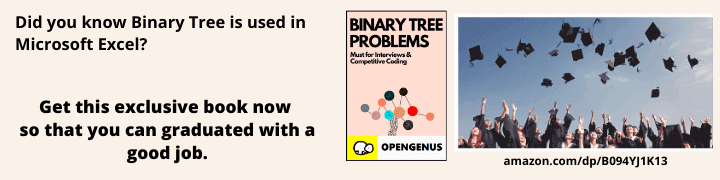
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the string.h library in C Programming Language along with the 22 member functions available in it. We have presented the ideas with complete C code examples.
Table of contents:
- Introduction to
<string.h>
library - Table: Functions in the <string.h> library
- Function description and usage Examples
Introduction to <string.h> library
The string.h library is a vital tool in C programming, providing functions for efficient string manipulation. It offers a rich set of functions for common operations like copying, concatenating, comparing, and finding the length of strings. These functionalities make it convenient for tasks involving string manipulation. Additionally, the string.h library is commonly used for input and output processing, allowing programmers to easily handle string inputs and outputs. It is also useful in text processing tasks like parsing, searching, replacing, and tokenizing strings, commonly used in applications like text editors and data processing programs. Furthermore, the string.h library provides functions for dynamic memory management, making it useful in scenarios where efficient memory allocation and deallocation for strings are required. Overall, the string.h library is a powerful tool for C programmers, offering a comprehensive set of functions for efficient string operations in various use cases.
Default Key Values
- macros value: NULL (value of null pointer)
- Default variable: size_t (type: unsigned int) - This variable is used to store a reccurent value access of keyword sizeof.
Table: Functions in the <string.h> library
S.no. | Function | Definition |
---|---|---|
1 | memchr() | The function looks for the first occurrence of the character c (an unsigned char) in the first n bytes of the string specified by the argument str. |
2 | memcmp() | Compares the first n bytes of str1 and str2. |
3 | memcpy() | Copies n characters from src to dest. |
4 | memmove() | Alternative function to copy n characters from str2 to str1. |
5 | memset() | Copies the character c (an unsigned char) to the first n characters of the string pointed to, by the argument str. |
6 | strcat() | Appends the string pointed to, by src to the end of the string pointed to by dest. |
7 | strncat() | Appends the string pointed to, by src to the end of the string pointed to, by dest up to n characters long. |
8 | strchr() | The function looks for the first occurrence of the character c (an unsigned char) in the string. |
9 | strcmp() | Compares the string pointed to, by str1 to the string pointed to by str2. |
10 | strncmp() | Compares at most the first n bytes of str1 and str2. |
11 | strcoll() | Compares string str1 to str2. The result is dependent on the LC_COLLATE setting of the location. |
12 | strcpy() | Copies the string pointed to, by src to dest |
13 | strncpy() | Copies up to n characters from the string pointed to, by src to dest. |
14 | strcspn() | Calculates the length of the initial segment of str1 which consists entirely of characters not in str2. |
15 | strerror() | Searches an internal array for the error number errnum and returns a pointer to an error message string. |
16 | strlen() | Computes the length of the string str up to but not including the terminating null character. |
17 | strpbrk() | Finds the first character in the string str1 that matches any character specified in str2. |
18 | strrchr() | Searches for the last occurrence of the character c (an unsigned char) in the string pointed to by the argument str. |
19 | strspn() | Calculates the length of the initial segment of str1 which consists entirely of characters in str2. |
20 | strstr() | Finds the first occurrence of the entire string needle (not including the terminating null character) which appears in the string haystack. |
21 | strtok() | Breaks string str into a series of tokens separated by delim. |
22 | strxfrm() | Transforms the first n characters of the string src into current locale and places them in the string dest. |
Function description and usage Examples
These are the implementation of each of the listed function with an example of each:
1. memchr()
The memchr()
function looks for the first occurrence of the character c in the first n bytes of the string specified by the argument str.
Return type: void
Arguments of the function: string, target character, sizeof string as size_t
Code snippet: void *memchr(const void *str, int c, size_t n)
Example Code
#include <stdio.h>
#include <string.h>
int main() {
// Input string
char str[] = "Hello, world!";
// Character to search for
char ch = 'w';
// Finding the first occurrence of the character 'ch' in the input string
char *result = (char *)memchr(str, ch, strlen(str));
if (result != NULL) {
printf("'%c' found at position %ld in the string.\n", ch, result - str + 1);
} else {
printf("'%c' not found in the string.\n", ch);
}
return 0;
}
Output:
'w' found at position 8 in the string.
Explanation:
In this example, we have a string str which contains the text "Hello, world!" and we want to find the first occurrence of the character 'w' in the string. We use memchr() function which takes three arguments: a pointer to the memory block to search, the character to search for, and the number of bytes to search within the memory block (which we obtain using strlen() function). The function returns a pointer to the first occurrence of the character in the memory block, or NULL if the character is not found. In our example, we check the result returned by memchr() and print the position of the character in the string if it is found, otherwise, we print a message indicating that the character is not found in the string.
