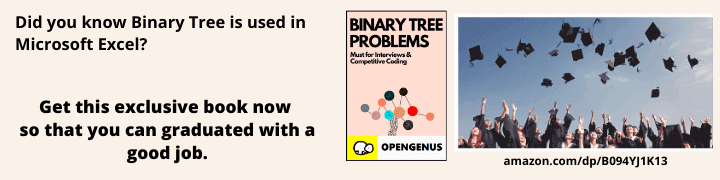
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
KEY TAKEAWAYS
- Enhance practical problem-solving skills through gas station optimization strategies.
- Master Binary Search adaptation for decimal-answer problems.
- Conduct comparative analysis of algorithmic approaches.
- Develop step-by-step problem-solving techniques.
- Achieve efficient precision in optimization problems.
Table of Contents:
- Problem Statement OverView
- Understanding the Problem
- Approach 1: Brute Force
- Approach 2: Heap-Based Solution
- Approach 3: Binary Search
- Comparative Analysis of Approaches
Problem Statement:
You are given a sorted array ‘arr’ of length ‘n’, which contains positive integer positions of ‘n’ gas stations on the X-axis. You are also given an integer ‘k’. You have to place ‘k’ new gas stations on the X-axis. You can place them anywhere on the non-negative side of the X-axis, even on non-integer positions. Let ‘dist’ be the maximum value of the distance between adjacent gas stations after adding k new gas stations.
Find the minimum value of ‘dist’.
Note:
Answers within 10^-6 of the actual answer will be accepted. For example, if the actual answer is 0.65421678124, it is okay to return 0.654216. Our answer will be accepted if that is the same as the actual answer up to the 6th decimal place.
Example 1:
Input:
For k=4 and arr = {1,2,3,4,5}
Output:
The output should be 0.5..
For the scenario when 'k' is 4, and 'arr' contains gas station positions {1, 2, 3, 4, 5}, one of the possible ways to place 4 new gas stations is as follows: {1, 1.5, 2, 2.5, 3, 3.5, 4, 4.5, 5}. This placement ensures that the maximum difference between adjacent gas stations is 0.5.
Therefore, the value of 'dist' in this case is 0.5, which represents the minimum possible maximum distance between gas stations after adding 4 new stations. It can be demonstrated that there is no feasible way to add 4 gas stations while achieving a lower value of 'dist' than 0.5.
Example 2:
Input :
For k=1 and arr = {1,2,3,4,5,6,7,8,9,10}
Output :
The output should be 1..
This scenario involves 'k' equal to 1 and an array 'arr' containing gas station positions {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}.
One of the possible ways to place 1 new gas station is as follows: {1, 1.5, 2, 3, 4, 5, 6, 7, 8, 9, 10}. In this placement, the maximum difference between adjacent gas stations remains 1.
As a result, the value of 'dist' in this case is indeed 1. It can be demonstrated that there is no feasible way to add 1 gas station while achieving a lower value of 'dist' than 1. Therefore, the correct answer for this scenario is indeed 1.
Understanding the Problem
The problem at hand involves optimizing the placement of gas stations to minimize the maximum distance between two consecutive stations. Given an array of existing gas stations and a number 'k' representing the additional stations to be added, we aim to determine the minimum possible maximum distance ('dist') achievable. This article will explore the concept and strategy for placing these new gas stations effectively.
To comprehend the problem, consider a small example with gas stations {1, 7} and k = 2. Initially, the maximum distance between stations is 6 (between 1 and 7). We want to add 2 new stations while minimizing the maximum distance.
Observation:
- Placing new stations before the first existing station or after the last existing station does not alter the maximum distance between stations.
- To minimize the maximum distance, new gas stations must be placed in between the existing stations.
Optimizing Station Placement:
To minimize the maximum distance between gas stations, we need to insert new stations with equal spacing. The formula to determine the spacing between consecutive stations is as follows:
Spacing = (section_length / (k + 1))
Here, 'section_length' represents the distance between the first and last existing stations.
