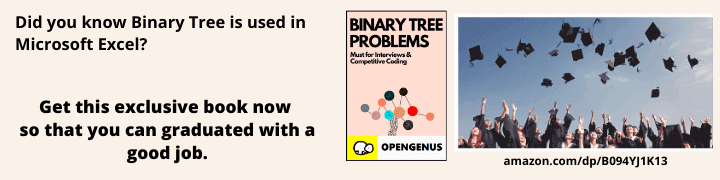
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
Managing and organizing student data is an essential aspect of any educational institution.
- As the number of students increases, it becomes increasingly difficult to keep track of their records manually.
- Therefore, a graphical user interface (GUI) for student database management can be a useful tool for schools, colleges, and universities.
- A student database GUI provides a user-friendly interface to store, retrieve, and manipulate student data efficiently.
- This system helps to automate many manual processes and provides a centralized platform to access student information quickly.
- A student database GUI can be developed using various programming languages and frameworks such as Java, Python, and C#.
In this article, we will discuss the implementation of a student database GUI using JAVA and Swing. Java is a popular programming language, while Swing is a user interface toolkit for creating graphical user interfaces (GUI) in Java applications.
Getting Started
To create a Student Database GUI,
- we will be using Java, which is a widely used programming language known for its simplicity and flexibility.
- For the graphical user interface, we will be using the Swing library in Java, which provides a set of user interface components for creating graphical user interfaces (GUIs).
- We will also be using the Java Database Connectivity (JDBC) API, specifically the java.sql library, to connect to the database.
- For our application, we will be using MySQL as our database and IntelliJ IDEA as our integrated development environment (IDE).
- Before we get started, make sure that you have Java and IntelliJ IDEA installed on your computer.
- You will also need to have MySQL installed and running on your system.
Once you have these requirements installed, you can proceed with creating the Student Database GUI.
Implementation
The implementation approach of this project involves creating a Java application that connects to a MySQL database and allows users to perform CRUD operations on a student data table.
-
CRUD - (create, read, update, delete)
-
The project consists of several classes, including the AppGUI class, which creates the user interface, and the dbConnect class, which connects to the MySQL database. The Table class is responsible for building a DefaultTableModel object from a result set returned by a SQL query, which is used to display data in the GUI.
-
The student data is stored in a MySQL database table called student_data, which has columns for Student_ID, first_name, last_name, major, Phone, GPA, and DOB. The dbConnect class is used to establish a connection to the database, and SQL queries are executed using a PreparedStatement object.
-
The Java application allows users to view, add, edit, and delete student data from the database table using the GUI. When a user performs an action, such as adding a new student, the appropriate SQL query is executed on the database table to reflect the change.
Overall, the implementation approach involved designing a GUI using Java Swing, connecting to a MySQL database using JDBC, and performing CRUD operations on a student data table using SQL queries.
Creating the GUI
As mentioned above, We will use IntelliJ IDE for our Project.
- Let's start with creating our project.