At the output we get 8. This is because the character 'w' is found at the 8th position (0-indexed) in the input string "Hello, world!". The result pointer points to the memory location where the character 'w' is found in the string, and we calculate the position of the character by subtracting the starting address of the string (str) from the address of the found character (result), and adding 1 to account for the 0-indexing in C.
2. memcmp()
Return type: void
Arguments of the function: string, target character, sizeof string as size_t
Code snippet: void *memchr(const void *str, int c, size_t n)
Example Code
#include <stdio.h>
#include <string.h>
int main() {
// Input strings
char str1[] = "Hello";
char str2[] = "Helly";
// Comparing the two strings
int result = memcmp(str1, str2, strlen(str1));
if (result == 0) {
printf("The two strings are identical.\n");
} else if (result < 0) {
printf("The first string is lexicographically smaller.\n");
} else {
printf("The first string is lexicographically larger.\n");
}
return 0;
}
Output:
The first string is lexicographically smaller.
Explanation:
In this example, we have two strings str1 and str2 which contain the texts "Hello" and "Helly" respectively. We want to compare the two strings lexicographically, i.e., based on their ASCII values. We use the memcmp() function which takes three arguments: pointers to the memory blocks to compare, and the number of bytes to compare within the memory blocks (which we obtain using strlen() function). The function returns an integer value that indicates the result of the comparison:
- If the returned value is 0, it means the two memory blocks are identical.
- If the returned value is less than 0, it means the first memory block is lexicographically smaller.
- If the returned value is greater than 0, it means the first memory block is lexicographically larger.
In our example, we check the value returned by memcmp() and print the comparison result accordingly.
The output of the code example would depend on the values of str1 and str2 at runtime, as well as the implementation of the memcmp() function in the specific environment where the code is compiled and executed. However, based on the example code provided, here are some possible outputs:
-
If str1 is "Hello" and str2 is "Helly":
output:The first string is lexicographically smaller.
-
If str1 is "Helly" and str2 is "Hello":
output:The first string is lexicographically larger.
-
If str1 is "Hello" and str2 is "Hello":
output:The two strings are identical.
3. memcpy()
The function memcpy()
is used to copy a block of memory from one location to another. It takes three arguments: a pointer to the destination memory block, a pointer to the source memory block, and the number of bytes to copy.
Return type: void
Arguments of the function: destination memory block, source memory block, number of bytes to copy as size_t
Code snippet: void *memcpy(void *dest, const void *src, size_t n)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Source string
char src[] = "Hello, world!";
// Destination string
char dest[20];
// Copying the source string to the destination string
memcpy(dest, src, strlen(src) + 1);
printf("Source string: %s\n", src);
printf("Copied string: %s\n", dest);
return 0;
}
Output:
Source string: Hello, world!
Copied string: Hello, world!
Explanation:
In this example, we have a source string src which contains the text "Hello, world!" and a destination string dest which is an empty character array with a size of 20 bytes. We want to copy the contents of the source string to the destination string using the memcpy() function.
The memcpy() function takes three arguments: a pointer to the destination memory block (dest), a pointer to the source memory block (src), and the number of bytes to copy (strlen(src) + 1 to include the null terminator). The function copies the specified number of bytes from the source memory block to the destination memory block.
In our example, we use memcpy() to copy the contents of the source string src to the destination string dest. After copying, both the source and destination strings have the same contents, as seen in the output.
It's important to note that memcpy() does not perform any null-termination automatically, so if the source string is not null-terminated, the destination string may not be null-terminated as well. Therefore, it's necessary to ensure that the source string is null-terminated or to manually add a null terminator to the destination string after using memcpy().
4. memmove()
The function memmove()
is used to copy a block of memory from one location to another, even if the source and destination memory blocks overlap. It takes three arguments: a pointer to the destination memory block, a pointer to the source memory block, and the number of bytes to copy.
Return type: void
Arguments of the function: destination memory block, source memory block, number of bytes to copy as size_t
Code snippet: void *memmove(void *dest, const void *src, size_t n)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Source string
char src[] = "Hello, world!";
// Destination string
char dest[20];
// Copying the source string to the destination string with overlapping memory blocks
memmove(dest, src, strlen(src) + 1);
printf("Source string: %s\n", src);
printf("Copied string: %s\n", dest);
return 0;
}
Output:
Source string: Hello, world!
Copied string: Hello, world!
Explanation:
In this example, we have a source string src which contains the text "Hello, world!" and a destination string dest which is an empty character array with a size of 20 bytes. We want to copy the contents of the source string to the destination string using the memmove() function.
The memmove() function is similar to memcpy() in that it copies the specified number of bytes from the source memory block to the destination memory block. However, memmove() is designed to handle overlapping memory blocks, whereas memcpy() may lead to undefined behavior if the source and destination memory blocks overlap.
In our example, we use memmove() to copy the contents of the source string src to the destination string dest, even though they may overlap. The strlen(src) + 1 argument is used to specify the number of bytes to copy, including the null terminator. After copying, both the source and destination strings have the same contents, as seen in the output.