Illustration:
Using the example of gas stations {1, 7} and k = 2:
- Initial 'dist' = (7 - 1) = 6.
- Spacing = 6 / (2 + 1) = 2.
- Optimal placement: {1, 3, 5, 7}.
Minimizing the maximum distance between gas stations involves strategically placing new stations between existing ones with uniform spacing. This approach ensures an efficient distribution of gas stations while reducing the 'dist' to the minimum possible value.
By following this strategy, we can solve the problem of minimizing the maximum distance between gas stations efficiently and effectively.
Approach 1 : Brute Force
In this section of this OpenGenus.org article, we will outline a naive approach to solving the problem of minimizing the maximum distance ('dist') between gas stations.
Given a sorted array 'arr' representing the positions of existing gas stations on the X-axis and an integer 'k' denoting the number of new gas stations to be added, our objective is to find the minimum 'dist' achievable by strategically placing the new gas stations.
Naive Approach
The naive approach is based on the idea of iteratively inserting 'k' new gas stations into sections between existing gas stations to reduce the maximum distance. Here are the steps involved in this approach:
Step 1: Initialize an array 'howMany[]' of size 'n-1' to keep track of the number of gas stations placed in each section between existing gas stations.
Step 2: Use a loop to pick 'k' gas stations one at a time for placement.
Step 3: Within another loop, identify the index 'i' where the distance ('arr[i+1] - arr[i]') is the maximum. Insert the current gas station between 'arr[i]' and 'arr[i+1]' by incrementing 'howMany[i]' to keep track of stations in that section.
Step 4: After placing all the new stations, find the distance between two consecutive gas stations for each section. The distance for a particular section can be calculated as follows:
Distance = (arr[i+1] - arr[i]) / (howMany[i] + 1)
Step 5: Among all the calculated distances, the maximum one will be the answer ('dist').
Algorithm
The algorithm for the naive approach is as follows:
- Initialize 'howMany[]' array of size 'n-1' to track stations per section.
- Loop 'k' times to pick 'k' gas stations.
- Within each loop iteration, find the index 'i' with the maximum distance ('arr[i+1] - arr[i]') and increment 'howMany[i]'.
- After placing all new stations, find the maximum distance ('dist') using the formula above.
The naive approach provides a straightforward way to minimize the maximum distance between gas stations by iteratively inserting new stations into sections with the maximum distances. However, it may not be the most efficient solution for large input sizes. More optimized approaches, such as binary search or heap-based solutions, may be preferred for larger datasets to reduce time complexity.
CODE:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
long double minimiseMaxDistance(vector<int> &arr, int k){
int n = arr.size();
vector<int> howMany(n-1,0);
for(int i = 1 ; i <= k; i++)
{
long double maxSection = -1;
int maxInd = -1;
for(int j = 0 ; j < n-1 ; j++)
{
long double diff = arr[j+1] - arr[j];
long double sectionLength = diff / (long double)(howMany[j]+1);
if(sectionLength > maxSection)
{
maxSection = sectionLength;
maxInd = j;
}
}
howMany[maxInd]++;
}
long double ans = -1;
for(int i = 0 ; i < n-1 ; i++)
{
long double diff = arr[i+1] - arr[i];
long double sectionLength = diff / (long double)(howMany[i]+1);
ans = max(ans, sectionLength);
}
return ans;
}
int main() {
int n, k;
cout << "Enter the number of elements in the array: ";
cin >> n;
vector<int> arr(n);
cout << "Enter the elements of the array: ";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
cout << "Enter the value of k: ";
cin >> k;
long double result = minimiseMaxDistance(arr, k);
cout << "The minimum maximum distance after " << k << " moves is: " << result << endl;
return 0;
}
Code Explanation:
The function minimiseMaxDistance takes 'arr' (existing gas station positions) and 'k' (number of new stations) as inputs.
Initializes 'howMany', a vector of size 'n-1' to track the number of stations in each section between existing stations.
A loop places 'k' new stations one by one.