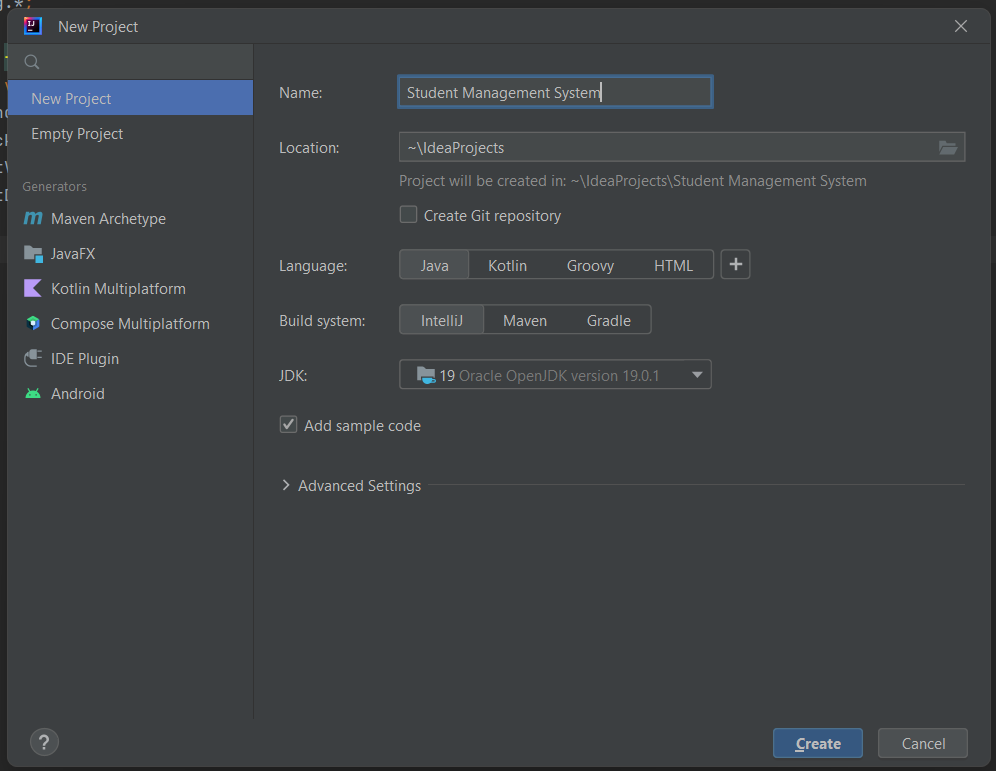
- create the required classes for our project by right clicking on src folder.
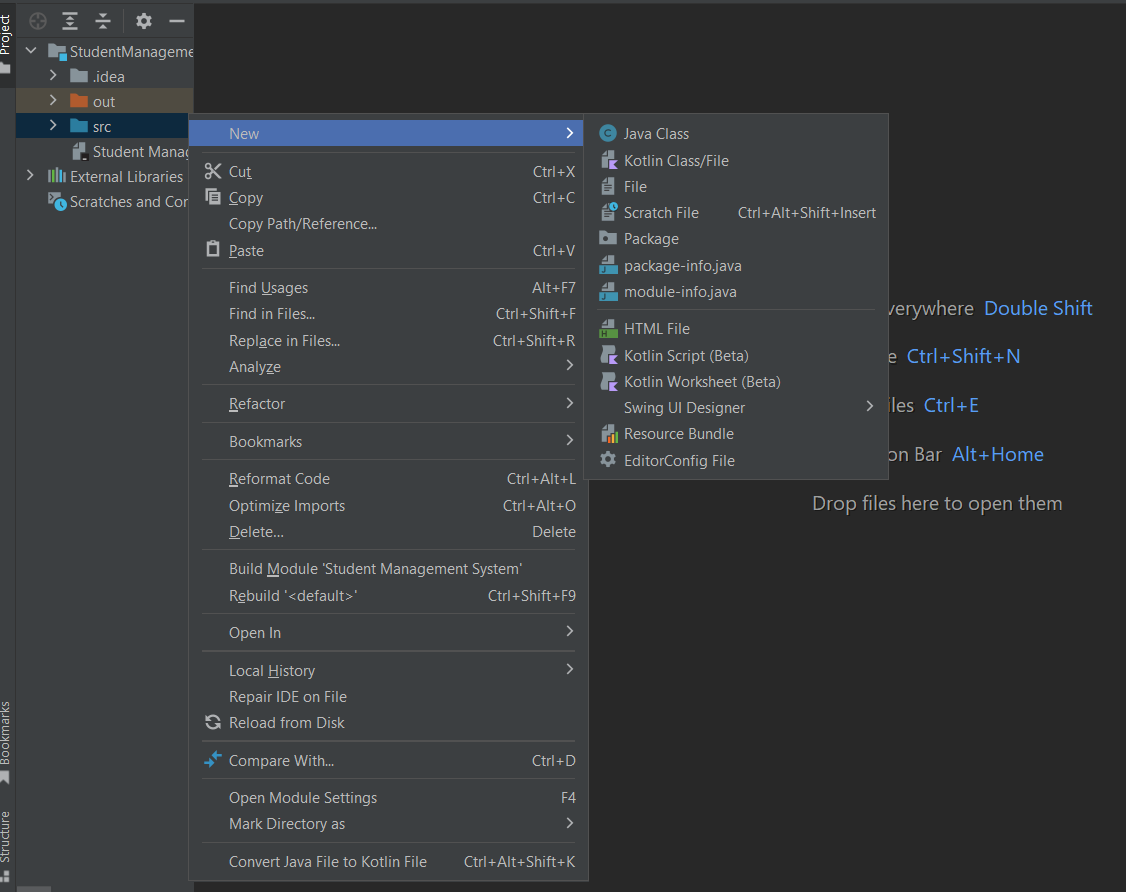
- AppGUI
- Table
- dBConnect
- Main
Now, let's code our project.
