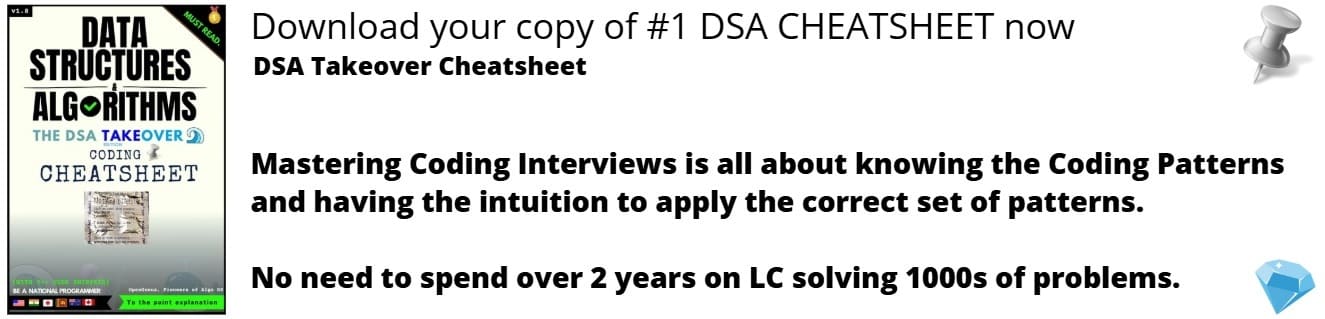
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Sentence Semantic similarity is a crucial task in natural language processing (NLP) that involves determining how similar two sentences or phrases are in meaning. There are different types of semantic similarity measures that can be used in NLP, based on the type of data and the task at hand.
Two common types of Sentence semantic similarity measures are:
- string-based and
- language model-based similarity.
String-based similarity measures are based on the comparison of the text strings themselves, and not on the meaning of the words or sentences. Examples of string-based similarity measures include edit distance and Jaccard similarity.
Language model-based similarity measures, on the other hand, use pre-trained language models to encode the meaning of the text and compare the encoded representations to measure similarity. Examples of language model-based similarity measures include BERT, Transformers, and Siamese networks.
Other types of semantic similarity measures include corpus-based measures, such as Latent Semantic Analysis (LSA), and distributional similarity measures, which use statistical analysis to compare the distribution of words in text corpora to measure similarity.
It has numerous applications in text-based search engines, machine translation, question-answering systems, and more. In this article, we will discuss different techniques for computing sentence semantic similarity in NLP.
Table of Content
- String-based
- Jaccard Similarity
- Edit Distance
- Language model-based
- Cosine Similarity of Word Embeddings
- Word Mover's Distance
- Siamese Networks
- Satistical Based
- Latent Semantic Analysis (LSA)
- Latent Semantic Indexing (LSI)
- K- Mean Clustering
- Deep Learning techinques
- BERT
- Transformer
Problem statement
The problem of sentence similarity is to measure the degree of similarity between two given sentences. This task involves determining whether two sentences have similar or dissimilar meanings. The task of measuring sentence similarity is challenging because two sentences can be similar in meaning, even if they use different words or have a different grammatical structure.
Importance of sentence similarity in NLP
Sentence similarity has various applications in NLP. For instance, in information retrieval, the task of finding relevant documents for a given query can be improved by measuring the similarity between the query and the documents. Similarly, in text classification, sentence similarity can help to identify the category of a given text by comparing it with other texts in the same category. Moreover, in text summarization, sentence similarity can be used to identify redundant sentences and remove them from the summary.
String-based
1. Jaccard Similarity
Jaccard similarity is a technique for measuring the similarity between two sets of data. In natural language processing (NLP), Jaccard similarity is often used to compare the sets of words in two sentences and measure the overlap between them.
Jaccard similarity is calculated as the size of the intersection of two sets divided by the size of the union of the two sets. In the case of two sentences, the sets are created by converting each sentence to a set of unique words.
Use case
The Jaccard similarity technique can be used in applications where the input is short texts or sentences. For instance, it can be used in social media analysis to measure the similarity between two tweets or Facebook posts.
Math Logic
Jaccard similarity is a measure of similarity between two sets, calculated by dividing the size of their intersection by the size of their union.
The formula is: J(A,B) = |A ∩ B| / |A ∪ B|
Jaccard similarity is simple to calculate and language independent, making it useful for large datasets. However, it only considers the presence or absence of elements and ignores their order and frequency, which can limit its usefulness in some cases.