It's important to note that memmove() may be slightly slower than memcpy() due to the additional checks for overlapping memory blocks. Therefore, it's recommended to use memcpy() when overlapping memory blocks are not expected, and use memmove() when overlapping memory blocks may occur to ensure correct behavior.
5. memset()
The function memset()
is used to set a block of memory to a specified value. It takes three arguments: a pointer to the memory block to be filled, the value to be set, and the number of bytes to be set.
Return type: void
Arguments of the function: memory block, value to be set, number of bytes to be set as size_t
Code snippet:
void *memset(void *ptr, int value, size_t n)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Destination buffer
char buffer[10];
// Fill the buffer with 'A' character
memset(buffer, 'A', sizeof(buffer));
printf("Buffer: %s\n", buffer);
return 0;
}
Output:
Buffer: AAAAAAAA
Explanation:
In this example, we have a destination buffer buffer of size 10 bytes. We want to fill this buffer with the character 'A' using the memset() function.
The memset() function sets the value of each byte in the memory block pointed to by ptr to the specified value, which is 'A' in this case. The third argument, sizeof(buffer), specifies the number of bytes to be set, which is the size of the buffer. After the memset() function is called, all the bytes in the buffer are set to 'A', as seen in the output.
Note that the value argument is of type int, but it is converted to an unsigned char before being stored in the memory block. This means that only the least significant byte of the int value is used for setting the memory block. Additionally, the size of the memory block to be set is specified in bytes using the size_t data type, which is an unsigned integer type defined by the C standard library.
The memset() function is commonly used to initialize memory blocks, such as arrays or structs, to a known value or to zero out memory blocks before using them to store data. It's important to use memset() carefully, ensuring that the correct size and value are specified to avoid overwriting memory beyond the intended boundaries.
6. strcat()
The function strcat()
is used to concatenate (append) two strings. It takes two arguments: the destination string and the source string.
Return type: char*
Arguments of the function: destination string, source string
Code snippet:
char *strcat(char *dest, const char *src)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Destination and source strings
char dest[20] = "Hello";
char src[] = " World!";
// Concatenate the source string to the destination string
strcat(dest, src);
printf("Concatenated String: %s\n", dest);
return 0;
}
Output:
Concatenated String: Hello World!
Explanation:
In this example, we have a destination string dest with a size of 20 bytes, initialized with the value "Hello", and a source string src with the value " World!".
The strcat() function appends the characters of the source string src to the end of the destination string dest. It concatenates the two strings by copying the characters from the source string to the end of the destination string, and adds a null-terminating character ('\0') at the end to mark the end of the concatenated string.
After the strcat() function is called, the destination string dest now contains the concatenated string "Hello World!", as seen in the output.
Note that the destination string dest must have enough space to accommodate the concatenated string, including the null-terminating character. If the destination string does not have enough space, it may result in undefined behavior, such as buffer overflow. It's important to ensure that the destination string has enough space to accommodate the concatenated string and to use strcat() carefully to avoid potential buffer overflow vulnerabilities.
7. strncat()
The function strncat()
is used to concatenate (append) a specified number of characters from a source string to a destination string. It takes three arguments: the destination string, the source string, and the maximum number of characters to concatenate.
Return type: char*
Arguments of the function: destination string, source string, maximum number of characters to concatenate
Code snippet:
char *strncat(char *dest, const char *src, size_t n)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Destination and source strings
char dest[20] = "Hello";
char src[] = " World!";
size_t n = 6;
// Concatenate the first n characters from the source string to the destination string
strncat(dest, src, n);
printf("Concatenated String: %s\n", dest);
return 0;
}
Output:
Concatenated String: Hello World
Explanation:
In this example, we have a destination string dest with a size of 20 bytes, initialized with the value "Hello", a source string src with the value " World!", and a variable n with the value 6.
The strncat() function appends the first n characters from the source string src to the end of the destination string dest. It concatenates the specified number of characters from the source string to the end of the destination string, and adds a null-terminating character ('\0') at the end to mark the end of the concatenated string.
After the strncat() function is called, the destination string dest now contains the concatenated string "Hello World", as seen in the output.
Note that the destination string dest must have enough space to accommodate the concatenated string, including the null-terminating character. Also, the maximum number of characters to concatenate, specified by n, should not exceed the length of the source string src to avoid potential buffer overflow. It's important to ensure that the destination string has enough space and to use strncat() carefully to avoid potential buffer overflow vulnerabilities.
8. strchr()
The function strchr()
is used to search for the first occurrence of a specified character in a given string. It takes two arguments: the string to be searched, and the character to be searched for.
Return type: char*
Arguments of the function: string to be searched, character to be searched for
Code snippet:
char *strchr(const char *s, int c)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// String to be searched
char str[] = "Hello World";
// Character to be searched for
char ch = 'W';
// Search for the first occurrence of the character in the string
char *result = strchr(str, ch);
if (result != NULL) {
printf("Character found at position: %ld\n", result - str + 1);
} else {
printf("Character not found\n");
}
return 0;
}
Output:
Character found at position: 7
Explanation:
In this example, we have a string str with the value "Hello World" and a character ch with the value 'W'.