1. AppGUI class
import javax.swing.*;
import java.awt.event.*;
import java.sql.*;
public class AppGUI extends JFrame implements ActionListener {
private final JLabel studentIdLabel, firstNameLabel, lastNameLabel, majorLabel, phoneLabel, gpaLabel, dobLabel;
private final JTextField studentIdField, firstNameField, lastNameField, majorField, phoneField, gpaField, dobField;
private final JButton addButton, displayButton, sortButton, searchButton, modifyButton;
private Statement stmt;
public AppGUI() {
JFrame frame = new JFrame("Student Database");
JPanel panel = new JPanel();
// Initialize labels
studentIdLabel = new JLabel("Student ID:");
firstNameLabel = new JLabel("First Name:");
lastNameLabel = new JLabel("Last Name:");
majorLabel = new JLabel("Major:");
phoneLabel = new JLabel("Phone:");
gpaLabel = new JLabel("GPA:");
dobLabel = new JLabel("Date of Birth (yyyy-mm-dd):");
// Initialize text fields
studentIdField = new JTextField(10);
firstNameField = new JTextField(10);
lastNameField = new JTextField(10);
majorField = new JTextField(10);
phoneField = new JTextField(10);
gpaField = new JTextField(10);
dobField = new JTextField(10);
// Initialize buttons
addButton = new JButton("Add");
displayButton = new JButton("Display");
sortButton = new JButton("Sort");
searchButton = new JButton("Search");
modifyButton = new JButton("Modify");
// Add action listeners to buttons
addButton.addActionListener(this);
displayButton.addActionListener(this);
sortButton.addActionListener(this);
searchButton.addActionListener(this);
modifyButton.addActionListener(this);
// Add components to panel
panel.add(studentIdLabel);
panel.add(studentIdField);
panel.add(firstNameLabel);
panel.add(firstNameField);
panel.add(lastNameLabel);
panel.add(lastNameField);
panel.add(majorLabel);
panel.add(majorField);
panel.add(phoneLabel);
panel.add(phoneField);
panel.add(gpaLabel);
panel.add(gpaField);
panel.add(dobLabel);
panel.add(dobField);
panel.add(addButton);
panel.add(displayButton);
panel.add(sortButton);
panel.add(searchButton);
panel.add(modifyButton);
// Add panel to frame
frame.add(panel);
frame.pack();
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
dbConnect db = new dbConnect();
Connection conn;
try {
conn = db.getConnection();
} catch (SQLException | ClassNotFoundException ex) {
throw new RuntimeException(ex);
}
try {
stmt = conn.createStatement();
} catch (SQLException e1) {
e1.printStackTrace();
}
Table tb = new Table();
if (e.getSource() == addButton) {
// Insert new student into database
String sql = "INSERT INTO sdata VALUES('" + studentIdField.getText() + "', '"
+ firstNameField.getText() + "', '" + lastNameField.getText() + "', '" + majorField.getText()
+ "', '" + phoneField.getText() + "', '" + gpaField.getText() + "', '" + dobField.getText() + "')";
try {
stmt.executeUpdate(sql);
JOptionPane.showMessageDialog(null, "Student added successfully.");
} catch (SQLException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == displayButton) {
// Display all sdata in database
String sql = "SELECT * FROM sdata";
try {
ResultSet rs = stmt.executeQuery(sql);
// Create table to display student data
JTable table = new JTable(tb.buildTableModel(rs));
JOptionPane.showMessageDialog(null, new JScrollPane(table));
} catch (SQLException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == sortButton) {
// Sort sdata by selected column
String[] options = {"First Name", "Last Name", "Major"};
int choice = JOptionPane.showOptionDialog(null, "Sort by:", "Sort", JOptionPane.DEFAULT_OPTION,
JOptionPane.PLAIN_MESSAGE, null, options, options[0]);
String sql = "";
switch (choice) {
case 0 -> sql = "SELECT * FROM sdata ORDER BY first_name";
case 1 -> sql = "SELECT * FROM sdata ORDER BY last_name";
case 2 -> sql = "SELECT * FROM sdata ORDER BY major";