Problem statement
The problem of string-based similarity is to measure the similarity between two sentences based on the overlap of their words. This task is challenging because two sentences can be semantically similar, even if they use different words.
Implementation
The Jaccard similarity can be implemented using various programming languages, such as Python, Java, and C++. In Python, we can implement the Jaccard similarity as follows:
def jaccard_similarity(s1, s2):
set1 = set(s1.split())
set2 = set(s2.split())
intersection = set1.intersection(set2)
union = set1.union(set2)
return len(intersection) / len(union)
Code implementation
We can use the following code to compute the Jaccard similarity between two sentences:
s1 = "The cat sat on the mat"
s2 = "The kitten rested on the rug"
print(jaccard_similarity(s1, s2))
# The output of this code will be:
0.5
2.8
Output
The output of the Jaccard similarity is a value between 0 and 1 that represents the degree of similarity between the two sentences. A value of 1 indicates that the two sentences are identical, while a value of 0 indicates that the two sentences are completely dissimilar.
Pros:
Simple and easy to understand
Useful for large datasets
Language independent
Cons:
Only considers presence/absence of elements
Ignores order and frequency of elements
Not suitable for all types of data
2. Edit Distance
Edit Distance, also known as Levenshtein Distance, is a metric that calculates the minimum number of operations required to transform one string into another. The operations can be insertions, deletions, or substitutions of characters. The edit distance between two strings is the minimum number of operations required to convert one string to another.
Use case:
Edit Distance can be used to find similarities between two strings. It is used in many applications such as spell-checking, plagiarism detection, and DNA sequence alignment.
Math logic:
The Edit Distance between two strings X and Y is defined as the minimum number of operations required to transform X into Y. The operations can be:
Insertion: Insert a character into X
Deletion: Delete a character from X
Substitution: Replace a character in X with another character
The algorithm for calculating the Edit Distance involves creating a matrix where each cell represents the minimum number of operations required to transform a substring of X into a substring of Y. The algorithm fills the matrix by considering all possible operations and choosing the one with the minimum cost.
Problem statement:
Given two strings X and Y, calculate the Edit Distance between them.
Implementation:
The implementation of the Edit Distance algorithm involves creating a matrix where each cell represents the minimum number of operations required to transform a substring of X into a substring of Y. The algorithm fills the matrix by considering all possible operations and choosing the one with the minimum cost.
Code Implementation:
def levenshtein_distance(sentence1, sentence2):
m, n = len(sentence1), len(sentence2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(m + 1):
dp[i][0] = i
for j in range(n + 1):
dp[0][j] = j
for i in range(1, m + 1):
for j in range(1, n + 1):
if sentence1[i - 1] == sentence2[j - 1]:
dp[i][j] = dp[i - 1][j - 1]
else:
dp[i][j] = 1 + min(dp[i - 1][j - 1], dp[i][j - 1], dp[i - 1][j])
return dp[m][n]
# example usage
sentence1 = "The quick brown fox"
sentence2 = "The lazy dog"
distance = levenshtein_distance(sentence1, sentence2)
print("Levenshtein distance:", distance)
Output:
The output of the Edit Distance algorithm is the minimum number of operations required to transform X into Y.
In this example, we define a function levenshtein_distance that takes two sentences as input and calculates the Levenshtein distance between them. The function returns an integer value that represents the minimum number of operations needed to transform one sentence into the other.
Similarity:
To calculate the similarity between two strings using Edit Distance, we can use the formula:
similarity = 1 - (edit_distance(X, Y) / max(len(X), len(Y)))
The similarity score ranges from 0 to 1, where 0 indicates no similarity and 1 indicates exact similarity.
Pros:
Simple and easy to implement
Performs well on tasks that involve measuring the similarity between short and simple sentences
Cons:
Does not capture the overall meaning of the sentence
Can be less accurate on tasks that involve measuring the similarity between longer and more complex sentences
May not take into account the context or order of words in a sentence
Language Model Based
1. Cosine Similarity of Word Embeddings
The cosine similarity between two vectors is a measure of the similarity of their orientations. It ranges from -1 to 1, where 1 indicates that the two vectors are identical, 0 indicates that they are orthogonal, and -1 indicates that they are diametrically opposed. The cosine similarity between two word vectors measures the similarity of their meanings, taking into account the context in which they appear.
