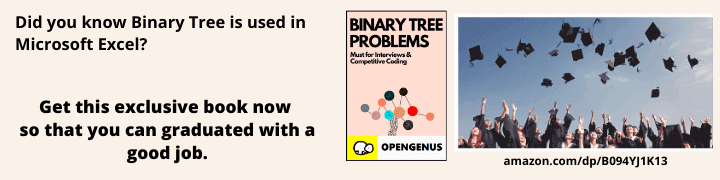
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
System Design for Elevator System
Understanding the problem (Elevator system)
Elevator system is a system that is used to move people from one floor to another floor in a building. Users must be able to request an elevator to a particular floor and the elevator must be able to move people from one floor to another floor in a given time with minimum number of stops.
The elevator system must also be able to handle multiple users and elevators in a building.
System Requirements
The key requirements that need to be offered by an elevator system can be classified into functional and non-functional requirements.
Functional Requirements
Functional Requirements describe the service that the system must offer. The functional requirements of an elevator system are as follows:
- The elevator car can have three states: moving up, moving down, and idle.
- The elevator must be able to move people from one floor to another floor with minimum number of stops.
- The elevator can only open its doors when it is idle.
- Users must be able to request an elevator to a particular floor.
- Minimal power consumption by the elevator system is also desired as minimum power consumption means less electricity bill.
- Operational zones of the elevator system must be defined as the system has multiple elevators and multiple floors. Zones are defined as a set of floors that are serviced by a particular elevator. Defining operational zones will help in reducing the number of stops made by the elevator when it comes to larger buildings with higher number of floors.
Non-Functional Requirements
Non-Functional Requirements describe the quality of the service that the system must offer. For the elevator system, the most important non-functional requirements include performance, reliability, security and emergency handling.
Performance
Performance is the most important non-functional requirement for an elevator system. The elevator system must be able to handle a large number of requests from users. The elevator system must be able to move people from one floor to another floor in a given time with minimum number of stops. The elevator system must also be able to handle multiple users and elevators in a building.
Reliability
Reliability is the ability of the system to perform its required functions under stated conditions for a specified period of time. The elevator system is said to be reliable if it is able to handle a large number of requests from users.
Security
Security is the ability of the system to protect its data from unauthorized access. The data that needs to be protected in the elevator system includes the user's information and the elevator's information. The elevator system is said to be secure if it is able to handle a large number of requests from users and the data is protected from unauthorized access.
Emergency Handling
Emergency handling is the ability of the system to handle emergency situations. The elevator system is said to be able to handle emergency situations if it is able to handle a critical situation like a fire or a power outage and transport all the people to the nearest exit.
Estimation of Requirements
The elevator system must be able to handle multiple users and elevators in a building. This means that the elevator system must be able to handle a large number of requests from users.
Let us assume that the number of floors in a building is 200 and the number of elevators in a building is 50.
Elevator specs:
- Maximum capacity: 10 people
- Maximum speed: 1 floor per second
- Door open time: 20 seconds
- Door close time: 5 seconds
Estimation of number of requests
Assuming that the elevator system is used by 1000 people per day, the number of requests per day can be estimated as follows:
- Number of requests per day = 1000 * 8 = 8000 (multiply by 8, assuming that each person makes 8 requests per day.)
Storage Estimation
Assuming that each request is stored in 100 bytes, the storage requirements for the elevator system can be estimated as follows:
- Storage requirements = 8000 * 100 = 800000 bytes = 800 KB = 0.8 MB
- The storage requirements for the elevator system are very low as the number of requests per day is very low.
Compute Estimation
For the elevator system, the compute requirements are very high as the elevator system must be able to handle a large number of requests from users. The system must also be able to formulate an algorithm to decide the path of the elevator to minimize the number of stops made by the elevator. The compute requirements for the elevator system can be estimated as follows:
- Compute requirements = 8000 * 1 = 8000 requests per second
The number of processing cores required for the elevator system can be estimated as follows: - Number of processing cores = 8000 / 1000 = 8 processing cores
- Thus, the server must have at least 8 processing cores to handle the requests from users.
Data flow
- The type of database used for the elevator system is a relational database as the data is structured and the data is related to each other.
- Relational databases like MySQL, PostgreSQL, Oracle, etc. can be used for storing structured data.
- The following class diagram will help understanding the various components of the system and their relation:
The above class diagram shows the various components of the elevator system and their relation. The components of the elevator system are as follows: - Person: The person is the user of the elevator system.
- The person can request an elevator to a particular floor.
- The person can also press the floor stop button inside the elevator to request the elevator to stop at a particular floor.