Inside this loop, 'maxSection' and 'maxInd' are initialized to -1, used for tracking the maximum section length and its index.
Another loop identifies the section with the maximum distance by calculating 'sectionLength' for each section.
'sectionLength' is determined by dividing the difference in positions ('diff') by the number of stations in that section plus one.
If 'sectionLength' exceeds 'maxSection', 'maxSection' and 'maxInd' are updated with the current section's values.
After the inner loop, the code increments the station count in the section with the maximum distance by updating 'howMany[maxInd]'.
Finally, We initialize 'ans' to -1 and loops again to calculate the actual maximum distance ('ans') among all sections. The maximum 'ans' represents the minimum possible maximum distance between gas stations after placing 'k' new stations, and it is returned as the result.
Sample input and output:
Time and Space Complexities :
Time Complexity:
-
The outer loop runs 'k' times, where 'k' is the number of new gas stations to be placed.
-
Inside the outer loop, there is an inner loop that iterates over the 'n-1' sections between existing gas stations.
-
In each iteration of the inner loop, the code performs constant time calculations to find the section length and update 'maxSection' and 'maxInd'.
-
After placing all 'k' new stations, another loop iterates over the 'n-1' sections to calculate the maximum distance ('ans').
-
The overall time complexity is O(k * (n-1)), where 'k' is the number of new stations and 'n' is the number of existing gas stations. In practice, if 'k' is significantly smaller than 'n', this can be approximated as O(k * n).
Space Complexity:
-
The code uses additional space to store the 'howMany' vector of size 'n-1' to keep track of the number of stations in each section.
-
It also uses a few variables for calculations (e.g., 'maxSection', 'maxInd', 'ans').
-
The space complexity is primarily determined by the 'howMany' vector, which has a space complexity of O(n-1).
Overall, the code has a time complexity of approximately O(k * n) and a space complexity of O(n-1). The time complexity can be efficient for relatively small 'k' compared to 'n', but it may become less efficient for larger input sizes.
Approach 2 : Heap Based Solution
In this section, we present a better approach to solving the problem of minimizing the maximum distance ('dist') between gas stations. We leverage the priority queue (heap) data structure to efficiently identify and place new gas stations in sections that maximize distance reduction. This approach significantly improves the performance of the algorithm compared to the naive approach.
Improved Approach Using Heap
The improved approach employs a priority queue (heap), specifically a max heap implementation, to efficiently search for the section with the maximum distance between gas stations. The elements in the priority queue are represented as pairs <distance, index>, allowing us to keep track of distances and their corresponding indices sorted by distance.
Algorithm
The algorithm for the improved approach is as follows:
-
Initialize an array 'howMany[]' of size 'n-1' to track the number of gas stations placed in each section between existing gas stations.
-
Create a priority queue (max heap) to store pairs <distance, index>, where 'distance' represents the distance between adjacent gas stations and 'index' is the index of the section.
-
Insert the first 'n-1' indices into the priority queue along with their respective distances, which are calculated as 'arr[i+1] - arr[i]' for each index.
-
Enter a loop to place 'k' new gas stations one at a time.
-
In each iteration, pop the first element from the priority queue, as it represents the section with the maximum distance ('maxSection'). Let's denote its index as 'secInd'.
-
Place the current gas station at index 'secInd' by incrementing 'howMany[secInd]' to track the number of stations in that section.
-
Calculate the new section length ('new_section_length') after placing the gas station. It is calculated as follows:
new_section_length = initial_section_length / (number_of_stations_inserted + 1)
= (arr[secInd+1] - arr[secInd]) / (howMany[secInd] + 1)
-
Insert the pair <new_section_length, secInd> back into the priority queue for further consideration.
-
After placing all 'k' new stations, the distance at the top of the priority queue will represent the minimum possible maximum distance ('dist') between gas stations. This value is returned as the answer.
The improved approach, utilizing a priority queue (max heap), streamlines the search for the maximum distance between gas stations. It efficiently identifies sections with the greatest potential for distance reduction and optimally places new gas stations. This leads to a more efficient and faster solution compared to the naive approach.
