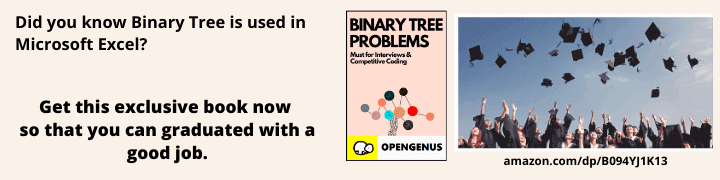
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will explore how to generate a fibonacci sequence and implement a program in C Programming Language to demonstrate the concept.
TABLE OF CONTENT
- QUESTION
- APPROACH TO SOLUTION
- IMPLEMENTATION
- OUTPUT
QUESTION
Here we are going find the first n numbers of a Fibonacci sequence by using C pogramme.
Where"n" is the number of terms by using for loop.
APPROACH TO SOLUTION
- Fibonacci sequence :The fibonacci sequence starts from 0 then 1 and then addittion of the precceding number and goes on till infinite or the number of term specified by the user
For example:
The number of term (n)=10
Then
fibonacci sequence= 0,1,1,2,3,5,8,13,21,34
- Here we are going to use FOR LOOP
The for loop is a entery controlled loop(the loop test the condition first then it gets executed). The loop provides more concise loop control structre.
THE STRUCTRE OF FOR LOOP IS:
for(intialization;test-condition;increment/decrement)
{
body loop
}
- Here the intilization stands for the loop controlling intials such as "i" or "count"
- Here the test condition defines when the loop will exit
such as:
i>10
*When the loop ends for the first time then it incrment or decrment according to the information provided by the coder such as "i++ "or "i--"
STEPS FOR THE FORMATION OF THE CODE:
1.Library and function
To enable the program to take user input, we need to use stdio.h library in C. stdio stands for "Standard Input and Output". It is used within the main function ("int main()") and is included as follows:
#include <stdio.h>
2.Declaration of data type
To specify or inform the computer about what type of data type we are going to use we will use data type. In this type of question, we will use:
integer(int):for integer type numbers
- INTRODUCTION TO VARIABELS
Here we are using
n= number of terms
i=intializer
f=fibonacci sequence
f1=0
f2=0
4.The User Input
The number of terms is user input.
printf("enter the numbers of terms:");
scanf("%d",&n);
printf("\nThe fibbonacci series generated is :\n");
printf("%d %d ",f1,f2);
5.THE BEGNNING OF FOR LOOP
After user input we will start the for loop
so according to for loop we have to use a intializer which is "i" such that i=1
then we have to set a test condition here since the number of terms is user input (n)
So, i<=n-2
Then since the loop should continue adding then we will use increment here. In the body, we will use the fibbonacci sequence formation such that:
// Add previous two terms
f = f1 + f2;
printf("%d ",f);
// Update / Shift the previous two terms
f1=f2;
f2=f;
At last, we will end the code by return 0.
0 denotes that the program has executed successfully.
Implementation in C
Following is the complete C program:
// Part of iq.opengenus.org
#include<stdio.h>
int main()
{
// Initialize first 2 terms as 0 and 1
// First two terms f1 and f2
int n,i,f1=0,f2=1,f;
printf("\nEnter the number of terms :");
scanf("%d",&n);
printf("\nThe fibbonacci series generated is :\n");
printf("%d %d ",f1,f2);
for(i=1;i<=n-2;i++)
{
// Add previous two terms
f=f1+f2;
printf("%d ",f);
// Update / Shift the previous two terms
f1=f2;
f2=f;
}
return 0;
}
OUTPUT
Output:
Enter the number of terms :20
The fibbonacci series generated is :
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987 1597 2584 4181
With this article at OpenGenus, you must have the complete idea of how to implement a C program to generate a list of fibonacci numbers.