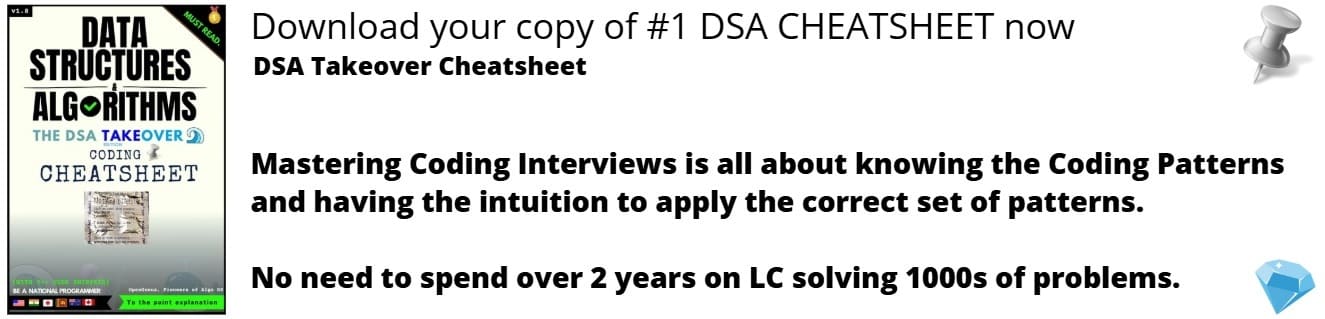
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will learn combination of information related to C programming language, terminal commands, common data types, operators, conditionals (if, if-else, else if), and loops (while, do-while, for). It covers basic concepts and provides examples for each topic.
Table of content
- Header file
- CLI (command line Interface)
- Datatypes
- Operators
- conditional
- If
- If and else
- else if
- nested if
- Loops
- while loop
- do while loop
- for loop
Header file
In the C Programming Language, the #include directive tells the preprocessor to insert the contents of another file into the source coIn the C Programming Language, the #include directive tells the preprocessor to insert the contents of another file into the source code
include <cs50.h>
include <stdio.h>
- Files of C is saved with .c notation at the end of a file name.
hello.c
some of the common terminal command lines for basic use .
- cd (change directory)
- rm (remove file)
- rmdir (remove directory)
- touch ( make new file) for linux user
- mv (to move or rename file)
- make "file name" to compile a file
- ./file_name to run a file
Some common datatypes
- int uses 4 bytes to store a data i.e. 32 bits. 1 byte = 8bits. 32 bits can store 4.2 billion signs (0 or 1). 2^16 negative integer and 2^16-1 positive integer. 1 bit is used for 0.
- unsigned int also uses 4 bytes data, it eliminates negative integer as a result you can use all the storage for positive integer. This is used when the input will not be a negative integer.
- bool uses 1 byte and stores either true or false value.
- float uses 4 bytes. It stores decimal integers and works similar as integer. but when the decimal values goes over the storage limit it breaks the data and shows what was calculated. you will notice it when finding the values of pi.
- double uses 8 bytes. It is just a modified version of float. As it uses 64 bits the storage capacity is exponentially high. for reference it is around 18 million billion.
- long is modified storage version of int. it uses 64 bits.
- char uses 1 byte as it stores only one character. like 'A', 'a', ‘1’, ‘@’ etc
Operators
- In mathematics '=' sign is used to signify a variable is equals to a value/real number. but in programming world '=' is used to assign value to the variable on left side of the sign, from whatever the value of right is. it will simply assign the value to the variable.
- '==' this is the operand that does what maths = does.
- '<' '>' '<=' '>=' these operands does exactly what you have been studying in elementary or middle school.
- "&&" this operation checks if both given conditions are true or not. If both conditions turns out to be true it will execute the programme.
- || this operator checks if any one condition is true then print the result.
if x is true and y is true = true
if x is true and y is false = false
if x is false and y is true = false
if x is false and y is false = false
if x is true and y is true = true
if x is true and y is false = true
if x is false and y is true = true
if x is false and y is false = false
int a; //Declaration
a = 2;
it can be written as int a = 2; // Initialization.
X = X + Y; what does this statement means to you? It takes the value of X and then add it to Y and stores the value in X.
It can be written as X += Y
This is same as above statement but a little cleaner and little less time consuming.
Conditionals
If Statement checks if the boolean expression is true and then executes the statement.
if (boolean expression){
}
code example
if (num > 5) {
printf("The number is greater than 5.\n");
}
If Else Statement checks if the boolean expression is true or false and then executes the statement respectively.
when a boolean expression is true it prints the if statement else statement if it is false.
if (boolean expression){
}
else {
}
code example
if (num > 5) {
printf("The number is greater than 5.\n");
} else {
printf("The number is less than or equal to 5.\n");
}
else if statement checks for multiple boolean expression and executed the program when expression is true or false respectively.
if (boolean expression){
}
else if (boolean expression){
}
else if (boolean expression){
}
else {
}
code example
if (num > 10) {
printf("The number is greater than 10.\n");
} else if (num > 5) {
printf("The number is greater than 5 but not greater than 10.\n");
} else if (num > 0) {
printf("The number is greater than 0 but not greater than 5.\n");
} else {
printf("The number is less than or equal to 0.\n");
}
Nested if statement uses multiple if statement. It is very similar to ‘else if’ but in ‘else if ‘where it check for true statement and runs false statement once. In nested if you can use else statement for every if.
if (boolean expression){
}
else{
}
if (boolean expression){
}
else{
}
if (boolean expression){
}
else{
}
Loops
while Loop is used when you don't know the number of times the loop needs to run. it runs the statement again and again until the boolean expression is wrong.
it can be used in games, like when you don't know till when a player will quit, and you need to run the environment or gameplay, Or other background activities.
int a = 10;
int b = 5;
while (a > b) {
printf("Hello\n");
a--;
}
do while Loop only difference is do while loop runs the programme once and then check the boolean expression and then program accordingly . It is used when you need to run once and the loop.
do{
printf(hello);
}
while(a>b);
int count = 0;
do {
printf("Count: %d\n", count);
count++;
} while (count < 5);
For loop for loop is used when you have to run a loop to a certain number of time.
don't get intimidated by all the syntax, read step by step. firstly ’i’ is initialized and then a boolean expression is given to i and lastly increment/decrement the value by 1.
for(int i = 0; i<=b; i++){
printf(hello);
}
int b = 5;
for (int i = 0; i <= b; i++) {
printf("Hello\n");
}
return 0;
With this article at OpenGenus, you must have a strong introduction to C Programming.