CODE :
#include<bits/stdc++.h>
long double minimiseMaxDistance(vector<int> &arr, int k){
int n = arr.size();
vector<int>howMany(n-1,0);
priority_queue<pair<long double,int>>pq;
for(int i = 0 ; i < n-1 ; i++)
{
pq.push({arr[i+1]-arr[i] , i});
}
for(int i = 1 ; i <= k; i++)
{
auto it = pq.top();
pq.pop();
int ind = it.second;
howMany[ind]++;
long double diff = arr[ind+1]-arr[ind];
long double sectionLength = diff / (long double)(howMany[ind]+1);
pq.push({sectionLength,ind});
}
return pq.top().first;
}
int main() {
int n, k;
cout << "Enter the number of elements in the array: ";
cin >> n;
vector<int> arr(n);
cout << "Enter the elements of the array: ";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
cout << "Enter the value of k: ";
cin >> k;
long double result = minimiseMaxDistance(arr, k);
cout << "The minimum maximum distance after " << k << " moves is: " << result << endl;
return 0;
}
Code Explanation :
The function minimiseMaxDistance takes 'arr' (existing gas station positions) and 'k' (number of new stations) as inputs.
Initializes 'howMany', a vector of size 'n-1' to track the number of stations in each section between existing gas stations.
A priority queue ('pq') is initialized to store pairs <distance, index>. This priority queue is a max heap, meaning the maximum distance will always be at the top.
A loop initializes the priority queue with the distances between adjacent gas stations ('arr[i+1] - arr[i]') and their corresponding indices.
Another loop is used to place 'k' new gas stations.
In each iteration, the code retrieves the element with the maximum distance from the priority queue ('pq.top()') and stores it in 'it'. The index of the section with the maximum distance is extracted as 'ind'.
The number of stations in the section with the maximum distance ('howMany[ind]') is incremented.
We calculate the new section length ('sectionLength') after placing the gas station using the formula mentioned in the problem statement.
The priority queue is updated with the new section length and index.
After placing all 'k' new stations, the maximum distance ('dist') between gas stations can be found at the top of the priority queue, and it is returned as the result.
Sample input and output:
Time and Space Complexities :
Time Complexity:
-
Initializing the priority queue with distances between adjacent gas stations takes O(n-1 * log(n-1)) time, as each insertion into the priority queue (heap) takes O(log(n-1)) time, and this operation is performed for 'n-1' sections.
-
The loop to place 'k' new gas stations involves 'k' iterations. In each iteration, we perform the following operations:
- Extracting the maximum element from the priority queue ('pq.top()') takes O(log(n-1)) time.
- Incrementing 'howMany[ind]' takes constant time.
- Calculating the new section length ('sectionLength') takes constant time.
- Inserting the new section length and index back into the priority queue takes O(log(n-1)) time.
-
The overall time complexity of the loop to place 'k' stations is O(k * log(n-1)), where 'k' is the number of new stations and 'n-1' is the number of sections.
-
Finally, extracting the maximum distance ('dist') from the priority queue takes O(log(n-1)) time.
The dominant time complexity is the loop to place 'k' stations, making the overall time complexity approximately O(k * log(n-1)).
Space Complexity:
-
The space complexity is primarily determined by the 'howMany' vector, which has a size of 'n-1'. Therefore, the space complexity for the 'howMany' vector is O(n-1).
-
The space used by the priority queue ('pq') also depends on the number of sections ('n-1') and the size of the 'pair' elements.
-
Overall, the space complexity is determined by the 'howMany' vector and the priority queue, which both depend on 'n-1'. Therefore, the space complexity is O(n-1).
In summary, Our approach has a time complexity of approximately O(k * log(n-1)) and a space complexity of O(n-1). This approach efficiently utilizes a priority queue (heap) to optimize the search for the maximum distance between gas stations.