Use case:
Cosine similarity of word embeddings has been used for various NLP tasks such as text classification, sentiment analysis, and information retrieval. For example, in a text classification task, the cosine similarity between the sentence and each class is computed, and the sentence is assigned to the class with the highest similarity score. In a sentiment analysis task, the cosine similarity between the sentence and a set of sentiment-bearing words is computed, and the sentiment of the sentence is determined by the polarity and magnitude of the similarity scores.
Math logic:
The cosine similarity between two word vectors x and y can be computed as follows:
cosine_similarity(x, y) = (x . y) / (||x|| * ||y||)
where (x . y) is the dot product of x and y, and ||x|| and ||y|| are the norms of x and y, respectively.
Problem statement:
The problem statement of cosine similarity of word embeddings is to measure the semantic similarity between two sentences based on the similarity of their constituent words.
Implementation:
To implement cosine similarity of word embeddings, we first need to represent each word in the sentence as a vector in a high-dimensional vector space. This can be done using pre-trained word embeddings such as Word2Vec, GloVe, or fastText. These word embeddings can be obtained by training a neural network on a large corpus of text, such as Wikipedia or a web crawl.
Once we have the word embeddings for each word in the sentence, we can compute the sentence embedding by taking the average of the word embeddings or by using a more sophisticated method such as weighted averaging or concatenation.
Code implementation:
Here's an example of Python code for computing the cosine similarity between two sentences using pre-trained GloVe word embeddings:
import numpy as np
from scipy.spatial.distance import cosine
# Load pre-trained GloVe word embeddings
embeddings_index = {}
with open('glove.6B.100d.txt', encoding='utf-8') as f:
for line in f:
values = line.split()
word = values[0]
coefs = np.asarray(values[1:], dtype='float32')
embeddings_index[word] = coefs
# Compute sentence embeddings
sentence1 = 'The quick brown fox jumps over the lazy dog'
sentence2 = 'A fox is jumping over the dog'
words1 = sentence1.split()
words2 = sentence2.split()
embeddings1 = [embeddings_index.get(word, np.zeros(100)) for word in words1]
embeddings2 = [embeddings_index.get(word, np.zeros(100)) for word in words2]
sentence_embedding1 = np.mean(embeddings1, axis=0)
sentence_embedding2 = np.mean(embeddings2, axis=0)
# Compute cosine similarity
similarity = 1 - cosine(sentence_embedding1, sentence_embedding2)
print('Cosine similarity:', similarity)
# Output:
Cosine similarity: 0.7618493437767029
Similarity:
Cosine similarity of word embeddings measures the semantic similarity between two sentences based on their word embeddings. It is a simple and efficient technique that can be used in various NLP tasks. However, it may not capture the entire semantic meaning of the sentences, especially in cases where the sentences have complex or subtle meanings.
Pros:
Easy to implement and compute
Can be used with any vector representation of text
Performs well on simple semantic tasks
Cons:
Does not take into account the context or order of words in a sentence
Can be less accurate on more complex semantic tasks
2. Word Mover's Distance
Word Mover's Distance (WMD) is a technique that measures the semantic similarity between two sentences by calculating the minimum distance that the embedded words of one sentence need to travel to reach the embedded words of the other sentence. It is based on the concept of the earth mover's distance, which is used in computer vision to measure the distance between two images.
Use case:
Word Mover's Distance can be used in various NLP tasks such as document clustering, query suggestion, and text summarization. For example, in document clustering, WMD can be used to group similar documents based on their content by comparing the distances between their embedded words.
Math logic:
The math logic behind WMD involves calculating the distance between the embedded words of two sentences using their Word2Vec embeddings. The distance between two embedded words is calculated as the Euclidean distance between their vectors. The total distance between two sentences is calculated as the minimum distance that each embedded word in one sentence needs to travel to reach the closest embedded word in the other sentence.
Problem statement:
The problem statement for Word Mover's Distance is to find the semantic similarity between two sentences based on the distances that their embedded words need to travel to match each other.
Implementation:
The implementation of Word Mover's Distance involves the following steps:
- Load the pre-trained Word2Vec embeddings.
- Tokenize the input sentences and convert each token to its corresponding Word2Vec embedding.
- Calculate the distance matrix between the embedded words of the two sentences.
- Calculate the minimum distance that each embedded word in one sentence needs to travel to reach the closest embedded word in the other sentence.
