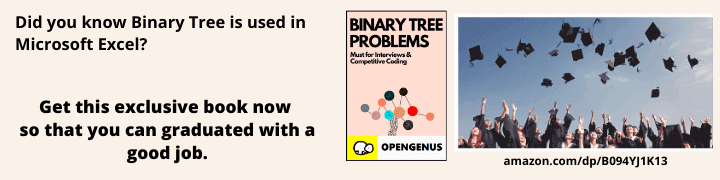
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to read last N characters from a file in C programming language along with a complete C program.
Table of contents:
- Approach
- C Program to read last N characters from file
Approach
In C, we can open a file as a pointer as follows:
FILE *fp;
fp = fopen("opengenus.txt", "r");
We can read a character using fgetc function. This reads the character from the starting of the file:
ch = fgetc(fp);
On calling fgetc again, it will fetch the 2nd character and so on. The pointer in the file moves further into the file with each fgetc call.
One approach is to read all characters and then, calculate and return only the last N characters. This is an expensive approach. The real approach will be to move the pointer to the N-th place from the last and then, use fgetc method for N times.
To move the pointer to the N-th position from the end:
- Go to the last position of the file
- Get length of file that is number of characters
- Go to the position at length - N
This C code snippet captures the idea:
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fseek(fp, (length - number), SEEK_SET);
After this, simply read the characters until end of file (EOF) is reached.
do {
ch = fgetc(fp);
putchar(ch);
} while (ch != EOF);
This gives last N characters from file efficiently.
The steps to read last N characters from file in C are as follows:
- Open the file as a file pointer in C
- Move pointer in the file to the N-th position from the last
- Read all characters till the end of file.
C Program to read last N characters from file
Following is the complete C Program to read last N characters from file named "opengenus.txt":
// Part of iq.opengenus.org
#include<stdio.h>
int main() {
FILE *fp;
char ch;
// Read last num characters from end
int number = 10;
long length;
fp = fopen("opengenus.txt", "r");
if (fp == NULL) {
puts("cannot open this file");
exit(1);
}
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fseek(fp, (length - number), SEEK_SET);
do {
ch = fgetc(fp);
putchar(ch);
} while (ch != EOF);
fclose(fp);
return(0);
}
With this article at OpenGenus, you must have the complete idea of how to read last N characters from file in C Programming Language.