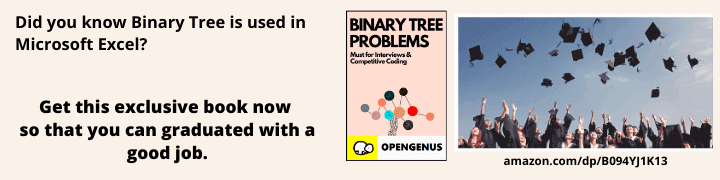
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
One of the core ideas of object-oriented programming (OOP), inheritance enables a new class to be built upon an existing class. In Java, inheritance is accomplished via extending a class, which allows the new class to take on the parent class's methods and properties. In this post at OpenGenus, we'll examine the fundamentals of Java inheritance and look at its benefits for OOP.
Learn more about OOP in Java.
Syntax and Types of Inheritance
In Java, the syntax for inheriting a class is as follows:
Here, the extends keyword is used to signify that the ChildClass will inherit the ParentClass's properties and methods. Java supports inheritance at several levels, allowing a class to derive from another class, which in turn may have descended from another class, and so forth. Java does not, however, support multiple inheritance, which allows a class to simultaneously inherit from numerous classes.
Java has five different kinds of inheritance:
- Single Inheritance: Only one parent class is extended by a child class.
- Multilevel Inheritance: One parent class extends another parent class, and so forth.
- Hierarchial Inheritance:Multiple child classes extend a single parent class through hierarchical inheritance.
- Multiple Inheritance:A child class extends multiple parent classes through multiple inheritance. (not supported in Java).
- Hybrid Inheritance: A synthesis of many hierarchical inheritance (not supported in Java).
Access Modifiers and Inheritance
Java access modifiers specify the level of access to a class's methods and attributes. The OOP access modifier guidelines are also followed by Java's inheritance system. A child class typically has access to all the protected and public properties and methods of its parent class. However, it is unable to use the parent class's private properties and methods.
The access modifiers of the parent class members are crucial when a child class inherits from a parent class. If a parent class member is marked as private, the child class cannot see it. If it is marked as protected, only classes that are members of the same package will be able to see it. if the parent class participant
is declared as public, then it is visible to the child class in any package.
Overriding Methods and Polymorphism
Java also supports the idea of method overriding, which lets a child class substitute its own implementation for a parent class-inherited method. When a child class wants to change the way a method from the parent class behaves, method overriding can be useful.
Using Java's @Override annotation, a child class can replace a parent class method. The parent class method's name, return type, and parameter list should all be used in the child class method. A child class method's actual override of a parent class method is confirmed by the @Override annotation.
Polymorphism, where the same method can have several implementations based on the class instance, is another result of method overriding in Java. When there is a need for polymorphism, same behavior needs to be implemented differently for different class instances.
The Advantages of Inheritance in OOP
In Java, inheritance has many benefits for OOP. Among them are:
- Code Reusability: By allowing the child class to inherit the parent class's methods and properties, inheritance helps to minimize the amount of duplicative code.
- Modularity: By allowing for the establishment of modular and hierarchical class structures, inheritance improves the organization and maintainability of the code.
- Polymorphism:Inheritance enables polymorphism, or the ability for the same method to have different implementations depending on the class instance. This enables software design to be more flexible and adaptable.
- Abstraction:A greater level of abstraction is provided by abstract classes and interfaces, which can be created through inheritance and keep the implementation details hidden from the outside world.
Best Practices for Inheritance in Java
Java's inheritance capability is strong, but it should only be used sparingly. The following are some recommended methods for leveraging inheritance in Java:
- When it makes sense, only use inheritance. The relationship between the child and parent classes should be established through inheritance. It is not advisable to use inheritance if there is no obvious tie.
- Steer clear of complex inheritance hierarchies. The code may become complex and challenging to comprehend due to deep inheritance hierarchies. Inheritance hierarchies ought to be no deeper than three to four layers.
- To describe the shared behavior of a collection of classes, use abstract classes or interfaces. The behavior of the implementing classes is clearly defined through abstract classes and interfaces, which improves the readability and maintainability of the code.
- Utilize the term "final" to stop further subclassing of a class. The "final" keyword can be used to prevent the creation of further subclasses of a class. This can be useful when the class implementation is complete and should not be modified.
- Prefer composition over inheritance. Composition is an alternative to inheritance, where a class can contain objects of other classes to achieve the desired behavior. Composition can be more flexible and modular than inheritance in some cases.
Finally, I would like to point out that inheritance is a strong feature of Java that allows for code reuse, modularity, polymorphism, and encapsulation. To prevent concerns with code complexity and maintainability, it should, however, be used sparingly and in accordance with best practices. Java developers may write elegant, flexible code that adapts to the changing requirements of their applications by carefully using inheritance.