default -> {
}
}
try {
ResultSet rs = stmt.executeQuery(sql);
// Create table to display student data
JTable table = new JTable(tb.buildTableModel(rs));
JOptionPane.showMessageDialog(null, new JScrollPane(table));
} catch (SQLException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == searchButton) {
// Search for student by selected column
String[] options = {"Student ID", "Last Name", "Major"};
int choice = JOptionPane.showOptionDialog(null, "Search by:", "Search", JOptionPane.DEFAULT_OPTION,
JOptionPane.PLAIN_MESSAGE, null, options, options[0]);
String column = "";
switch (choice) {
case 0 -> column = "student_id";
case 1 -> column = "last_name";
case 2 -> column = "major";
default -> {
}
}
String searchTerm = JOptionPane.showInputDialog("Enter search term:");
String sql = "SELECT * FROM sdata WHERE " + column + " LIKE '%" + searchTerm + "%'";
try {
ResultSet rs = stmt.executeQuery(sql);
// Create table to display student data
JTable table = new JTable(tb.buildTableModel(rs));
JOptionPane.showMessageDialog(null, new JScrollPane(table));
} catch (SQLException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == modifyButton) {
// Modify selected student's data
String studentId = JOptionPane.showInputDialog("Enter student ID:");
String sql = "SELECT * FROM sdata WHERE student_id = '" + studentId + "'";
try {
ResultSet rs = stmt.executeQuery(sql);
if (rs.next()) {
String[] options = {"First Name", "Last Name", "Major", "Phone", "GPA", "Date of Birth"};
int choice = JOptionPane.showOptionDialog(null, "Select field to modify:", "Modify",
JOptionPane.DEFAULT_OPTION, JOptionPane.PLAIN_MESSAGE, null, options, options[0]);
String column = "";
switch (choice) {
case 0 -> column = "first_name";
case 1 -> column = "last_name";
case 2 -> column = "major";
case 3 -> column = "phone";
case 4 -> column = "gpa";
case 5 -> column = "date_of_birth";
default -> {
}
}
String newValue = JOptionPane.showInputDialog("Enter new value:");
sql = "UPDATE sdata SET " + column + " = '" + newValue + "' WHERE student_id = '" + studentId + "'";
stmt.executeUpdate(sql);
JOptionPane.showMessageDialog(null, "Student data updated successfully.");
} else {
JOptionPane.showMessageDialog(null, "Student not found.");
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
}
This code is a Java Swing application that allows a user to interact with a student database.
- The application provides a GUI that allows the user to add new students to the database, display all students in the database, sort students by various criteria, search for students by various criteria, and modify student information.
- The GUI consists of several JLabels and JTextFields for entering student information, as well as several JButtons for performing various actions.
- The application uses a MySQL database to store and retrieve student information, and uses the JDBC API to connect to the database and execute SQL queries.
- The actionPerformed() method is an implementation of the ActionListener interface that is used to handle button clicks.
- When a button is clicked, the actionPerformed() method checks which button was clicked and performs the appropriate action.
- For example, if the "Add" button is clicked, the actionPerformed() method retrieves the student information entered by the user, constructs an SQL INSERT statement to add the student to the database, and executes the statement using a Statement object.
- If the "Display" button is clicked, the actionPerformed() method constructs an SQL SELECT statement to retrieve all students from the database, executes the statement using a Statement object, and displays the resulting table in a JOptionPane.
Overall, this code provides a basic framework for building a Java Swing application that interacts with a database.