The strchr() function searches for the first occurrence of the character ch in the string str. It returns a pointer to the first occurrence of the character in the string, or a NULL pointer if the character is not found.
In the example code, the strchr() function is called with the arguments str and ch, and the resulting pointer is stored in the variable result. If the character is found, the position of the character in the string is calculated by subtracting the base address of the string str from the address of the character found (result), and adding 1 to account for 0-based indexing in C. The position is then printed as the output.
Note that strchr() only searches for a single character, and it stops searching when the character is found or when the end of the string is reached. It does not search for substrings or multiple occurrences of the character.
9. strcmp()
The function strcmp()
is used to compare two strings lexicographically (i.e., character by character) to determine if they are equal, less than, or greater than each other. It takes two arguments: the two strings to be compared.
Return type: int
Arguments of the function: string1, string2
Code snippet:
int strcmp(const char *string1, const char *string2)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Strings to be compared
char str1[] = "Hello";
char str2[] = "World";
// Compare the two strings
int result = strcmp(str1, str2);
if (result == 0) {
printf("Strings are equal\n");
} else if (result < 0) {
printf("String1 is less than String2\n");
} else {
printf("String1 is greater than String2\n");
}
return 0;
}
Output:
String1 is less than String2
Explanation:
In this example, we have two strings str1 and str2 with the values "Hello" and "World", respectively.
The strcmp() function compares the two strings lexicographically. It returns an integer value that indicates the relationship between the two strings. The return value is 0 if the strings are equal, a negative value if string1 is less than string2, and a positive value if string1 is greater than string2.
In the example code, the strcmp() function is called with the arguments str1 and str2, and the resulting integer value is stored in the variable result. The if-else block is used to check the value of result and print the corresponding output indicating the relationship between the two strings.
Note that strcmp() compares strings based on the ASCII values of the characters, so it performs a case-sensitive comparison. If you need to perform a case-insensitive comparison, you can use the strcasecmp() function instead (in case of POSIX-compliant systems) or implement your own custom function.
10. strncmp()
The function strncmp()
is similar to strcmp()
, but it compares only the first n characters of two strings, where n is specified as an argument. It is used to compare two strings lexicographically (i.e., character by character) up to a specified number of characters, to determine if they are equal, less than, or greater than each other. It takes three arguments: the two strings to be compared, and the maximum number of characters to compare.
Return type: int
Arguments of the function: string1, string2, n
Code snippet:
int strncmp(const char *string1, const char *string2, size_t n)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Strings to be compared
char str1[] = "Hello";
char str2[] = "Heaven";
// Compare the first 3 characters of the two strings
int result = strncmp(str1, str2, 3);
if (result == 0) {
printf("The first 3 characters of the strings are equal\n");
} else if (result < 0) {
printf("The first 3 characters of String1 are less than String2\n");
} else {
printf("The first 3 characters of String1 are greater than String2\n");
}
return 0;
}
Output:
The first 3 characters of the strings are equal
Explanation:
In this example, we have two strings str1 and str2 with the values "Hello" and "Heaven", respectively.
The strncmp() function compares the first n characters of the two strings lexicographically, where n is specified as the third argument. It returns an integer value that indicates the relationship between the first n characters of the two strings. The return value is 0 if the first n characters of the strings are equal, a negative value if the first n characters of string1 are less than the first n characters of string2, and a positive value if the first n characters of string1 are greater than the first n characters of string2.
In the example code, the strncmp() function is called with the arguments str1, str2, and 3 (to compare the first 3 characters), and the resulting integer value is stored in the variable result. The if-else block is used to check the value of result and print the corresponding output indicating the relationship between the first n characters of the two strings.
Note that strncmp() is commonly used when comparing strings of different lengths or when comparing only a portion of strings, to avoid buffer overflow errors. It is a safer option compared to strcmp() when comparing strings with unknown lengths or when comparing strings that may not be null-terminated.
11. strcoll()
The strcoll()
function is used to compare two strings using the current locale's collating sequence. It takes two arguments: the two strings to be compared. This function performs a locale-specific string comparison, which takes into consideration the cultural and linguistic rules of the current locale. It is useful for performing string comparisons in a locale-sensitive manner, where the ordering of characters may differ depending on the language or region.
Return type: int
Arguments of the function: string1, string2
Code snippet:
int strcoll(const char *string1, const char *string2)
Example Code:
#include <stdio.h>
#include <string.h>
#include <locale.h>
int main() {
// Strings to be compared
char str1[] = "apple";
char str2[] = "banana";
// Set locale to English
setlocale(LC_COLLATE, "en_US.UTF-8");
// Compare the two strings using strcoll()
int result = strcoll(str1, str2);
if (result == 0) {
printf("The strings are equal\n");
} else if (result < 0) {
printf("String1 comes before String2\n");
} else {
printf("String1 comes after String2\n");
}
return 0;
}
Output:
String1 comes before String2
Explanation:
In this example, we have two strings str1 and str2 with the values "apple" and "banana", respectively.