Approach 3 : Binary Search
Our approach utilizes the Binary Search algorithm to efficiently tackle the problem of minimizing the maximum distance ('dist') between gas stations. This optimized approach is tailored for decimal answer spaces and ensures accurate results.
Key Observations:
-
Minimum and Maximum Possible Answers:
- The minimum possible answer is achieved when all gas stations are placed at a single location, resulting in a maximum distance of 0.
- The maximum possible answer is the maximum distance between two consecutive existing gas stations.
-
Sorted Answer Space:
- Our answer space is sorted, and we can observe a pattern that allows us to divide it into two halves: potential answers and non-viable options.
Binary Search Modifications for Decimal Answer Space:
We need to make adjustments to the traditional Binary Search algorithm to handle a decimal answer space effectively:
-
While Loop Condition: Instead of 'while(low <= high)', we use 'while(high - low > 10^(-6))' to consider differences up to the 6th decimal place, as specified in the problem.
-
Low and High Updates:
- We use 'low = mid' instead of 'low = mid+1' to avoid skipping decimal values in the left half.
- Similarly, we use 'high = mid' instead of 'high = mid-1' to ensure we don't miss potential answers in the right half.
Calculating the Number of Gas Stations for a Given Distance ('dist'):
We introduce a function, 'numberOfGasStationsRequired(dist, arr[])', to determine the number of gas stations we can place for a specific distance 'dist'. The process involves iterating through sections between gas stations and calculating the number of stations needed based on the section length. We also handle an edge case where the section length is completely divisible by 'dist'.
Algorithm Overview:
-
Find the maximum distance between two consecutive gas stations, denoted as 'max(dist)'.
-
Initialize two pointers, 'low' and 'high', initially set to 0 and 'max(dist)'.
-
Utilize a 'while' loop with the condition 'while(high - low > 10^(-6))' to narrow down the answer space.
-
Calculate the 'mid' value as '(low+high) / 2.0'.
-
Eliminate halves based on the number of gas stations returned by 'numberOfGasStationsRequired(mid, arr[])':
- If 'result > k', eliminate the left half by setting 'low = mid'.
- Otherwise, 'mid' is a possible answer, so eliminate the right half by setting 'high = mid'.
-
Outside the loop, return either 'low' or 'high' as their difference is within the specified precision of 10^(-6). Both values are potential answers, and we return 'high' in this case.
Our approach efficiently applies Binary Search to find the minimum possible maximum distance between gas stations while considering the precision required by the problem statement. This ensures accurate and optimized results without revealing the source of the approach.
CODE :
long double minimiseMaxDistance(vector<int> &arr, int k){
int n = arr.size();
long double low = 0;
long double high = 0;
for(int i = 0 ; i < n-1 ; i++)
{
high = max(high , (long double)(arr[i+1]-arr[i]));
}
while(high - low > 1e-6)
{
long double mid = (low+high)/(2.0);
int cnt = gasStations(mid,arr);
if(cnt <= k)
{
high = mid;
}
else
{
low = mid;
}
}
return high;
}
int main() {
int n, k;
cout << "Enter the number of elements in the array: ";
cin >> n;
vector<int> arr(n);
cout << "Enter the elements of the array: ";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
cout << "Enter the value of k: ";
cin >> k;
long double result = minimiseMaxDistance(arr, k);
cout << "The minimum maximum distance after " << k << " moves is: " << result << endl;
return 0;
}
Code Explanation :
The function minimiseMaxDistance takes 'arr' (existing gas station positions) and 'k' (number of new stations) as inputs.
We initialize 'low' and 'high' as 0. 'low' represents the lower bound of the answer space, and 'high' represents the upper bound.
In a loop, we calculate the 'high' value by finding the maximum distance between consecutive existing gas stations ('arr[i+1] - arr[i]').
We enter a Binary Search loop to find the minimum maximum distance ('dist').
Inside the Binary Search loop:
We calculate 'mid' as the average of 'low' and 'high'.
