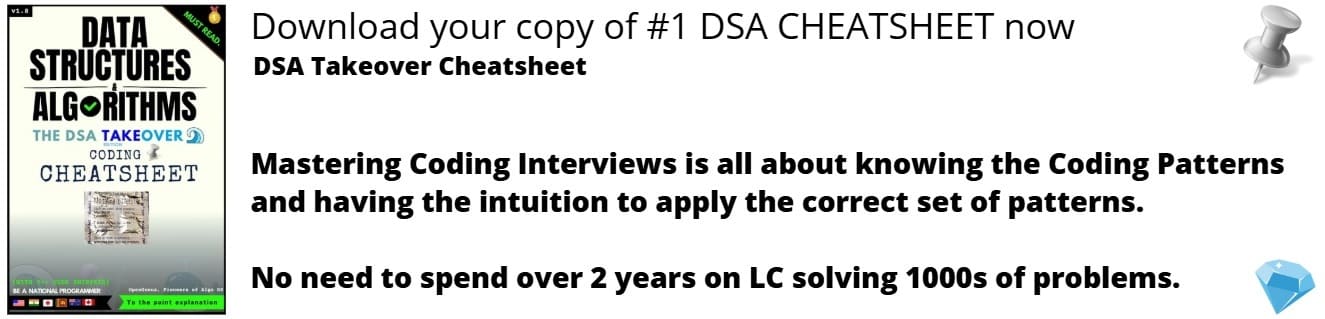
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered 6 different approaches to reverse a string in C++ Programming Language.
Reversing a string is basic question used as a base for many medium and hard levels questions aked in interviews to understand basic level understanding of a candidate.
There are many methods to so ,we will be understanding all those methods one by one.
Table of contents / Different ways to reverse a string in C++:
- Method 1: Swapping individual characters of a string
- Method 2: Using prebuilt functions
- Method 3: Using an extra space or array to store
- Method 4: Pointer approach
- Method 5: Using stack data structure
- Method 6: Using Vector data structure
Method 1: Swapping individual characters of a string
In this method, we are swapping the characters of a string with its length-(end).
This method is the most basic method for reversing a string as it does not involves any complex logic.
Algorithm-
- Firstly, we calculate the length of the string as we just need to travel till only the half of length of string.
- We are replacing character at i'th position wth character at n-i-1'th positon.
So,Complexity Analysis-
- Time Complexity: O(N)
- Auxiliary Space: O(1)
Code
Below is the code for more clear understading of the algorithm.
#include<bits/stdc++.h>
using namespace std;
void revstring(char* str)
{
int temp;
int len = strlen(str);
for(i = 0;i < len/2;i++)
{
//swapping the variable with len-1
temp = str[i];
str[i] = str[len - i - 1];
str[len - i - 1] = temp;
}
}
int main()
{
char str[10] = "racing";
cout << "Original string: " << str;
revstring(str);
cout<< "\nReversed string: " << str;
return 0;
}
Output-
Original string: racing
Reversed string: gnicar
Method 2: Using prebuilt functions
In this method we are using prebuilt functions that help in reversing the ,these are prebuilt to help in development by reducing the time taken to apply the approach so it is a good way to learn these also.
We can use reverse which is defined under the header file algorithm, #include<algorithm>
where str.begin() denotes the start and str.end() denotes end.
#include<bits/stdc++.h>
includes all the files in the CPP.
Algorithm-
- In this approach we are directly using the predefined method,in which we are required the begining of string and the ned of string.
Below is the code explanation ,if you want to know in depth about the can check out the docmentation of any CPP.
So, Complexity Analysis-
- Time Complexity: O(N)
- Auxiliary Space: O(1)
Code-
#include<bits/stdc++.h>
using namespace std;
int main()
{
string str = "racing";
cout << "Original String: " << str;
reverse(str.begin(), str.end());//inbuilt function
cout << "\nReversed String: "<< str;
return 0;
}
Output-
Original string: racing
Reversed string: gnicar
Method 3: Using an extra space or array to store
In this method we use a extra space to solve our problem although it increase the space complexity but it reduces the stress to find a complex solution with lower space complexity.