- To call the elevator, the person can use the pressButton() method.
- Elevator: The elevator is the system that is used to move people from one floor to another floor in a building.
- The elevator can have three states: moving up, moving down, and idle.
- The next floor the elevator must visit can be processed by using the goTo() method.
- Elevator Controller: The elevator controller is the component that is used to control the elevator.
- The elevator controller is responsible for moving the elevator to the requested floor.
- The requestElevator() method is used to request the elevator to a particular floor.
- The addToStopFloors() method is used to add a floor to the stop floors list.
- The getNextStopFloors() method is used to get the next floors to stop the elevator.
- The clearAllRequests() method is used to clear all the requests from the elevator.
- The removeFromStopFloors() method is used to remove a floor from the stop floors list.
- The sufficientPeople() method returns true if the elevator has sufficient people to move to the next floor.
- Door: The door is the component that is used to open and close the elevator doors. The door can only open its doors when it is idle.
- The door can have two states: open and closed.
- The open() method is used to open the door.
- The open() method is used to close the door.
- The countPeopleEntering() method is used to count the number of people entering the elevator.
- The countPeopleExiting() method is used to count the number of people exiting the elevator.
- Floor Stop Button: This button is present inside the elevator. The user can press this button to request the elevator to stop at a particular floor.
- The stopFloor() method is used to stop the elevator at a particular floor.
- The addStopToQueue() method is used to add a floor to the stop floors queue.
- The removeStopFromQueue() method is used to remove a floor from the stop floors queue.
- The pressButton() method is used to press the floor stop button.
High Level Component Design
After analysing and deciding the requirements and the data flow, the next step is to describe the system architecture on a higher level.
The architecture style for the elevator system is a client-server architecture.
Client-Server Architecture
- The client-server architecture is a distributed architecture where the client and the server are connected over a network.
- The client sends requests to the server and the server responds to the client.
- The client-server architecture is used for the elevator system as the client is the user and the server is the elevator system.
- The user sends a request to the elevator by pressing a button on the elevator panel. The elevator system receives the request and processes it. The elevator system then sends a response to the user by moving the elevator to the requested floor.
Detailed Component Design
After deciding the architecture style for the elevator system, the next step is to describe the system architecture in detail.
User management
The user need not register to use the elevator system. The user can use the elevator system by pressing a button on the elevator panel.
Elevator status management
The status of the elevator must be dynamically updated in the database. The status of the elevator includes the current floor, the direction of the elevator, the number of people in the elevator, etc. The status of the elevator must be updated in the database for every stop made by the elevator.
Elevator request management
The request made for an elevator must also be dynamically updated in the database. The database must just store the floor number of the requested floor. The number of requests for each floor and the direction of request (up or down) must be used to decide an optimal path for the elevator with minimal stops.
Technology Stack
The technology stack for the elevator system is as follows:
- Programming language: Python
- Python is a general-purpose object-oriented programming language. Python can be used for writing the backend code for the elevator system.
- Database: MySQL
- MySQL is a relational database management system. MySQL is used for storing structured data.
Code Sample
A sample implementation of the elevator system using Python is as follows:
# Elevator System Design
# The component classes are as follows:
# Person: The person who is using the elevator
# ElevatorController: The controller for the elevator
# Door: The door of the elevator
# Elevator: The elevator itself
# FloorStopButton: The button on each floor to call the elevator
class Person:
def __init__(self, name, currentFloor, requestDirection):
self.name = name
self.currentFloor = currentFloor
self.requestDirection = requestDirection
# Function to request elevator
def pressButton(self, floorStopButton):
floorStopButton.press(self)
class ElevatorController:
def __init__(self, currentFloor, direction, stopFloorList):
self.currentFloor = currentFloor
self.direction = direction
self.stopFloorList = stopFloorList
# Function to move elevator
def requestElevator(self, floorStopButton):
floorStopButton.press(self)
# Function to add a floor to the stopFloorList
def addToStopFloors(self, floor):
self.stopFloorList.append(floor)
# Function to remove a floor from the stopFloorList
def removeFromStopFloors(self, floor):
self.stopFloorList.remove(floor)
