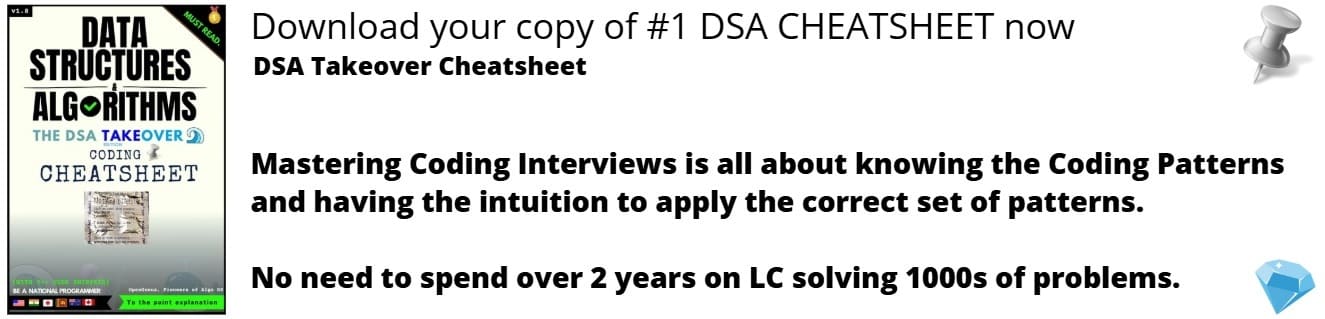
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented how to print boolean values in C++ (as integer and text) and explored the concepts of std::boolalpha and std::noboolalpha.
Table of contents:
- Print boolean in C++ by default
- std::boolalpha
- std::noboolalpha
- Conclusion
Print boolean in C++ by default
Boolean values are interpreted by cout in C++ as integers. As true is mapped to 1 and false is mapped to 0. This is by default and can be changed.
Following C++ code illustrates the concept:
#include <iostream>
int main()
{
std::cout << false << std::endl;
std::cout << true << std::endl;
}
Output:
0
1
std::boolalpha
std::boolalpha is an I/O manipulator that can be passed to cout to change the way boolean values are interpreted by cout.
When std::boolalpha is passed to cout, boolean values are treated as string values. true is interpreted as "true" and false is interpreted as "false".
std::cout << std::boolalpha << true << std::endl;
If we set the following:
std::cout << std::boolalpha;
Then, all cout following the above line will consider that boolaplha is already set. To revert to the original behavior, one need to set:
std::cout << std::noboolalpha;
Following C++ code illustrates the concept:
#include <iostream>
int main()
{
std::cout << std::boolalpha << false << std::endl;
std::cout << std::boolalpha << true << std::endl;
}
Output:
0
1
std::noboolalpha
Similar to std::boolalpha, std::noboolalpha is an I/O manipulator that enables cout to treat boolean values as integer values. This is the default behaviour.
std::cout << std::noboolalpha << false << std::endl;
Following C++ code illustrates the concept:
#include <iostream>
int main()
{
std::cout << std::noboolalpha << false << std::endl;
std::cout << std::noboolalpha << true << std::endl;
}
Output:
0
1
Conclusion
Following C++ code summarizes the idea of printing boolean using cout:
#include <iostream>
int main()
{
std::cout << false << std::endl;
std::cout << std::boolalpha << false << std::endl;
std::cout << true << std::endl;
std::cout << std::boolalpha << true << std::endl;
std::cout << std::noboolalpha << true << std::endl;
std::cout << std::noboolalpha << false << std::endl;
}
Output:
0
false
1
true
1
0
With this article at OpenGenus, you must have the complete idea of how to print boolean in C++.