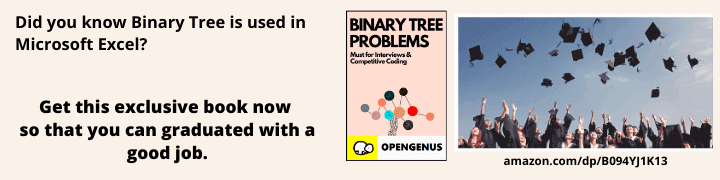
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of contents:
- What is An Exception
- Steps Involved in Creating Custom Exceptions
- Reasons For Using Custom Exceptions
- Benefits Of Creating Custom Exceptions
What is an Exception?
A runtime error that arises during the execution of a program is referred to as an exception. There are two types of exceptions:
- Built-in Exceptions
- Custom Exceptions
In this article at OpenGenus, custom exceptions will be discussed.
The term "custom exception" or "user-defined exception" refers to the creation of our own exceptions in Java, which are derived classes of the Exception class. In general, Java custom exceptions are used to tailor the exception to user needs.
Steps Involved in Creating Custom Exceptions
These steps will be explained using the example of a voting system where the program throws "Invalid Age" if the person's entered age is less than 18 years.
Step 1: Create a subclass of the Exception class
class InvalidAgeException extends Exception{
}
Step 2: Create a Constructor from the custom Exception class(InvalidAge) with String as a parameter and then call the constructor from Exception class using the super() keyword.
class InvalidAgeException extends Exception{
public InvalidAgeException(String message){
// calling the constructor of parent Exception
super(message);
}
}
}
Note: The Exception class can be called without the use of parameters and calling super() is not necessary.
Step 3: Create an object of the custom exception class and throw it using the throw clause
import java.util.Scanner;
class InvalidAgeException extends Exception{
public InvalidAgeException(String message){
super(message);
}
}
class Main{
private static int age;
//method to check user input
static void validate() throws InvalidAgeException{
Scanner scanner = new Scanner(System.in);
System.out.println("Enter your Age");
age = scanner.nextInt();
if(age<18){
// throw an object of user defined exception
throw new InvalidAgeException("You are not eligible to vote");
}
else{
System.out.println("You are eligible to vote");
}
}
//main method
public static void main(String[]args){
try
{
//calling the method
validate();
}
catch(InvalidAgeException exception){
System.out.println("Caught an Exception: "+exception);
}
}
}
Output
Enter your Age
25
You are eligible to vote
Enter your Age
13
Caught an Exception: InvalidAgeException: You are not eligible to vote
Reasons For Using Custom Exceptions
In software development, exceptions are used to manage and communicate any faults or unforeseen circumstances that may arise. While most programming languages come with a collection of built-in exceptions that may be used in a variety of situations, it may occasionally be essential to develop your own exceptions that more accurately reflect the particular error or issue that is occurring in the code.
When creating complex software systems or libraries, custom exceptions come in particularly handy because they allow for a more precise error handling approach. Developers can give a concise error message that expresses the precise nature of the issue and its placement in the code by generating custom exceptions. This can help other developers who might use the code or library to find and address the problem.
Additionally, Custom exceptions can also aid in making the codebase easier to manage. Developers can make it easier to understand the meaning of the code and the intended behavior of the code by developing a set of custom exceptions that are particular to the domain or functionality of the code. As a result, developers can create test cases that particularly target the custom exceptions, verifying that the code handles them appropriately. This can also aid in testing and debugging.
Benefits Of Creating Custom Exceptions
- Improved Exception Handling: When developing the business logic, developers can use custom exceptions to help them decide which exceptions to throw in accordance with the business requirements. Therefore, if done consistently, it guarantees proper exception handling without problems.
- Improved Code Usability: Custom exceptions, like the one in the aforementioned example, greatly aid in the understanding and readability of code. It matches the way that code is organized when class and method names are related to functionality and also to exception handling. Additionally, this makes it easier for developers to relate exceptions to business needs rather than just those that are connected to them.
- Ease in Debugging: Custom exceptions can give more specific information about the error that occurred, such as the precise line of code where it occurred, the values of variables at the moment of the error, and other pertinent information. This makes it easier for developers to find a solution to the problem.
- Customized Responses: Custom exceptions provide you the option to modify how your application responds. You can specify how your application should respond to particular failures by generating custom exceptions, such as by showing the user a custom error message or redirecting them to a particular page. This could improve your application's user experience overall.
In conclusion of this article at OpenGenus, Java programming's use of custom exceptions significantly improves the clarity, maintainability, and reliability of software systems. Developers can provide a more exact and descriptive error handling mechanism that can make it easier to discover and fix issues by generating custom exceptions that precisely depict the unique faults or problems that may occur in the code.