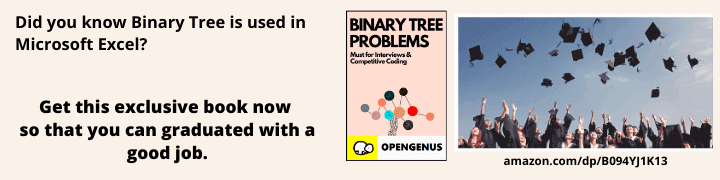
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have developed a command line tool to test typing speed for the user. We have implemented this in Java Programming Language. This is a strong addition to a SDE portfolio.
We'll discuss:
- Introduction
- Features
- Basic Idea of Implementation
- TypingTest Class
- Constructor
- typeFullText()
- typeInOneMinute()
- displayResult()
- TimerThread inner class
- Main Class
- Example
- Use of Multithreading
- Conclusion
Introduction
Typing speed is an essential skill in the digital world we live in today. Whether you're a student, a professional, or just someone who spends a lot of time typing, it's important to have a good typing speed and accuracy. In this blog, we'll explore how to create a Java console application that measures typing speed and accuracy.
The application we'll build will have two modes - one where you type the full text and another where you type as much as you can in one minute. The code will provide the typing speed in characters per second and accuracy in percentage. This is a great way to track your progress and improve your typing skills over time. Let's get started!
Features
The features of this code are:
-
Two modes of testing: The code provides two modes of testing, one is typing the full text and the other is typing the text in one minute.
-
Accuracy calculation: The code calculates the accuracy of typing by comparing the input text with the given text.
-
Typing speed calculation: The code calculates the typing speed by dividing the number of characters typed by the time taken to type the text.
-
User-friendly interface: The code provides a user-friendly interface where the user can select the mode of testing and start the test.
-
Thread timer: The code uses multithreading to implement the timer for the one-minute typing test. The timer is implemented as a separate thread which runs alongside the main thread and terminates the test once the time is up.
-
Result display: The code displays the typing speed and accuracy of the test on the console.
-
Exception handling: The code provides basic exception handling for the InterruptedException that can be thrown by the timer thread.
Basic Idea of Implementation
The design strategy for this code is to create a TypingTest class that allows users to perform a typing test with either full text or one minute modes. The flow of the code is as follows:
-
Initialize the TypingTest class with a string for the text to be typed and an integer for the mode of the test.
-
Call the
startTest()
method to start the test. -
If the mode is set to 1, the
typeFullText()
method is called to type the full text and if the mode is set to 2, thetypeInOneMinute()
method is called to type the text in one minute -
According to the method choosen, method prompts the user to start typing, records the start time, and displays the text to be typed.
-
The code then compares each character of the user's input with the corresponding character in the text to be typed, counting the number of correctly typed characters.
-
The accuracy is calculated as a percentage of the number of correct characters, and the typing speed is calculated as the number of characters typed per second.
-
The results are then displayed to the user.
TypingText Class
// Import the Scanner class to allow user input
import java.util.Scanner;
// Class for the typing test
class TypingTest {
// String for the text to be typed
private String text;
// Integer for the mode of the test (full text or one minute)
private int mode;
// Double for the user's typing speed (characters per second)
private double typingSpeed;
// Integer for the user's accuracy (percent)
private int accuracy;
// Constructor for the TypingTest class
public TypingTest(String text, int mode) {
// Set the text to be typed to the passed argument
this.text = text;
// Set the mode of the test to the passed argument
this.mode = mode;
}
// Method to start the test
public void startTest() {
// If the mode is set to full text, run the typeFullText method
if (mode == 1) {
typeFullText();
// If the mode is set to one minute, run the typeInOneMinute method
} else {
typeInOneMinute();
}
}
// Method for typing the full text
private void typeFullText() {
// code is written below
}
private void typeInOneMinute() {
// code is written below
}
private void displayResult() {
// code is written below
}
class TimerThread extends Thread {
// code is written below
}
}
The TypingTest
class is the main class of the typing speed test application. It contains the logic for calculating the typing speed and accuracy of the user. The class has three private variables, text
, mode
, typingSpeed
and accuracy
. These variables store the text that the user has to type, the mode in which the user wants to perform the test (full text or one-minute test), the typing speed of the user, and the accuracy of the user respectively.
