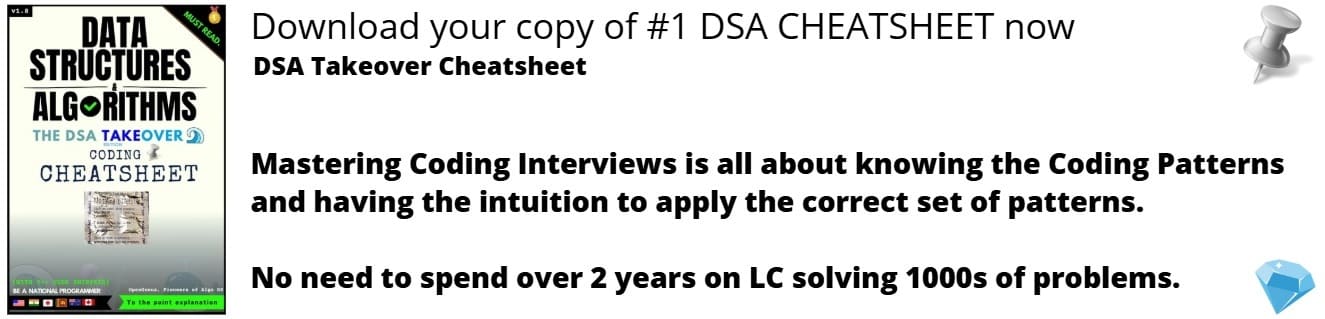
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to read and write to a text file in C programming language using multiple C code examples.
Table of contents:
- Header file needed
- Write to a text file
- Different modes
- Read from a text file
Header file needed
The header file stdio.h is needed in C to read and write to a text file.
The functions from stdio.h we will be using are:
- fopen: To open a file
- fprintf: To write to a file
- fgetc: To read a character from a file
- fclose: To close an opened file
- feof: To check end of file
This is same as reading and writing to console which in reality, interacts with stdio file (console).
Write to a text file
The steps to read a text file is as follows:
- Open the file in reading mode using fopen()
- Using fprintf, write anything in the file.
- Once done, close the file pointer using fclose.
Consider the following C code:
#include <stdio.h>
int main () {
FILE * fp;
fp = fopen ("file.txt", "w+");
fprintf(fp, "This is a sample line %d from OpenGenus", 1);
fclose(fp);
return(0);
}
Code: file-create.c
Compile and run the above code as:
gcc file-create.c
./a.out
This will create a text file named as "file.txt" with the following content:
This is a sample line 1 from OpenGenus
fopen creates the text file if it does not exist. The mode is w+ so it will override the contents of the file. If you want to add the text at the end of the existing file, use mode "a".
Different modes
The different modes of opening a file are:
- r: open a existing file for reading
- w: create a new file (with no content) for writing. If file exists, it will be overridden.
- a: appends text to a file. If the file does not exist, it will be created.
- r+: opens an existing file for both reading and writing.
- w+: creates a new file for both reading and writing.
- a+: opens an existing file for both reading and appending.
A mode is added as follows:
fp = fopen ("file.txt", "a+");
Create a text file named "text-content.txt" with the following content:
Line 1
Line 2 opengenus
Line 3
Now compile and run the following C code to append a line to the above file:
#include <stdio.h>
int main () {
FILE * fp;
fp = fopen ("file-content.txt", "a+");
fprintf(fp, "This is a sample line from OpenGenus");
fclose(fp);
return(0);
}
Code: file-append.c
The contents of the text file will be:
Line 1
Line 2 opengenus
Line 3
This is a sample line from OpenGenus
Read from a text file
The steps to read a text file is as follows:
- Open the file in reading mode using fopen()
- In a loop, read each character from the file one by one using fgetc and print it.
- If end of file is reached, then break the loop. This can be checked using feof().
Consider the following C code for reading a text file:
#include <stdio.h>
int main () {
FILE *fp;
int c;
fp = fopen("file-content.txt","r");
while(1) {
c = fgetc(fp);
if( feof(fp) ) {
break ;
}
printf("%c", c);
}
fclose(fp);
return(0);
}
Code: file-read.c
Output:
Line 1
Line 2 opengenus
Line 3
This is a sample line from OpenGenus
With this article at OpenGenus, you must have the complete idea of how to read and write to a text file in C.