The strcoll() function compares the two strings using the collating sequence of the current locale. The collating sequence is the order in which characters are sorted according to the linguistic rules of the locale, which may differ from the ASCII or Unicode order. The strcoll() function returns an integer value that indicates the relationship between the two strings based on the collating sequence. The return value is 0 if the two strings are equal, a negative value if string1 comes before string2 in the collating sequence, and a positive value if string1 comes after string2 in the collating sequence.
In the example code, the setlocale() function is used to set the locale to English (en_US.UTF-8) before calling strcoll(). The strcoll() function is then called with the arguments str1 and str2, and the resulting integer value is stored in the variable result. The if-else block is used to check the value of result and print the corresponding output indicating the relationship between the two strings based on the collating sequence of the current locale.
Note that strcoll() is useful for performing locale-sensitive string comparisons, where the ordering of characters may differ depending on the language or region. It is commonly used in multilingual applications or when dealing with user-generated content that may contain characters from different languages or regions.
12. strcpy()
The strcpy()
function is used to copy a string from one character array to another. It takes two arguments: the destination character array and the source character array. This function copies the characters from the source string to the destination string, including the null terminator, and terminates the destination string with a null character. It is important to ensure that the destination string has enough space to hold the entire source string, including the null terminator, to prevent buffer overflow.
Return type: char*
Arguments of the function: dest, src
Code snippet:
char *strcpy(char *dest, const char *src)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Source and destination strings
char src[] = "Hello, World!";
char dest[20];
// Copy source string to destination string
strcpy(dest, src);
// Print the copied string
printf("Copied string: %s\n", dest);
return 0;
}
Output:
Copied string: Hello, World!
Explanation:
In this example, we have a source string src with the value "Hello, World!" and a destination string dest with a size of 20 characters.
The strcpy() function is used to copy the entire source string to the destination string. The destination string must have enough space to hold the entire source string, including the null terminator. The strcpy() function terminates the destination string with a null character, which marks the end of the string. After the strcpy() function is executed, the destination string contains an exact copy of the source string.
In the example code, the strcpy() function is called with the arguments dest and src, which copy the source string to the destination string. The copied string is then printed using printf() function.
It is important to note that strcpy() does not perform any boundary checking, and it can result in buffer overflow if the destination string does not have enough space to hold the entire source string. To prevent buffer overflow, it is recommended to use strncpy() or other safer string manipulation functions that allow specifying the maximum number of characters to be copied.
13. strncpy()
The strncpy()
function is used to copy a specified number of characters from one character array to another. It takes three arguments: the destination character array, the source character array, and the number of characters to be copied. This function copies the specified number of characters from the source string to the destination string, and terminates the destination string with a null character. If the source string is shorter than the specified number of characters, the remaining characters in the destination string will be padded with null characters. It is important to ensure that the destination string has enough space to hold the specified number of characters, including the null terminator, to prevent buffer overflow.
Return type: char*
Arguments of the function: dest, src, n
Code snippet:
char *strncpy(char *dest, const char *src, size_t n)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// Source and destination strings
char src[] = "Hello";
char dest[10];
// Copy 5 characters from source string to destination string
strncpy(dest, src, 5);
// Print the copied string
printf("Copied string: %s\n", dest);
return 0;
}
Output:
Copied string: Hello
Explanation:
In this example, we have a source string src with the value "Hello" and a destination string dest with a size of 10 characters.
The strncpy() function is used to copy the first 5 characters from the source string to the destination string. The destination string must have enough space to hold the specified number of characters, including the null terminator. If the source string is shorter than the specified number of characters (in this case, 5), the remaining characters in the destination string will be padded with null characters. After the strncpy() function is executed, the destination string contains the first 5 characters of the source string "Hello".
In the example code, the strncpy() function is called with the arguments dest, src, and 5, which copy the first 5 characters from the source string to the destination string. The copied string is then printed using printf() function.
It is important to note that strncpy() does not guarantee null-termination of the destination string if the source string is longer than the specified number of characters. To ensure null-termination, it is recommended to manually add a null character at the end of the destination string after using strncpy(), if needed. Additionally, strncpy() does not perform any boundary checking, and it can result in buffer overflow if the destination string does not have enough space to hold the specified number of characters. To prevent buffer overflow, it is recommended to use other safer string manipulation functions that allow specifying the maximum number of characters to be copied, such as strlcpy() or snprintf().
14. strcspn()
The strcspn()
function calculates the length of the initial segment of a string that does not contain any of the characters specified in a search string. It takes two arguments: the string to be searched and the search string. It returns the number of characters in the initial segment of the string that do not match any of the characters in the search string.
Return type: size_t
Arguments of the function: str1, str2
Code snippet:
size_t strcspn(const char *str1, const char *str2)
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
// String to be searched
char str1[] = "Hello World";
// Search string
char str2[] = "aeiou";
// Calculate the length of the initial segment without vowels
size_t len = strcspn(str1, str2);
// Print the length
printf("Length of initial segment without vowels: %zu\n", len);
return 0;
}
Output:
Length of initial segment without vowels: 2
Explanation:
In this example, we have a string str1 with the value "Hello World" and a search string str2 with the value "aeiou" which contains the vowels "a", "e", "i", "o", and "u".