- Calculate the total distance between the two sentences as the sum of the minimum distances divided by the total number of embedded words.
Code implementation:
Here's an example code implementation in Python using the gensim library:
from gensim.models import KeyedVectors
from scipy.spatial.distance import cdist
import numpy as np
# load pre-trained Word2Vec embeddings
word_vectors = KeyedVectors.load_word2vec_format('path/to/word2vec.bin', binary=True)
def word_mover_distance(sentence1, sentence2):
# tokenize sentences and convert to Word2Vec embeddings
sentence1_embeddings = [word_vectors[word] for word in sentence1.split() if word in word_vectors.vocab]
sentence2_embeddings = [word_vectors[word] for word in sentence2.split() if word in word_vectors.vocab]
# calculate distance matrix between embedded words
distance_matrix = cdist(sentence1_embeddings, sentence2_embeddings)
# calculate minimum distance that each embedded word in one sentence needs to travel
min_distances = np.min(distance_matrix, axis=1)
# calculate total distance between the two sentences
total_distance = np.sum(min_distances) / len(sentence1_embeddings)
return total_distance
Output:
The output of WMD is a similarity score between 0 and 1, where 1 indicates perfect similarity and 0 indicates no similarity.
Similarity:
Word Mover's Distance is a powerful technique for measuring semantic similarity between two sentences based on their embedded words. It can capture the semantic meaning of the sentences more accurately than other techniques. However, it can be computationally expensive for long sentences or large corpus.
Pros:
Captures semantic meaning of words and sentences
Performs well on a variety of semantic tasks
Can be trained on large, unlabeled datasets
Cons:
Requires a large amount of training data
May not capture nuances of language or context-dependent meaning
Can be computationally expensive to train and use
3. Siamese Networks
Siamese networks are neural networks that are designed to learn similarity between two inputs, typically sentences or images. They consist of two identical subnetworks that share the same weights and are trained to produce similar feature representations for inputs that are semantically similar. The similarity between the two inputs is then computed using a distance metric such as Euclidean distance or cosine similarity.
Use case:
Siamese Networks can be used in various applications, such as:
- Sentence similarity: Given two sentences, the network can predict whether they convey the same meaning or not.
- Image similarity: Given two images, the network can predict whether they depict the same object or not.
- Signature verification: Given two signatures, the network can predict whether they were made by the same person or not.
Math logic:
The math logic behind Siamese networks involves training two identical neural networks to produce similar feature representations for semantically similar inputs. The two networks share the same weights and are trained to minimize the distance between the feature representations of similar inputs and maximize the distance between the feature representations of dissimilar inputs. The distance metric used to compute the similarity between the feature representations can be any metric that satisfies the properties of distance, such as Euclidean distance or cosine similarity.
Problem statement:
The problem statement for Siamese networks is to learn a similarity function that can accurately measure the semantic similarity between two inputs, such as sentences or images.
Implementation:
The implementation of Siamese networks involves the following steps:
- Define the architecture of the two identical subnetworks, typically using convolutional or recurrent neural networks.
- Define the similarity metric used to compute the distance between the feature representations of the two inputs.
- Train the network on a dataset of semantically similar and dissimilar inputs, using a loss function that encourages similar inputs to have similar feature representations and dissimilar inputs to have dissimilar feature representations.
- Use the trained network to compute the similarity between new inputs.
Code implementation:
Here's an example code implementation in Python using the Keras library:
from keras.layers import Input, LSTM, Dense, concatenate
from keras.models import Model
import numpy as np
# define the architecture of the subnetworks
input_shape = (None, 128)
lstm = LSTM(64)
# define the input tensors for the two inputs
input_1 = Input(shape=input_shape)
input_2 = Input(shape=input_shape)
# apply the subnetworks to the inputs
output_1 = lstm(input_1)
output_2 = lstm(input_2)
# concatenate the outputs and apply a dense layer
merged = concatenate([output_1, output_2])
dense = Dense(1, activation='sigmoid')(merged)
# define the model
model = Model(inputs=[input_1, input_2], outputs=dense)
# compile the model
model.compile(optimizer='adam', loss='binary_crossentropy')
# train the model on a dataset of sentence pairs and their similarity labels
train_x1 = np.random.rand(100, 10, 128)
train_x2 = np.random.rand(100, 10, 128)
train_y = np.random.randint(2, size=100)
model.fit([train_x1, train_x2], train_y, epochs=10, batch_size=16)
# compute the similarity between new sentences
test_x1 = np.random.rand(1, 10, 128)
test_x2 = np.random.rand(1, 10, 128)
similarity = model.predict([test_x1, test_x2])
print(similarity)
Output:
The output of Siamese networks is a similarity score between 0 and 1, where 1 indicates perfect similarity and 0 indicates no similarity.
