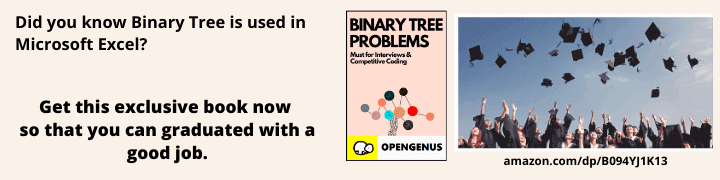
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will take a look at the key features a movie ticket booking system needs to offer, its high-level, low-level design, database design, and some of the already existing library management software.
The key requirements that need to be offered by the Movie Ticket Booking System can be classified into functional and non-functional requirements.
Functional Requirements
- Allow users to search for movies by title, genre, actor, or release date, and view movie details such as showtimes, ratings, and reviews.
- Users can book movie tickets, select seats, and make payments using a secure payment gateway.
- The system should send a confirmation email or SMS to the user with the booking details and QR code for entry.
- Allow users to cancel or modify their bookings, and refund the appropriate amount based on the cancellation policy.
- The system should notify the user and the theater management about any changes to the show timings, cancellations, or rescheduling of movies.
- Provide an admin dashboard for the theater management to add and manage movies, update show timings, and view sales reports.
- The system should ensure data privacy and security, and protect against fraud and unauthorized access.
Non-Functional Requirements
- The system should be highly available and scalable to handle peak loads during weekends and holidays.
- The system should be user-friendly, responsive, and accessible on different devices and platforms.
- The system should have a fast and reliable search and booking engine, and provide real-time updates on seat availability and pricing.
- The system should have a robust and reliable payment gateway, and ensure secure and seamless transactions.
- The system should comply with industry standards and regulations, and protect the user's personal and financial data.
In this diagram, there are two primary actors: User and Admin. The User can search for movies, view movie details, book tickets, view movie times, make payments, modify bookings, and cancel bookings. The Admin can manage movies and showtimes.
The use cases are represented as ovals, and the actors are represented as stick figures. The arrows show the flow of information between the actors and use cases.
Software Requirements
- A server running Windows Server/Linux OS
- A multi-threading capable backend language like JavaScript
- Front-end frameworks like React/Vue for the client
- Relational DBMS like MySQL, PostgreSQL, etc
- Containers and orchestration services like Kubernetes (for a large setting like a national library).
JavaScript version of the low-level design and classes for a movie ticket booking system with User and Admin roles:
class Person {
constructor(firstName, lastName, gender, address, phoneNo) {
this.firstName = firstName;
this.lastName = lastName;
this.gender = gender;
this.address = address;
this.phoneNo = phoneNo;
}
}
class User extends Person {
constructor(firstName, lastName, gender, address, phoneNo, email, password) {
super(firstName, lastName, gender, address, phoneNo);
this.email = email;
this.password = password;
}
viewMovies() {
// view movies logic here
}
searchMovies(searchQuery) {
// search movies logic here
}
bookTicket(movie, showtime, seats) {
// book ticket logic here
}
makePayment(paymentInfo) {
// make payment logic here
}
modifyBooking(bookingId, newShowtime, newSeats) {
// modify booking logic here
}
cancelBooking(bookingId) {
// cancel booking logic here
}
}
class Admin extends Person {
constructor(firstName, lastName, gender, address, phoneNo, email, password) {
super(firstName, lastName, gender, address, phoneNo);
this.email = email;
this.password = password;
}
manageMovies() {
// manage movies logic here
}
manageShowtimes() {
// manage showtimes logic here
}
viewBookings() {
// view bookings logic here
}
cancelBooking(bookingId) {
// cancel booking logic here
}
}
class Movie {
constructor(title, genre, director, cast, synopsis, duration, releaseDate, image) {
this.title = title;
this.genre = genre;
this.director = director;
this.cast = cast;
this.synopsis = synopsis;
this.duration = duration;
this.releaseDate = releaseDate;
this.image = image;
}
}
class Showtime {
constructor(movie, startTime, endTime, theater, seats) {
this.movie = movie;
this.startTime = startTime;
this.endTime = endTime;
this.theater = theater;
this.seats = seats;
}
bookSeats(numSeats) {
// book seats logic here
}
cancelSeats(numSeats) {
// cancel seats logic here
}
}
class Booking {
constructor(user, showtime, seats, paymentInfo) {
this.user = user;
this.showtime = showtime;
this.seats = seats;
this.paymentInfo = paymentInfo;
this.status = "Booked";
}
modifyBooking(newShowtime, newSeats) {
// modify booking logic here
}
cancelBooking() {
// cancel booking logic here
}
}
class Payment {
constructor(cardholderName, cardNumber, expiryDate, cvv) {
this.cardholderName = cardholderName;
this.cardNumber = cardNumber;
this.expiryDate = expiryDate;
this.cvv = cvv;
}
makePayment(amount) {
// make payment logic here
}
}
The User class contains the basic information of a user, such as name, email, phone number, etc. It also has a list of bookings made by the user and methods to view movies, search for movies, book tickets, make payments, modify bookings, and cancel bookings.