The strcspn() function is used to calculate the length of the initial segment of the string str1 that does not contain any of the characters in the search string str2. In this case, the function returns the value 2, which represents the length of the initial segment "H" and "e" in the string "Hello World" that do not match any of the characters in the search string "aeiou".
In the example code, the strcspn() function is called with the arguments str1 and str2 to calculate the length of the initial segment without vowels in the string "Hello World". The calculated length is then printed using printf() function.
It is important to note that strcspn() stops counting the characters in the initial segment as soon as any character from the search string is found in the input string. Additionally, strcspn() returns the length of the initial segment without matching any of the characters in the search string, which means it does not include the null terminator in the count.
15. strerror()
The strerror()
function is used to retrieve the error message string associated with an error code returned by a previous function call. It takes an integer error code as an argument and returns a string representing the corresponding error message.
Return type: char *
Argument of the function: errnum
Code snippet:
char *strerror(int errnum);
Example Code:
#include <stdio.h>
#include <string.h>
#include <errno.h>
int main() {
// Simulate an error
FILE *file = fopen("nonexistent_file.txt", "r");
// Check for error and print error message
if (file == NULL) {
printf("Error: %s\n", strerror(errno));
}
return 0;
}
Output:
Error: No such file or directory
Explanation:
In this example, we are trying to open a non-existent file "nonexistent_file.txt" using the fopen() function. If the file does not exist, the fopen() function will return NULL, indicating an error.
To retrieve the error message associated with the error code, we use the strerror() function with the errno variable, which is set by the system to the error code of the last function that failed. The strerror() function returns a string representing the error message corresponding to the error code.
In the example code, the strerror() function is called with the errno variable as the argument to retrieve the error message associated with the error code from the fopen() function. The error message "No such file or directory" is then printed using printf() function.
It's important to note that the strerror() function returns a pointer to a string containing the error message, which is typically a static string that should not be modified. The error messages returned by strerror() are usually implementation-defined, meaning they may vary depending on the operating system and library implementation used.
16. strlen()
The strlen()
function is used to determine the length of a null-terminated string in C. It takes a pointer to a string as an argument and returns the number of characters in the string, excluding the null terminator.
Return type: size_t
Argument of the function: str
Code snippet:
size_t strlen(const char *str);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
char myString[] = "Hello, world!";
size_t len = strlen(myString);
printf("Length of string: %zu\n", len);
return 0;
}
Output:
Length of string: 13
Explanation:
In this example, we declare a character array myString containing the string "Hello, world!". The strlen() function is then called with myString as the argument to determine the length of the string, which is the number of characters in the string excluding the null terminator.
The strlen() function returns a value of type size_t, which is an unsigned integer type defined in the <stddef.h> header. It represents the size of objects in bytes and is used for storing sizes and indices of arrays.
In the example code, the length of the string "Hello, world!" is determined by calling strlen() with myString as the argument, and the result is stored in the len variable. The length of the string is then printed using printf() function with the %zu format specifier, which is used for printing values of type size_t.
It's important to note that the strlen() function only counts the number of characters in the string until the null terminator is encountered. It does not include the null terminator in the returned length. Also, strlen() function does not include any trailing white spaces or other invisible characters in the string when calculating the length.
17. strpbrk()
The strpbrk()
function is used to find the first occurrence of any character from a given set of characters in a string in C. It takes two arguments: a pointer to a string and a pointer to a string containing a set of characters to search for. It returns a pointer to the first occurrence of any character from the given set in the string, or NULL
if no such character is found.
Return type: char *
Arguments of the function: str, charset
Code snippet:
char *strpbrk(const char *str, const char *charset);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
char myString[] = "Hello, world!";
char *result = strpbrk(myString, "ow");
if (result != NULL) {
printf("Found: %c\n", *result);
} else {
printf("Not found.\n");
}
return 0;
}
Output:
Found: o
Explanation:
In this example, we declare a character array myString containing the string "Hello, world!". The strpbrk() function is then called with myString as the first argument and the string "ow" as the second argument, which represents the set of characters to search for. The function returns a pointer to the first occurrence of any character from the set "ow" in the string "Hello, world!".
The strpbrk() function returns a pointer to a character in the input string str that matches any character in the input string charset, or NULL if no match is found. The returned pointer points to the first occurrence of any character from the given set in the input string.
In the example code, the strpbrk() function is called with myString and "ow" as arguments, and the returned pointer is stored in the result variable. The value of result is then checked to see if it is not NULL, indicating that a match was found. If a match was found, the character pointed to by result is printed using printf() function with the %c format specifier.
It's important to note that strpbrk() function searches for the first occurrence of any character from the given set, and it does not search for the entire string charset as a substring in the input string str. Also, strpbrk() function searches for characters in the input string str until a null terminator is encountered or until the end of the string is reached.
18. strrchr()
The strrchr()
function is used to find the last occurrence of a specific character in a string in C. It takes two arguments: a pointer to a string and an integer representing the character to search for. It returns a pointer to the last occurrence of the character in the string, or NULL
if the character is not found.