# Function to get the next stop floor
def getNextStopFloor(self):
if self.direction == "up":
# Return minimum value in stopFloorList as the lift is going up
return min(self.stopFloorList)
else:
# Return maximum value in stopFloorList as the lift is going down
return max(self.stopFloorList)
# Function to clear all requests
def clearAllRequests(self):
self.stopFloorList = []
# Function to check if there are sufficient people to move the elevator
def sufficientPeople(self):
if len(self.stopFloorList) > 1:
return True
else:
return False
class Door:
# Function to open the door
def open(self):
self.doorState = "open"
# Function to close the door
def close(self):
self.doorState = "closed"
# Function to count the number of people entering the elevator
def countPeopleEntering(self):
self.peopleEntering = 0
for person in self.people:
if person.currentFloor == self.currentFloor:
self.peopleEntering += 1
return self.peopleEntering
# Function to count the number of people exiting the elevator
def countPeopleExiting(self):
self.peopleExiting = 0
for person in self.people:
if person.requestFloor == self.currentFloor:
self.peopleExiting += 1
return self.peopleExiting
class Elevator:
# Function to move the elevator to a specified floor
def goTo(self, floor):
self.currentFloor = floor
class FloorStopButton:
def stopFloor(self):
return self.floor
def addToStopQueue(self, elevatorController):
self.elevatorController = elevatorController
self.elevatorController.addToStopFloors(self.floor)
def removeFromStopQueue(self, elevatorController):
self.elevatorController = elevatorController
self.elevatorController.removeFromStopFloors(self.floor)
def press(self, person):
self.person = person
self.person.currentFloor = self.floor
self.addToStopQueue(self.elevatorController)
# Create a list of people
people = []
people.append(Person("Person 1", 1, "up"))
people.append(Person("Person 2", 2, "up"))
people.append(Person("Person 3", 3, "up"))
people.append(Person("Person 4", 4, "up"))
people.append(Person("Person 5", 5, "up"))
# Create a list of floor stop buttons
floorStopButtons = []
for i in range(1, 6):
floorStopButtons.append(FloorStopButton(i))
# Create an elevator controller
elevatorController = ElevatorController(1, "up", [])
# Create an elevator
elevator = Elevator(1)
# Create a door
door = Door()
# Create a list of people in the elevator
peopleInElevator = []
# Create a list of people who have exited the elevator
peopleExitedElevator = []
# Create a list of people who have entered the elevator
peopleEnteredElevator = []
# Create a list of people who have requested the elevator
peopleRequestedElevator = ["Person 1", "Person 4", "Person 5"]
# Implement the elevator system
while True:
# Check if there are any people who have requested the elevator
for person in people:
if person.currentFloor == elevator.currentFloor:
peopleRequestedElevator.append(person)
# Check if there are sufficient people to move the elevator
if elevatorController.sufficientPeople():
# Move the elevator to the next stop floor
elevator.goTo(elevatorController.getNextStopFloor())
# Open the door
door.open()
# Count the number of people entering the elevator
peopleEntering = door.countPeopleEntering()
# Count the number of people exiting the elevator
peopleExiting = door.countPeopleExiting()
# Add the people entering the elevator to the peopleInElevator list
for i in range(peopleEntering):
peopleInElevator.append(peopleRequestedElevator[i])
# Add the people exiting the elevator to the peopleExitedElevator list
for i in range(peopleExiting):
peopleExitedElevator.append(peopleInElevator[i])
# Remove the people exiting the elevator from the peopleInElevator list
for i in range(peopleExiting):
peopleInElevator.remove(peopleExitedElevator[i])
# Remove the people entering the elevator from the peopleRequestedElevator list
for i in range(peopleEntering):
peopleRequestedElevator.remove(peopleEnteredElevator[i])
# Close the door
door.close()
# Remove the floor from the stopFloorList
elevatorController.removeFromStopFloors(elevator.currentFloor)
else:
# Clear all requests
elevatorController.clearAllRequests()
# Check if there are any people who have requested the elevator
for person in people:
if person.currentFloor == elevator.currentFloor:
peopleRequestedElevator.append(person)
# Check if there are sufficient people to move the elevator
if elevatorController.sufficientPeople():
# Move the elevator to the next stop floor
elevator.goTo(elevatorController.getNextStopFloor())
# Open the door
door.open()
# Count the number of people entering the elevator
peopleEntering = door.countPeopleEntering()
# Count the number of people exiting the elevator
peopleExiting = door.countPeopleExiting()
# Add the people entering the elevator to the peopleInElevator list
for i in range(peopleEntering):
peopleInElevator.append(peopleRequestedElevator[i])
Identify and resolve bottlenecks
The bottlenecks in the design made so far are as follows:
- The elevator may not be able to handle a large number of requests from users.
- This can be resolved by using a load balancer to distribute the requests to multiple servers.
- Two or more elevators might respond to the same request.
- This can be resolved by using a distributed lock to ensure that only one elevator responds to a request.
With this article at OpenGenus, you must have got a strong idea about the System Design of an elevator system.