The method is to initialize a array of the equal size and start copying the elements of the input string from the end.
This is the most simplest method to try out as all we need is size of array.
Algorithm-
- We initialize the another array of same size of array tostore the reversed characters of original array.
- Intialize an variable with length of array.
- Now we traverse the whole array and store characters from the last of array in reverse by using the created variable and derementing it.
So, Complexity Analysis-
- Time Complexity: O(N)
- Auxiliary Space: O(N)
#include<bits/stdc++.h>
using namespace std;
int main() {
char str[10] = "racing", rev[10];
int len = strlen(str);
cout << "Original String: " << str;
int j = len - 1;
// Storing into another array
for (i = 0; i <= len; i++) {
rev[i] = str[j];
j--;
}
cout << "\nReversed String: " << rev;
return 0;
}
Output-
Original string: racing
Reversed string: gnicar
Method 4: Pointer approach
Pointers are basically symbolic representation of the addresses.It stimulates call by reference.
A pointer of type integer can hold the address of a variable of type integer. A pointer of character type can hold the address of a variable of character type. So, it tells type of variablechanges the type of pointer.
Algorithm-
- In this approach we put the pointer at last of the string character then reversing it on by one with the starting character .
- In the below code we are simple printing the characters by placing pointer at the last character.
Complexity Analysis-
- Time Complexity: O(N)
- Auxiliary Space: O(N)
Code-
#include<bits/stdc++.h>
using namespace std;
int main() {
char str[] = "racing";
cout << "Original string: " << str;
cout << endl << "Reversed String: ";
for(int i = (strlen(str) - 1); i >= 0; i--)
cout << str[i];
return 0;
}
Output-
Original string: racing
Reversed string: gnicar
Method 5: Using stack data structure
Stack are containers that work on LIFO(Last in first out) principle.
LIFO works as a new element is added at one end (top) and an element is removed from that end only.
Stack uses encapsulated object of vector or deque,providing acess to specific set of functions.It usues deque by default.
Algorithm-
- We create a empty stack and push the characters of the string in the stack from starting.
- Due the property of stack of LIFO we input the characters from start and remove from last.So, we just use the pop() function to reversethe string.
So,Complexity Analysis-
- Time Complexity: O(N)
- Auxiliary Space: O(N)
Code-
#include <bits/stdc++.h>
using namespace std;
int main()
{
string str = "racing";
stack<char> st;
for (char i : str)
{
st.push(i);
}
cout << "Original string: " << str;
cout << endl << "Reversed String: ";
while (!st.empty()) {
cout << st.top();
st.pop();
}
return 0;
}
Output-
Original string: racing
Reversed string: gnicar
Method 6: Using Vector data structure
One of the most useful tool of c++ that helps to solve complex problems is STL(standard template library) and one of its tools is vector.
Vectors are sequence containers that represent arrays that can change sizes.
Algorithm-
- We create an empty vector as vector is a dynamic array.
- Now just push the characters from the last of string in the vector.
- At last we just print the reversed string stored in the vector.
-->Below is the code explanation.
So, Complexity Analysis-
- Time Complexity: O(N)
- Auxiliary Space: O(N)
Code-
#include <bits/stdc++.h>
using namespace std;
int main()
{
string str = "racing";
int n=str.length();
vector<char> v;
for (int i = n - 1; i >= 0; i--)
{
v.push_back(str[i]);
}
cout << "Original string: " << str;
cout << endl << "Reversed String: ";
for(auto i:v){
cout<<i;
}
return 0;
}
Output-
Original string: racing
Reversed string: gnicar
With this article at OpenGenus, you must have the complete idea of different ways to reverse a string in C++.