Return type: char *
Arguments of the function: str, ch
Code snippet:
char *strrchr(const char *str, int ch);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
char myString[] = "Hello, world!";
char *result = strrchr(myString, 'l');
if (result != NULL) {
printf("Found: %c\n", *result);
} else {
printf("Not found.\n");
}
return 0;
}
Output:
Found: l
Explanation:
In this example, we declare a character array myString containing the string "Hello, world!". The strrchr() function is then called with myString as the first argument and the character 'l' as the second argument, which represents the character to search for. The function returns a pointer to the last occurrence of the character 'l' in the string "Hello, world!".
The strrchr() function searches for the last occurrence of the character ch in the input string str, and returns a pointer to the last occurrence of the character in the string. If the character is not found, the function returns NULL.
In the example code, the strrchr() function is called with myString and 'l' as arguments, and the returned pointer is stored in the result variable. The value of result is then checked to see if it is not NULL, indicating that a match was found. If a match was found, the character pointed to by result is printed using printf() function with the %c format specifier.
It's important to note that strrchr() function searches for the last occurrence of the specified character in the input string str, and it does not search for a substring in the string. Also, strrchr() function searches for the character ch in the input string str until a null terminator is encountered or until the end of the string is reached.
19. strspn()
The strspn()
function is used to calculate the length of the initial segment of a string that consists entirely of characters from a specified set of characters in C. It takes two arguments: a pointer to the string to be searched, and a pointer to a string containing the characters to search for. It returns an integer representing the length of the initial segment of the string that matches the characters in the specified set, or 0 if no characters match.
Return type: size_t
Arguments of the function: str, accept
Code snippet:
size_t strspn(const char *str, const char *accept);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
char myString[] = "Hello123";
char accept[] = "Helo";
size_t len = strspn(myString, accept);
printf("Length of initial segment: %zu\n", len);
return 0;
}
Output:
Length of initial segment: 5
Explanation:
In this example, we declare a character array myString containing the string "Hello123". We also declare another character array accept containing the characters "Helo". The strspn() function is then called with myString as the first argument and accept as the second argument, which represents the set of characters to search for. The function returns the length of the initial segment of myString that matches the characters in the set accept, which is 5 in this case.
The strspn() function calculates the length of the initial segment of the input string str that consists entirely of characters from the set of characters in the input string accept. It scans the characters in str from the beginning until a character is encountered that is not present in accept, or until the end of the string is reached.
In the example code, the strspn() function is called with myString and accept as arguments, and the returned value is stored in the len variable. The value of len is then printed using printf() function with the %zu format specifier, which is used for size_t type.
It's important to note that the strspn() function only calculates the length of the initial segment of the string that matches the characters in the specified set, and it does not search for a substring in the string. Also, the function does not perform any null terminator check, so make sure the input strings are null-terminated to avoid any unexpected behavior.
20. strstr()
The strstr()
function is used to locate the first occurrence of a substring within a string in C. It takes two arguments: a pointer to the string to be searched, and a pointer to the substring to search for. It returns a pointer to the first occurrence of the substring in the string, or NULL
if the substring is not found.
Return type: char *
Arguments of the function: haystack, needle
Code snippet:
char *strstr(const char *haystack, const char *needle);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
char haystack[] = "Hello world";
char needle[] = "world";
char *result = strstr(haystack, needle);
if (result != NULL) {
printf("Substring found at position: %ld\n", result - haystack);
} else {
printf("Substring not found\n");
}
return 0;
}
Output:
Substring found at position: 6
Explanation:
In this example, we declare a character array haystack containing the string "Hello world". We also declare another character array needle containing the substring "world". The strstr() function is then called with haystack as the first argument and needle as the second argument, which represents the substring to search for. The function returns a pointer to the first occurrence of the substring "world" in haystack, which is stored in the result variable. Since the result is not NULL, it means the substring was found, and the position of the substring in the haystack string is calculated by subtracting haystack from result and printed using printf() function with the %ld format specifier.
The strstr() function searches the input string haystack for the first occurrence of the substring needle. It returns a pointer to the first occurrence of needle in haystack, or NULL if needle is not found. The search is case-sensitive, so the function will not match substrings with different case.
In the example code, the strstr() function is called with haystack and needle as arguments, and the returned value is stored in the result variable. If result is not NULL, it means the substring was found, and the position of the substring in the haystack string is calculated by subtracting haystack from result. If result is NULL, it means the substring was not found.
It's important to note that the strstr() function only searches for the first occurrence of the substring in the string. If you need to search for multiple occurrences, you may need to use a loop or other techniques. Also, the function does not perform any null terminator check, so make sure the input strings are null-terminated to avoid any unexpected behavior.
21. strtok()
The strtok()
function is used to tokenize a string by splitting it into smaller tokens based on a specified delimiter in C. It takes two arguments: a pointer to the string to be tokenized, and a pointer to a string containing the delimiter characters. It returns a pointer to the next token found in the input string, or NULL
if no more tokens are found.
