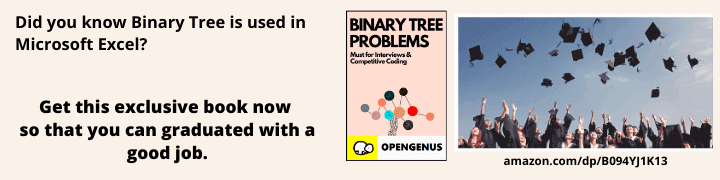
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will check whether the given alphabet or letter is a vowel, consonant or an alphabet. We have demonstrated this with a C program.
TABLE OF CONTENT
- Question
- Approach to the solution
- Implementation
- Output
QUESTION
The problem is to write a pogram in C to read a character from the user and test it whether it a vowel or consonant or not an alphabet.
For example if we choose the alphabet "A", the output should be an vowel
APPROACH TO THE SOLUTION
Here Vowel: is defined as the any of the sounds represented in English by the letters a, e, i, o, u or A,E,I,O,U.
Consonant: is defined are the alphabets except vowels
An alphabet: is a set of graphs or characters used to represent the phonemic structure of a language. In most alphabets, the characters are arranged in a definite order or sequence (e.g., A, B, C, etc.).
Here, we gonna use if else stament by C pogramming.
Now, let us know about if else statment.
When we run the code if the statment is true inside the f blocks the computer shows the f block statment.
If the statment is wrong the else block is shown
THE STRUCTRE OF IF ELSE STATMENT
if(statment conditon)
{
printf("the statment");
}
else
{
printf("the false statment");
}
- LIBRARY AND FUNCTION
To enable the program to take user input, we need to use stdio.h library in C. stdio stands for "Standard Input and Output". It is used within the main function ("int main()")
#include <stdio.h>
2.DECLARATION OF DATATYPE
Here we are going to use character (char) data type
which is used to store alphabets and characters for example "1","a","@"
3.INTRODUCTION TO VARIABELS AND USER INPUT
Here we are using ch as a variable to store our character or alphabets since the character is an user input
we will scanf function is an input function
char ch;
printf("enter an alphabet :");
scanf("%char"&ch);
The ampersand (&) allows us to pass the address of variable number
4.THE IF ELSE COMMAND
Here we gonna start if else command
- 1st we will write the if command to check wether the character is an alphabet or not
if((ch>='a' && ch<='z')||(ch>='A' && ch<='Z'))
- inside it we will write another if command to check wether the alphabet is vowel or consonent so here we are using nested if else
if((ch>='a' && ch<='z')||(ch>='A' && ch<='Z'))
{
if(ch=='a'||ch=='A'||ch=='e'||ch=='E'||
ch=='i'||ch=='I'||ch=='o'||ch=='O'||
ch=='u'||ch=='U')
printf("the alphabet is an vowel\n");
else
printf("the alphabet is an consonent\n");
}
- At last, we will end the if else by the last else command which is:
else
printf("the given character is not an alphabet");
return 0;
}
IMPLEMENTATION IN C
Following is the complete C program:
#include<stdio.h>
int main()
{
char ch;
printf("enter an alphabet :\n");
scanf("%c",&ch);
if((ch>='a' && ch<='z')||(ch>='A' && ch<='Z'))
{
if(ch=='a'||ch=='A'||ch=='e'||ch=='E'||ch=='i'||ch=='I'||ch=='o'||ch=='O'||ch=='u'||ch=='U')
printf("the alphabet is an vowel\n");
else
printf("the alphabet is an consonant\n");
}
else
printf("the given character is not an alphabet");
return 0;
}
OUTPUT
Output:
enter an alphabet :
b
the alphabet is an consonant
In this article at OpenGenus, you must have the complete idea of how to detect a character as a vowel or consonant in C Programming.