2. Table class
import java.sql.*;
import java.util.Vector;
import javax.swing.table.DefaultTableModel;
public class Table {
DefaultTableModel buildTableModel(ResultSet rs) throws SQLException {
ResultSetMetaData metaData = rs.getMetaData();
// Get column names
int columnCount = metaData.getColumnCount();
Vector<String> columnNames = new Vector<String>();
for (int i = 1; i <= columnCount; i++) {
columnNames.add(metaData.getColumnName(i));
}
// Get row data
Vector<Vector<Object>> rowData = new Vector<Vector<Object>>();
while (rs.next()) {
Vector<Object> row = new Vector<Object>();
for (int i = 1; i <= columnCount; i++) {
row.add(rs.getObject(i));
}
rowData.add(row);
}
return new DefaultTableModel(rowData, columnNames);
}
}
This Java code defines a class called Table that has a method called buildTableModel.
- This method takes a ResultSet object as input and returns a DefaultTableModel object.
- The ResultSet object represents a table of data retrieved from a database query.
- The ResultSetMetaData object contains metadata about the columns of the result set.
- The buildTableModel method first retrieves the column names from the metadata and stores them in a Vector object.
- It then iterates over each row of the result set, retrieves the data for each column, and stores it in a Vector object.
- These Vector objects are used to construct a new DefaultTableModel object, which is then returned by the method.
- The DefaultTableModel class is a standard Swing class used to represent data in a JTable.
- It extends the AbstractTableModel class and provides default implementations of the required methods.
- The DefaultTableModel constructor takes two arguments: a Vector of Vector objects representing the row data, and a Vector of String objects representing the column names.
3. Main class
import javax.swing.*;
public class Main {
public static void main(String[] args) {
AppGUI window = new AppGUI();
window.pack();
window.setVisible(true);
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
This is our entry program that creates a GUI window using the Swing framework.
Here's what this code does:
- Import the javax.swing package, which contains the classes for creating GUI components.
- Define a Main class with a main method.
- In the main method, create an instance of the AppGUI class, which is presumably a class that extends JFrame and contains the GUI components.
- Call the pack method on the window object, which sizes the window to fit its components.
- Call the setVisible method on the window object to display the window.
- Call the setDefaultCloseOperation method on the window object and pass the constant JFrame.EXIT_ON_CLOSE to specify that the application should exit when the window is closed.
This completes our Application design and I/O operations. Now, we need to make connection between database and application.For that, we still need to code dbConnect class and install drivers for mysql-java connector.
Setting up the Data Base
- To set up the connectivity, we should have MySQL Connector to the Java (JAR file).
- Go to this website Connector/J
- Then, select the Operating System platform-independent.
- Then, download the zip file Platform Independent (Architecture Independent), ZIP Archive.
- extract JAR file anywhere on your filesystem.
4. dbConnect class
import java.sql.*;
public class dbConnect {
private static Connection mycon=null;
public Connection getConnection() throws ClassNotFoundException, SQLException {
String db="studata"; // replace with your database name
String user = "root", pass = <your password comes here>;
String url = "jdbc:mysql://localhost:3306/"+db;
Class.forName("com.mysql.cj.jdbc.Driver");
Connection conn = DriverManager.getConnection(url,user,pass);
return conn;
}
}
- This code defines a Java class called "dbConnect" which includes a method "getConnection()" that returns a database connection to a MySQL database.
The database connection is established using the following information:
database name: "studata"
user name: "root"
password: "1236"
JDBC URL: "jdbc:mysql://localhost:3306/studata"
- The code first loads the MySQL JDBC driver by calling the static method "Class.forName()" with the name of the driver class as an argument.
- Then it establishes a connection to the database by calling "DriverManager.getConnection()" method with the JDBC URL, username, and password as arguments.
- The connection is returned to the caller of the "getConnection()" method. The connection can be used to execute SQL queries and updates on the database.
There is more one step remaining, that is registering our driver in IDE.
- To do this, we need navigate to File > Project Structure > Modules > Dependencies in IDE.
