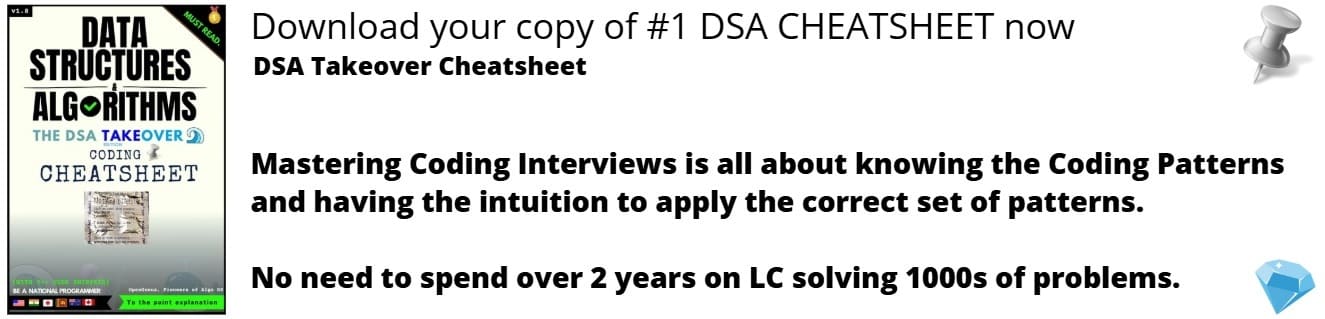
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to read and write from a CSV file in C++ Programming Language. We have illustrated the process using C++ code examples.
Contents
- CSV
- Why CSV File is used?
- Write in CSV File
- Example of writing data to a CSV File
- Read in CSV File
- Example of Reading data from a CSV File
- Optimize the performance of reading and writing large CSV files
- handling errors and exceptions when reading or writing to a CSV file
1.What is CSV?
CSV stands for Comma Separated Values.
CSV is a simple file format used to store tabular data i.e. data that is organized into rows and columns such as a spreadsheet or a database.
The data fields in a CSV file are separated by a comma (', ') and the individual rows are separated by a newline (‘\n’).
In C++, reading and writing to a CSV file can be achieved using the standard input/output libraries (iostream) and the fstream library.
In a CSV file, each line represents a record and the values within a record are separated by commas. For example, the following CSV file represents a list of people with their names, ages, and addresses:
Name, Age, Address
John Doe, 32, 123 Main Street
Jane Doe, 29, 456 Elm Street
2.Why CSV File is used?
CSV files are widely used because they are simple, lightweight, and easily readable by both humans and computers. They are also compatible with a variety of software applications, including spreadsheets, databases, and programming languages. Additionally, CSV files can be imported and exported between different software programs, making it easy to transfer data between applications.
Another advantage of CSV files is their ability to store large amounts of data in an organized manner. This makes them ideal for storing and sharing large datasets, such as customer data, sales data, and financial data.
3. Write in CSV File
To write to a CSV file, we first need to create a file object and open the file in write mode using the ofstream object. Then, we can write data to the file using the << operator. We should separate the values with a comma to ensure that the values are stored in separate columns. Finally, We should close the file using the close() method.
4. Example of writing data to a CSV File:-
Here's an example of how to write data to a CSV file in C++:
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ofstream file;
file.open("example.csv");
file << "Name, Age, Gender" << endl;
file << "John, 30, Male" << endl;
file << "Jane, 25, Female" << endl;
file.close();
return 0;
}
5. Read in CSV File
To read from a CSV file, we first need to create a file object and open the file in read mode using the ifstream object. Then, we can use the getline() method to read the contents of the file line by line. Finally, we should close the file using the close() method.
since whatever data reading from the file, is stored in string format, so always convert string to the required datatype before comparing or calculating, etc.
6. Example of reading data from a CSV File
Here's an example of how to read data from a CSV file in C++:
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream file;
file.open("example.csv");
string line;
getline(file, line);
while (getline(file, line)) {
cout << line << endl;
}
file.close();
return 0;
}
7. Optimize the performance of reading and writing large CSV files
optimizing the performance of reading and writing large CSV files can be achieved in many ways:-
- Buffered I/O:
Instead of reading or writing one character at a time, use buffered I/O by reading or writing a block of data at once. - Parallel Processing:
We can use parallel processing to split a large CSV file into smaller chunks and process each chunk concurrently, which can significantly improve performance. This can be done using threads, OpenMP, or other parallel processing frameworks. - Compression:
Compressing the data before writing it to a file can significantly reduce the size of the file and reduce the time required to read and write it. - Data Formatting:
The format of the data can greatly impact performance when reading and writing large CSV files.
8. handling errors and exceptions when reading  or writing to a CSV file
Handling errors and exceptions when reading or writing to a CSV file is important to ensure that the data is read and written correctly and to prevent unexpected behavior.
When reading a CSV file, some common errors and exceptions that may occur include :-
1. File Not Found:
If the file being read cannot be found, an error or exception can be thrown. This can be handled by using try-catch blocks to catch the error and handle it gracefully.
2. Invalid Format:
If the CSV file is in an invalid format, an error or exception may occur when trying to parse it. This can be handled by checking the format of the file before parsing it.
3. Data Validation:
When reading data from a CSV file, it is important to validate the data to ensure that it is in the correct format and contains the expected values. If data validation fails, an error or exception can be thrown.
When writing to a CSV file, some common errors and exceptions that may occur include :-
1.File Not Found:
If the file being written to cannot be found, an error or exception can be thrown. This can be handled by using try-catch blocks to catch the error and handle it gracefully.
2.File Permission Denied:
If the file being written to does not have the correct permissions, an error or exception may occur. This can be handled by checking the file permissions before writing to it.
3. Disk Space:
If there is not enough disk space to write the data, an error or exception may occur. This can be handled by checking the available disk space before writing to the file.
With this article at OpenGenus, you must have the complete idea of working with CSV files in C++.