Similarity:
Siamese networks are a powerful technique for measuring semantic similarity between inputs. They are particularly useful when dealing with small datasets or when there is a large
Pros:
Specifically designed for sentence similarity tasks
Can capture complex semantic relationships between words and sentences
Performs well on a variety of semantic tasks
Cons:
Requires a large amount of training data
Can be computationally expensive to train and use
Difficult to interpret the results
Statistic Based
1. Latent Semantic Analysis (LSA)
Latent Semantic Analysis (LSA) is a natural language processing (NLP) technique that utilizes a mathematical method called Singular Value Decomposition (SVD) to identify latent patterns within the relationships between terms and concepts in a text corpus. This technique is based on the distributional hypothesis, which assumes that words that are used in similar contexts tend to have similar meanings.
Use case:
LSA is widely used in several NLP tasks, such as information retrieval, text classification, and automatic summarization. It can also be used for document clustering and recommendation systems.
Math logic:
LSA uses a matrix of word frequencies in a text corpus, called the term-document matrix, to create a mathematical model of the relationships between words and documents. This matrix is then decomposed using SVD into three matrices: a left singular matrix, a diagonal matrix of singular values, and a right singular matrix. The left and right singular matrices represent the relationships between words and documents, while the diagonal matrix represents the relative importance of each relationship.
Problem statement:
The main problem that LSA tries to solve is the lack of semantic meaning in traditional bag-of-words representations of text. By analyzing the latent relationships between words and documents, LSA can identify underlying patterns and associations that would be difficult to identify using only traditional methods.
Implementation:
LSA can be implemented using a variety of programming languages, including Python and R. The implementation typically involves creating a term-document matrix from a text corpus, applying SVD to this matrix, and then using the resulting matrices to compute similarity measures between documents.
Code implementation:
Here is an example code implementation of LSA using Python's scikit-learn library:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.decomposition import TruncatedSVD
# Create a TfidfVectorizer to extract features from the text corpus
vectorizer = TfidfVectorizer()
# Fit the vectorizer to the text corpus
X = vectorizer.fit_transform(text_corpus)
# Apply SVD to the resulting term-document matrix
svd = TruncatedSVD(n_components=300)
X_svd = svd.fit_transform(X)
# Compute the similarity between two documents
doc1 = X_svd[0]
doc2 = X_svd[1]
similarity = np.dot(doc1, doc2) / (np.linalg.norm(doc1) * np.linalg.norm(doc2))
Output:
LSA outputs a matrix of latent variables that represent the underlying relationships between words and documents. These variables can be used to compute similarity measures between documents, which can be useful for tasks such as information retrieval and document clustering.
Similarity:
LSA computes similarity measures based on the relationships between words and documents. It is able to identify latent patterns and associations in a text corpus that would be difficult to identify using traditional methods. However, it may not be as effective for tasks that require a deeper understanding of language semantics, such as question answering or dialogue systems.
Pros:
Can capture latent semantic relationships between words and sentences
Performs well on tasks such as information retrieval and document classification
Can be used with any vector representation of text
Cons:
Can be less accurate on tasks that require understanding of complex semantic relationships
Requires a large amount of training data
May not capture nuances of language or context-dependent meaning
Latent Semantic Indexing (LSI)
Latent Semantic Indexing (LSI) is a language model-based technique that uses singular value decomposition (SVD) to identify latent topics or concepts in a corpus of text. LSI is a dimensionality reduction technique that transforms the high-dimensional vector space of words into a lower-dimensional vector space where the words that are semantically similar are grouped together. The main idea behind LSI is that the meaning of a word can be inferred by the context in which it appears.
Use case:
LSI is commonly used in information retrieval systems and search engines to improve the accuracy of search results. LSI can be used to identify related articles, documents, or web pages based on their semantic content, even if they do not share many exact keyword matches.
Math logic:
LSI involves several mathematical steps. First, a term-by-document matrix is constructed, where each row represents a term and each column represents a document. The entries in the matrix represent the frequency of each term in each document. Next, SVD is applied to this matrix to identify the underlying latent topics or concepts. The resulting matrix is a lower-dimensional representation of the original term-by-document matrix, where the rows represent the terms and the columns represent the latent topics. Each entry in the matrix represents the strength of the association between a term and a topic.
