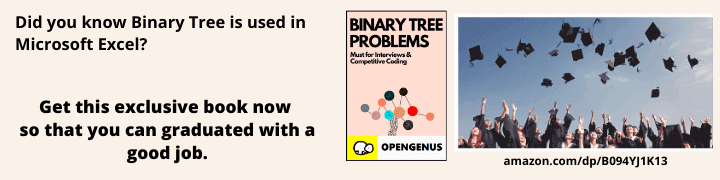
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the approach to find the length of a string using Pointer in C Programming Language.
Table of contents:
- Approach
- C Program to find Length of a String using Pointer
Understand:
Approach
In C programming language, a string is an array of characters that is a character pointer. A string in C is as follows:
char* string
One can get the current character as "*p
". This refers to the first character. On incrementing p (p++), *p
is the second character and so on.
The last character of a string is always a Null Character that is \0
.
So, the approach to find Length of a String using Pointer is as follows:
- Get the string as a character array
- Compare the current character at current pointer with
\0
- Increment pointer to get to next character
- If current character is not null, increment counter else current counter is the length of string.
We can traverse an array by just incrementing its pointer:
while (true) {
p++;
}
The current needs to be compared with null character:
*p = '\0';
If p is a pointer, then the data at the pointer location is accessed using *p
.
In C, this approach can be implemented as follows as a function:
int getLength(char* p) {
int count = 0;
while (*p != '\0') {
count++;
p++;
}
return count;
}
C Program to find Length of a String using Pointer
Following is the complete C Program to find Length of a String using Pointer:
// Part of iq.opengenus.org
#include<stdio.h>
int getLength(char*p) {
int count = 0;
while (*p != '\0') {
count++;
p++;
}
return count;
}
int main() {
char str[20] = "OpenGenus";
int length = 0;
length = getLength(str);
printf("The length of the string \"%s\" is %d", str, length);
return 0;
}
Output:
The length of the string "OpenGenus" is 9
With this article at OpenGenus, you must have the complete idea of how to find implement a C Program to find Length of a String using Pointer.