We call a helper function 'gasStations(mid, arr)' to determine the number of gas stations that can be placed with the current 'mid' distance. The result is stored in 'cnt'.
If 'cnt' (the number of gas stations needed) is less than or equal to 'k' (the number of new stations we can place), it means we can potentially reduce the maximum distance. So, we update 'high' to 'mid' to narrow down the search to the left half of the answer space.
If 'cnt' is greater than 'k', it means we need to increase the maximum distance to accommodate all the gas stations. So, we update 'low' to 'mid' to narrow down the search to the right half of the answer space.
The Binary Search loop continues until the difference between 'high' and 'low' is less than or equal to 1e-6, which is the specified precision in the problem statement.
Finally, we return 'high' as the minimum maximum distance that allows placing 'k' new gas stations while maintaining the specified precision.
Explanation of helper function :
CODE :
int gasStations(long double dist , vector<int> &arr)
{
int cnt = 0;
for(int i = 0 ; i < arr.size()-1;i++)
{
int between = (arr[i+1]-arr[i])/dist;
if((arr[i+1]-arr[i]) == between*dist)
{
between--;
}
cnt += between;
}
return cnt;
}
Code Explanation :
The gasStations function takes two parameters: 'dist' (the distance between gas stations to be considered) and 'arr' (a vector containing the positions of existing gas stations).
We initialize a variable 'cnt' to keep track of the total number of gas stations needed to cover the given distance.
We enter a loop that iterates through the elements of the 'arr' vector, which represents the positions of existing gas stations.
Inside the loop:
We calculate the variable 'between', which represents how many gas stations can fit in the current section between two consecutive existing gas stations. This is determined by dividing the length of the current section ('arr[i+1] - arr[i]') by the specified distance 'dist'.
We check for an edge case where the section length is exactly divisible by 'dist'. In this case, we reduce the count in 'between' by 1, as one less station is needed to cover the section entirely.
The value in 'between' represents the number of gas stations required for the current section, and we add it to the running total 'cnt'.
After processing all sections between existing gas stations, the function returns the total count of gas stations needed to cover the given distance with the specified distance between stations.
Sample input and output:
Time and Space Complexities :
Time Complexity:
-
Calculating 'high': O(n), where 'n' is the number of existing gas stations. We iterate through the gas stations once to find the maximum distance between two consecutive stations.
-
Binary Search: O(log(HIGH-LOW)), where 'HIGH' is the maximum possible distance between gas stations (initially set to 'high') and 'LOW' is 0. The binary search runs in logarithmic time as we repeatedly divide the search space into halves.
-
Inside the binary search loop, calling 'gasStations(mid, arr)' takes O(n) time, as we iterate through 'arr' to calculate the number of gas stations for a given distance 'mid'. However, the total complexity of this step is still O(log(HIGH-LOW) * n) because it is performed within the binary search loop.
The overall time complexity is O(n + n * log(HIGH-LOW)), which can be simplified to O(n * log(HIGH-LOW)).
Space Complexity:
- The space complexity is O(1) as we use constant space, except for a few variables (e.g., 'low', 'high', 'mid', and 'dist').
Comparative Analysis of Approaches:
Brute Force Approach:
Time Complexity: O(k * n) in the worst case, where 'k' is the number of new gas stations and 'n' is the number of existing gas stations.
Space Complexity: O(n-1) for the 'howMany' vector.
Heap-Based Approach:
Time Complexity: O(k * log(n-1)) in the worst case, where 'k' is the number of new gas stations and 'n' is the number of existing gas stations.
Space Complexity: O(n-1) for the 'howMany' vector and the priority queue.
Binary Search Approach:
Time Complexity: O(n * log(HIGH-LOW)), where 'n' is the number of existing gas stations, and 'HIGH' and 'LOW' represent the upper and lower bounds of the answer space, respectively.
Space Complexity: O(1) for constant space usage.
In summary, the binary search approach is the most efficient solution for this problem, providing accurate and optimized results within the specified precision. It leverages the properties of the problem and the binary search algorithm to achieve optimal performance.