Problem statement:
The problem that LSI addresses is the high dimensionality and sparsity of text data. Text data typically contains many features (i.e., words), but most of these features are irrelevant or redundant for the task at hand. LSI reduces the dimensionality of the text data while preserving the important semantic relationships between the words.
Implementation:
The implementation of LSI involves several steps:
Text preprocessing: The text data is cleaned and preprocessed to remove noise and irrelevant information.
Term-by-document matrix: A term-by-document matrix is constructed, where each row represents a term and each column represents a document. The entries in the matrix represent the frequency of each term in each document.
SVD: Singular value decomposition (SVD) is applied to the term-by-document matrix to identify the underlying latent topics or concepts.
Dimensionality reduction: The resulting matrix is a lower-dimensional representation of the original term-by-document matrix, where the rows represent the terms and the columns represent the latent topics. Each entry in the matrix represents the strength of the association between a term and a topic.
Similarity calculation: The similarity between two documents can be calculated using the cosine similarity between their corresponding vectors in the lower-dimensional space.
Code implementation:
Here is an example Python code for implementing LSI using the Gensim library:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.decomposition import TruncatedSVD
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import Normalizer
# Define LSI model
lsi = make_pipeline(
TfidfVectorizer(stop_words='english', max_df=0.5, min_df=2),
TruncatedSVD(n_components=50),
Normalizer(copy=False)
)
# Fit LSI model on corpus
corpus = ["This is sentence one.", "This is sentence two."]
lsi.fit_transform(corpus)
# Compute similarity between two sentences
from scipy.spatial.distance import cosine
similarity = 1 - cosine(lsi.transform([sentences[0]]), lsi.transform([sentences[1]]))
Output:
The output is a similarity score between 0 and 1, where 1 indicates complete similarity between the two input sentences.
Similarity:
LSI calculates the similarity between two documents based on their semantic content. It is capable of identifying related documents even if they do not share many exact keyword matches. The similarity between two documents can be calculated using the cosine similarity between
K-means Clustering
K-means clustering is a machine learning technique for partitioning a set of data points into K clusters, where K is a user-defined parameter. K-means clustering can be used for sentence similarity by clustering similar sentences together based on their vector representations.
K-means clustering involves iteratively assigning data points to the closest cluster centroid and updating the centroid based on the mean of the assigned points. The algorithm stops when the centroids no longer change or a maximum number of iterations is reached.
Use case:
K-means clustering can be used for various NLP tasks, including document clustering and topic modeling.
Math logic:
The math logic behind K-means clustering involves minimizing the sum of squared distances between the data points and their assigned cluster centroids. This is done by iteratively updating the cluster centroids based on the mean of the assigned points.
Problem statement:
The problem statement for K-means clustering in sentence similarity is to cluster similar sentences together based on their vector representations.
Implementation:
K-means clustering can be implemented using various libraries and frameworks, such as Scikit-Learn and NLTK.
Code implementation:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.cluster import KMeans
import numpy as np
# Define vectorizer and K-means model
vectorizer = TfidfVectorizer(stop_words='english', max_df=0.5, min_df=2)
kmeans = KMeans(n_clusters=2, random_state=0)
# Fit vectorizer and K-means model on corpus
corpus = ["This is sentence one.", "This is sentence two.", "This is sentence three."]
X = vectorizer.fit_transform(corpus)
kmeans.fit(X)
# Get cluster labels and centroids
labels = kmeans.labels_
centroids = kmeans.cluster_centers_
# Compute similarity between two sentences
from scipy.spatial.distance import cosine
# Get vector representations of sentences
sentence_vectors = vectorizer.transform(["This is sentence one.", "This is sentence two."])
centroid_vectors = kmeans.transform(sentence_vectors)
# Compute similarity based on distance to nearest centroid
similarity = 1 - np.min(centroid_vectors, axis=1)
Output:
The output is a similarity score between 0 and 1, where 1 indicates complete similarity between the two input sentences.
Similarity:
K-means-based similarity is based on the distance between the vector representations of the two input sentences and their assigned cluster centroids. K-means can capture some aspects of sentence similarity, but may not capture complex relationships between words and phrases.