The Admin class inherits from the User class and has additional methods to manage movies and show times. The Admin can add, delete, or modify movie details such as title, description, genre, duration, and rating. The Admin can also set the show times for each movie and manage the available seats for each show.
Overall, the system provides a simple and efficient way for users to book movie tickets and for admins to manage the movie details and show times. The classes and methods provided above can serve as a foundation for developing a robust movie ticket booking system using JavaScript.
Database Design in Movie Ticket Booking System
The above database includes five tables - Booking, Movie, Payment, Show Time, Admin, and User.
The Booking table stores details related to the ticket booking such as the booking ID, user ID, showtime, number of seats booked, payment ID, and show ID. The table is defined with a primary key constraint on the ID column.
The Movie table stores information about movies such as title, genre, director, cast, synopsis, duration, release date, and image. The table is defined with a primary key constraint on the ID column.
The Payment table stores details about the payment made for the ticket booking, such as the payment ID, cardholder name, card number, expiry date, and CVV. The table is defined with a primary key constraint on the ID column.
The Show Time table stores information related to the movie shows such as the show ID, movie ID, start time, end time, theater, and available seats. The table is defined with a primary key constraint on the ID column and also has a foreign key constraint on the movie ID column referencing the Movie table.
The Admin table stores details about the system administrator such as email, password, first name, last name, gender, address, and phone number. The table is defined with a primary key constraint on the ID column.
The User table stores details about the users such as first name, last name, gender, address, and phone number. The table is defined with a primary key constraint on the ID column.
Foreign key constraints are defined on the Booking table to reference the user ID column of the User table, the payment ID column of the Payment table, and the show ID column of the Show Time table.
Overall, the database schema is well-designed and provides a robust foundation for building a movie ticket booking system.
Leading Movie Ticket Booking Systems
Leading Movie Ticket Booking Systems are:
- BookMyShow
- Atom Tickets
- Fandango
- MovieTickets.com
Movie Ticket Booking Systems
-
BookMyShow is a popular online movie ticket booking platform that offers movie ticket booking for theaters across India, UAE, and Indonesia. It also provides the option to book tickets for sports events, concerts, and theater shows.
-
Atom Tickets is a mobile-first movie ticket booking platform that allows users to purchase tickets, order concessions, and invite friends to join the movie experience. It also provides personalized recommendations based on user preferences.
-
Fandango is a movie ticket booking platform that offers ticket booking for more than 40,000 theaters across the United States. It also provides movie reviews, trailers, and celebrity interviews.
-
MovieTickets[]com is a global movie ticket booking platform that allows users to purchase tickets for movies playing in more than 20 countries. It also provides movie reviews and showtimes.
These leading movie ticket booking systems offer users a seamless movie ticket booking experience with a wide range of features and options to choose from. From personalized recommendations to access to movie reviews and trailers, these platforms make it easy for users to plan and enjoy their movie-going experience.
With this article at OpenGenus, you must have the complete idea of System Design of Movie Ticket Booking System.