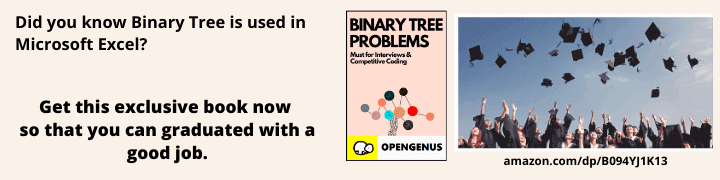
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 45 minutes
Classes in Java are structures that allow programmers to define rules to handle data in a custom way and objects are the actual classes in execution with data. A class is defined by the data it holds and the functions it provides to handle its data. In short, classes can be seen as user defined data types.
In this article, you will understand:
- Why we need classes?
- How to define and use classes in Java?
- Structure of classes in Java along with constructors and access specifiers
- How to use objects in Java?
- Characteristics and attributes of objects along with comparing two objects
Before going to classes, we will take a brief review of primitive data types and the need for classes
Why we need for classes? and Primitive data types
Primitive data types are the built in data types that are natively supported by the programming language. There are eight primitive data types in Java. They are:
- byte
- short
- int
- long
- float
- double
- char
- boolean
The issue is that in this case, we do not have a data type to represent a particular thing say cat. A cat can be defined by data such as weight, color, age and others while it has behaviour like eating preferences. To represent a cat in a program, we can simply use multiple integers and functions with arrays to work around but the problem is that this approach:
- is not scalable when we need dynamic number of instances (cats)
- difficult to manage as the data is not self explanatory
To tackle this, we can create custom data types using classes. Infact, classes are just a sophisticated structure with the primitive data types at the lowest level.
Data types that can be created using the primitive data types are known as user defined data types. Since they are created by the user they are known as user defined data types or composite data types.
Hence, we need classes for:
- better code design and management
- code scalability
What are Classes in Java?
Class can be defined as a structure for a group of objects having the same properties. A class in Java has two properties/ fields:
- data members: variables to store data
- member functions: functions that denote a class functionality
Syntax for creating a class:
class ClassName
{
// data members
<user-defined or primitive data type> variable name;
//member functions
<return type> function(function paramters)
{
... // to handle data members
}
}
Here class is a keyword used to declare a class and className can be anything but it should start with uppercase letter.
As said above ,a user defined data type is one which can be created by the primitive data type. A class is also a user defined data type. Consider this example
class Demo // a class named Demo
{ // start of class
int a; // primitive data type int
float b; // primitive data type float
public void getData() // method getData
{
....
}
public void display() // method display
{
....
}
} // end of class
A class has some data members and member functions.The data members are the variables and the member functions are the block of statements used to perform a specific task.
Data members represent the state of the class and they are of two types:
-
Class variable (static variables): It is the data member that is declared once for a class. All objects of the class share these data members as there is a single copy of them available in memory.
-
Instance variable: It is the data member that is created for every object of the class.For example there are ten objects, then there would be ten copies of the instance variable, one copy for each object.
consider the following code
class Sample
{
int anInt; // instance variable
float aFloat; // instance variable
static float anotherFloat; // static variable
}
What are Member functions in a class?
Member functions are the block of statements used to perform a specific task. Syntax for creating a method:
access specifier returntype methodName()
{
...
}
Consider the following example
public void display() //method
{
System.out.println("Hello world!");
}
Here, public is access specifier, void is the return type and display is the method name.
They are also of two types: Instance methods and class methods.
-
Instance methods: They operate on the current object's instance variables but also have access to the class variables.
-
Class methods: They are also known as static methods.They cannot access the instance variables declared within the class.They can be invoked without any instance.
Access Specifiers
Access or visibility rules determine whether a method or a data variable can be accessed by another method in another class or subclass.
There are four access specifier:
-
Default: It will be accessible within the same folder.(Note that default is not a keyword, in the absence of any other access specifier, default access takes place.)
-
public: It will be accessible everywhere.
-
private: It will be accessible in the same class.
-
protected: It will be accessible within parent and child class only.
What are Objects in Java?
Object is an identifiable entity with some characterstics and behaviour. We can see it as a class in execution with real data on which we can use member functions.
For example, Orange is an object for Fruit class, its characterstics are- it is spherical in shape and its colour is orange and its behaviour is - it is juicy and tastes sweet-sour.
Syntax to create an object of a class:
ClassName objectName = new constructor();
Example:
Demo d1 = new Demo();
Here d1 is the object of class Demo. And, constructor has the same name as the class name.
With the help of object we can access the functions of the class. Example:
class Demo //class Demo
{ //start of class
public void add(int a) //method add
{
a=a+10;
System.out.println("The new value is "+a);
}
public static void main(String args[]) //main method
{
Demo d1=new Demo(); //object d1 of class Demo
d1.add(10); //calling method using object d1 of class Demo
}
}//end of class
What are Constructors in Java?
A member function with the same name as its class name is called a constructor and it is used to initialize the objects of that class with legal initial values.
The main purpose of constructor is to create an instance of a class.
Example:
Student s1 = new Student();
Here Student after the new keyword is the constructor. the new keyword is used to allocate the memory to the object.
There are two types of constructors:
- Parameterized constructors: It is the constructor that takes arguments.
Consider the following example:
class Example // class declaration
{
int i; //instance variable
float j; //instance variable
Example(int a,float b) // parameterized constructor
{
i=a;
j=b;
}
....
} //end of class
Now while creating the objects of class Example we need to pass one int value and one float value.
Example e1 = new Example(2,2.5);
- Non parameterized constructor: It is the constructor that does not take any arguments.It is also called the default constructor. Example:
Student s1=new Student();
Here Student after the new keyword is the constructor.
Properties of constructor:
- It has the same name as its class.
- It has no return type not even void.
- It creates an instance of the class.
- It is called at the time of object creation.
How to compare objects in Java?
== tests for reference equality (whether they are the same object):
.equals() tests for value equality (whether they are logically "equal")
equals() is a method used to compare two objects for equality. The default implementation of the equals() method in the Object class returns true if and only if both references are pointing to the same instance. It therefore behaves the same as comparison by ==.
public class Foo
{
int field1, field2;
String field3;
public Foo(int i, int j, String k)
{
field1 = i;
field2 = j;
field3 = k;
}
public static void main(String[] args)
{
Foo foo1 = new Foo(0, 0, "bar");
Foo foo2 = new Foo(0, 0, "bar");
System.out.println(foo1.equals(foo2)); // prints false
}
}
Even though foo1 and foo2 are created with the same fields, they are pointing to two different objects in memory.Therefore it evaluates to false because whenever new keyword is used, memory is allocated for an object, so two objects are created since they have different memory allocated, the result is false.
What are the characterstics of Objects?
- An object is an instance of the class.
- An object has four key features:attributes,behaviour,state and identity.
- The connstructor instantiates the objects.
- Objects are used to access method defined in the class.
What are the Key features of an object?
-
Attributes: These are the properties that characterise an object.Example- a human being type object has attributes such as name, age, height, weight etc.
-
Behaviour: These are the functionalities associated with an object.Example- a human being type object has behaviour such as reading, writing, speaking, sleeping etc.
-
Identity: The unique way of refering to an object.Example- a human being type object's name is Ben,then to uniquely identify him we will use his identity Ben.
-
State: It depicts how the object has reacted to the methods.Example- A new born baby , is a human being type object,when grows to a toddler remains the same human being but his height and weight have changed. So it remains the same human being type object, but his height and weight have changed. So the states of an object change.