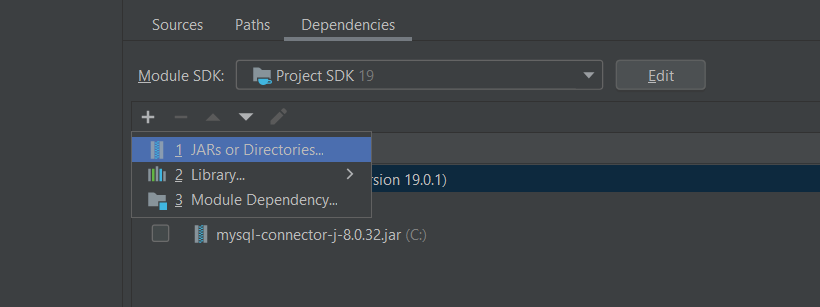
- Click "+" icon and select JARs or Dependencies
- filesystem will open and we have to select connector JAR file.
MySQL setup
- we need MySQL to be installed in our System.
- Go to this website, https://dev.mysql.com/downloads/ and download according to your OS.
- once installed, open command line or bash.
- login into your database by below command and enter your root password.
mysql -u root -p
- create our database and use it by following commands in mysql shell.
create database studentdata;
use studentdata;
- below code shows the example commands to create table and insert data
CREATE TABLE students (
Student_ID VARCHAR(10),
first_name VARCHAR(50),
last_name VARCHAR(50),
major VARCHAR(50),
Phone VARCHAR(15),
GPA DECIMAL(3,1),
DOB DATE
);
INSERT INTO students (Student_ID, first_name, last_name, major, Phone, GPA, DOB)
VALUES
('001', 'Sankar', 'Nagarapu', 'EEE', '9398555299', 7.0, '2002-06-24'),
('002', 'Dharani', 'Nagarapu', 'CSE', '9133401412', 9.0, '2004-06-05'),
('003', 'Sindhu', 'Makarapu', 'CSE', '9133505025', 10.0, '2004-06-25'),
('004', 'Sneha', 'kurma', 'CSE', '9548625756', 10.0, '2004-06-27'),
('005', 'meghana', NULL, 'CSE', '9548651652', 10.0, '2004-05-22');
- Now, Our Project Setup is complete.
Functionality
To Run the Application.
- run the Main.java in our IDE.
- the java app will be like this.(adjust the shape of your convenience)
- To view the database, click display button.
- We can add new student details by entering all textfields and clicking Add button.
- To view Sorted results, we can click on Sort button. It will prompts to ask by which factor to be sorted. Like this.
- It will show the results instantly.
- We can Search for an Student by his StudentId, lastname , major by clicking on search button.
- We can even modify student details with a studentId by clicking Modify button.
Further Improvements
Overall, this Project provides a basic framework for building a Java Swing application that interacts with a database. However, There are several features that can be added to the current student management system, such as fee tracking and attendance.
-
For example, it does not handle errors or exceptions very well, it does not sanitize user input to prevent SQL injection attacks, and it does not provide a way to delete student information. Additionally, the code could be refactored to improve modularity and reduce code duplication.
-
To implement fee tracking, we can create a new table in our database to store information about the fees paid by each student. This table could include columns such as student ID, date of payment, amount paid, and payment method. We could then add functionality to the application to allow administrators to view and update this information for each student.
-
Similarly, to implement attendance tracking, we can create another table in our database to store information about the attendance of each student. This table could include columns such as student ID, date of attendance, and whether the student was present or absent. We could then add functionality to the application to allow teachers to view and update this information for each student.
-
In terms of implementation approach, we would need to create new classes to handle the fee tracking and attendance functionality. These classes would likely be similar to the Student class we created earlier, but with additional methods to interact with the fee and attendance tables in the database.
-
In terms of database design, we would need to add two new tables to our database: one to store fee information and one to store attendance information. Both tables would need to be linked to the existing student table through the use of foreign keys.
Overall, the addition of fee and attendance tracking would greatly enhance the functionality of our student management system and allow administrators and teachers to more effectively track and manage student information.
Conclusion
In this article at OpenGenus,
- we have covered several topics related to databases and Java programming.
- We have learned about SQL, which is a standard language used to manage relational databases.
- We have also seen how to connect to a MySQL database using Java and how to retrieve data from a database and display it in a Java application.
- Additionally, we have covered the basics of Java programming, including classes, objects, methods, and variables.
Overall, understanding how to work with databases and Java programming is essential for developing robust and scalable applications that can manage and retrieve data efficiently.