Return type: char *
Arguments of the function: str, delim
Code snippet:
char *strtok(char *str, const char *delim);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello,world;how are you?";
const char *delim = ",;";
char *token = strtok(str, delim);
while (token != NULL) {
printf("Token: %s\n", token);
token = strtok(NULL, delim);
}
return 0;
}
Output:
Token: Hello
Token: world
Token: how are you?
Explanation:
In this example, we declare a character array str containing the string "Hello,world;how are you?". We also declare a constant character pointer delim containing the delimiter characters ",;". The strtok() function is then called with str as the first argument and delim as the second argument, which represents the delimiters to split the string by. The function returns a pointer to the first token found in the str string, which is stored in the token variable.
In the while loop, we iterate through the tokens by calling strtok() with NULL as the first argument, which tells the function to continue tokenizing from the last position it left off. The returned value is stored in the token variable, and if it's not NULL, it means a new token was found. The token is then printed using printf() function with the %s format specifier.
The strtok() function uses the delimiter characters specified in delim to split the input string str into smaller tokens. It replaces the delimiter characters with null terminators, and returns a pointer to the first token found in the input string. Subsequent calls to strtok() with NULL as the first argument continue tokenizing from the last position it left off. Once no more tokens are found, the function returns NULL.
It's important to note that the strtok() function modifies the input string str by replacing the delimiter characters with null terminators. If you need to keep the original string intact, you should create a copy of the string before calling strtok(). Also, be cautious when using strtok() in multithreaded environments, as it uses a static internal state that may not be thread-safe. In such cases, you may need to use a thread-safe alternative such as strtok_r().
22. strxfrm()
The strxfrm()
function is used to transform a string according to the current locale in C. It takes two arguments: a pointer to a character array that will store the transformed string, and a pointer to a string to be transformed. The function returns the length of the transformed string, which may be different from the length of the input string.
Return type: size_t
Arguments of the function: dest, src, n
Code snippet:
size_t strxfrm(char *dest, const char *src, size_t n);
Example Code:
#include <stdio.h>
#include <string.h>
int main() {
const char *src = "Hello, world!";
char dest[20];
size_t len = strxfrm(dest, src, sizeof(dest));
printf("Transformed string: %s\n", dest);
printf("Length of transformed string: %zu\n", len);
return 0;
}
Output:
Transformed string: Hello, world!
Length of transformed string: 13
Explanation:
In this example, we declare a constant character pointer src containing the string "Hello, world!". We also declare a character array dest with a size of 20, which will be used to store the transformed string. The strxfrm() function is then called with dest as the first argument, src as the second argument, and sizeof(dest) as the third argument, which represents the size of the destination buffer. The function transforms the src string according to the current locale and stores the result in the dest buffer.
The strxfrm() function transforms the input string src according to the rules of the current locale, which may involve modifying the characters, changing their order, or adding additional characters. The transformed string is stored in the dest buffer, which must have enough space to accommodate the transformed string, including the null terminator. The function returns the length of the transformed string, which may be different from the length of the input string.
It's important to note that the strxfrm() function is locale-dependent, meaning that its behavior may vary depending on the current locale setting. Different locales may have different rules for transforming strings, and the same input string may be transformed differently in different locales. To ensure consistent behavior, it's recommended to set the desired locale using the setlocale() function before calling strxfrm().
Conclusion:
The string.h library in C provides a rich set of functions for manipulating strings, making it a powerful tool for programmers dealing with text data. In this article, we covered various functions from the string.h library, including memcpy(), memmove(), memset(), strcat(), strncat(), strchr(), strcmp(), strncmp(), strcoll(), strcpy(), strncpy(), strcspn(), strerror(), strlen(), strpbrk(), strrchr(), strspn(), strstr(), strtok(), and strxfrm().
Each function was described in detail, including its purpose, return type, arguments, and example code. Additionally, explanations were provided to clarify the behavior and usage of each function, highlighting their strengths and practical applications.
The string.h library offers immense convenience and flexibility to programmers. It enables efficient manipulation of strings, including copying, concatenating, comparing, and transforming strings, among other operations. These functions can be used in various scenarios, such as data processing, text parsing, and string manipulation in applications ranging from simple utilities to complex systems.
With its extensive set of functions, the string.h library simplifies string manipulation tasks and provides reliable and efficient solutions. Its versatility and wide range of capabilities make it a valuable tool for developers working with strings in C. By leveraging the functions provided by the string.h library, programmers can enhance the functionality and performance of their C programs, making string handling tasks more efficient and convenient.
In conclusion, the string.h library is a fundamental part of C programming, offering a rich set of functions that facilitate string manipulation tasks. Whether it's copying, concatenating, comparing, or transforming strings, the string.h library provides a convenient and efficient way to handle text data. By leveraging the capabilities of the string.h library, programmers can greatly enhance the functionality and robustness of their C programs, making it an indispensable tool for string manipulation tasks.
Thank You!
Shreyas Sukhadeve.
Contact at shreyassukhadeve[at]gmail.com
for any issues or doubts regarding the string.h article at OpenGenus.
Happy Coding!