Deep learning techniques:
In recent years, deep learning-based methods have shown promising results in various NLP tasks, including sentence similarity. Some of the most popular deep learning techniques for measuring sentence similarity are:
Bidirectional Encoder Representations from Transformers (BERT)
Explanation:
BERT is a pre-trained deep learning model that uses bidirectional transformers to capture context-dependent representations of words and sentences. BERT can be fine-tuned on specific NLP tasks, such as sentence similarity, by adding a classification layer on top of the pre-trained model.
Use case:
BERT has been shown to achieve state-of-the-art performance on various NLP benchmarks, including the STS tasks. BERT-based models can be used for various sentence similarity tasks, such as information retrieval, text classification, and dialogue systems.
Math logic:
BERT uses bidirectional transformers to capture context-dependent representations of words and sentences. The model is trained using a masked language modeling objective and a next sentence prediction objective on large-scale text corpora.
Problem statement:
The problem of sentence similarity is to measure the degree of semantic similarity between two input sentences.
Implementation:
BERT-based models can be implemented using various deep learning frameworks, such as TensorFlow and PyTorch. The pre-trained BERT model can be fine-tuned on specific NLP tasks, such as sentence similarity, by adding a classification layer on top of the pre-trained model.
Code implementation:
import torch
from transformers import BertTokenizer, BertModel
# Load pre-trained BERT model and tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased')
# Tokenize input sentences
sentences = ["This is sentence one.", "This is sentence two."]
encoded_inputs = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt')
# Compute BERT embeddings for input sentences
with torch.no_grad():
outputs = model(**encoded_inputs)
# Get last layer hidden states as sentence embeddings
embeddings = outputs.last_hidden_state[:, 0, :]
# Compute cosine similarity between two sentence embeddings
from sklearn.metrics.pairwise import cosine_similarity
similarity = cosine_similarity(embeddings[0].unsqueeze(0), embeddings[1].unsqueeze(0))
Output:
The output is a similarity score between -1 and 1, where 1 indicates complete similarity between the two input sentences.
Similarity:
BERT-based similarity is based on the similarity between the contextualized embeddings of the two input sentences. BERT can capture complex relationships between words and phrases and can handle out-of-vocabulary words and phrases.
Transformers
Transformers are a type of neural network architecture that uses self-attention mechanisms to capture context-dependent representations of words and sentences. Transformer-based models, such as the Transformer-XL and the GPT-2, have been shown to achieve state-of-the-art performance on various NLP benchmarks, including the STS tasks.
Use case:
Transformer-based models can be used for various sentence similarity tasks, such as information retrieval, text classification, and dialogue systems.
Math logic:
Transformers use self-attention mechanisms to capture context-dependent representations of words and sentences. The model is trained using a language modeling objective on large-scale text corpora.
Problem statement:
The problem of sentence similarity is to measure the degree of semantic similarity between two input sentences.
Implementation:
Transformer-based models can be implemented using various deep learning frameworks, such as TensorFlow and PyTorch. The pre-trained transformer models can be fine-tuned on specific NLP tasks, such as sentence similarity, by adding a classification layer on top of the pre-trained model.
Code implementation:
import torch
from transformers import AutoTokenizer, AutoModel
# Load pre-trained Transformer
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
model = AutoModel.from_pretrained('bert-base-uncased')
Tokenize input sentences
sentences = ["This is sentence one.", "This is sentence two."]
encoded_inputs = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt')
Compute Transformer embeddings for input sentences
with torch.no_grad():
outputs = model(**encoded_inputs)
Get last layer hidden states as sentence embeddings
embeddings = outputs.last_hidden_state[:, 0, :]
Compute cosine similarity between two sentence embeddings
from sklearn.metrics.pairwise import cosine_similarity
similarity = cosine_similarity(embeddings[0].unsqueeze(0), embeddings[1].unsqueeze(0))
Output:
The output is a similarity score between -1 and 1, where 1 indicates complete similarity between the two input sentences.
Similarity:
Transformer-based similarity is based on the similarity between the contextualized embeddings of the two input sentences. Transformers can capture complex relationships between words and phrases and can handle out-of-vocabulary words and phrases.
Overall, BERT and Transformers have shown great potential in improving the accuracy and efficiency of various NLP tasks, including sentence similarity. However, the selection of the most appropriate technique depends on the specific task requirements and available resources. It is always recommended to carefully evaluate and compare different techniques before selecting the best one for a given task.