on their relevance to the query.
Pros:
N-gram language model is easy to understand and implement.
It is computationally efficient and can be used for real-time applications.
It can handle large amounts of text data and provide accurate results.
Cons:
N-gram language model suffers from the sparsity problem, where some N-grams may not occur in the training corpus, resulting in zero probabilities. This problem can be addressed using smoothing techniques such as Laplace smoothing and Good-Turing smoothing.
N-gram language model assumes that the probability of a word depends only on its previous N-1 words, which is not always true in real-world scenarios.
N-gram language model does not capture the semantic meaning of words and cannot handle the ambiguity of language.
With this article at OpenGenus, you must have the complete idea of N-gram language model in NLP.