- Constructor : The class has a constructor which takes two arguments, the
text
and themode
. This constructor sets the text and mode variables. The class also has astartTest()
method, which is called from the main method to start the test. This method calls eithertypeFullText()
ortypeInOneMinute()
method depending on the value of the mode variable.
// Method for typing the full text
private void typeFullText() {
// Create a Scanner object to allow user input
Scanner sc = new Scanner(System.in);
// Prompt the user to start typing
System.out.println("Start typing the following text:\n");
// Record the start time
long start = System.currentTimeMillis();
// Display the text to be typed
System.out.println(text + "\n");
// String to store the user's input
String input;
// Check if the user has provided input
if (sc.hasNextLine()) {
// Store the user's input in the input variable
input = sc.nextLine();
} else {
// If no input was provided, print a message and return
System.out.println("No input provided.");
sc.close();
return;
}
// Record the end time
long end = System.currentTimeMillis();
// Calculate the elapsed time in seconds
long elapsedTime = (end - start) / 1000;
// Integer to store the number of correctly typed characters
int correct = 0;
// Loop through the text to be typed
for (int i = 0; i < text.length(); i++) {
// If the current character in the text is the same as the user's input
// and the user's input is not shorter than the text
if (i < input.length() && input.charAt(i) == text.charAt(i)) {
// Increment the correct count
correct++;
}
}
// Calculate the accuracy as a percentage of correct characters
accuracy = (int)((double) correct / input.length() * 100);
// Calculate the typing speed in characters per second
typingSpeed = (int)((double) correct / elapsedTime);
// Close the Scanner object
sc.close();
// Display the results
displayResult();
}
- typeFullText() : The
typeFullText()
method is called when the user wants to type the full text. This method displays the text that the user has to type and starts the timer. It then takes input from the user and stops the timer. It then calculates the accuracy and typing speed of the user and displays the result. The accuracy is calculated by comparing the characters typed by the user with the characters in the text. The typing speed is calculated by dividing the number of correct characters typed by the user by the time taken to type the text.
private void typeInOneMinute() {
// Create a Scanner object to read user input
Scanner sc = new Scanner(System.in);
System.out.println("Start typing the following text:\n");
System.out.println(text + "\n");
// Create a TimerThread object to handle the timer for one minute
TimerThread timerThread = new TimerThread();
// Start the timer thread
timerThread.start();
// Use a StringBuilder to collect user input
StringBuilder inputBuilder = new StringBuilder();
// Keep looping until the timer thread finishes
while (timerThread.isAlive()) {
if (sc.hasNextLine()) {
// Add the user input to the StringBuilder
inputBuilder.append(sc.nextLine());
}
}
// Close the Scanner object
sc.close();
// Convert the StringBuilder to a string
String input = inputBuilder.toString();
// Calculate the number of correct characters typed by the user
int correct = 0;
for (int i = 0; i < text.length(); i++) {
if (i < input.length() && input.charAt(i) == text.charAt(i)) {
correct++;
}
}
// Calculate the accuracy as a percentage
accuracy = (int)((double) correct / input.length() * 100);
// Calculate the typing speed in characters per second
typingSpeed = (double) correct / 60;
// Display the results
displayResult();
// Exit the system
System.exit(0);
}
- typeInOneMinute() : The
typeInOneMinute()
method is called when the user wants to type in one minute. This method displays the text that the user has to type and starts a timer thread. The timer thread counts for 60 seconds and then stops. The user can type the text while the timer is running. After the timer stops, the application calculates the accuracy and typing speed of the user and displays the result. The accuracy is calculated by comparing the characters typed by the user with the characters in the text. The typing speed is calculated by dividing the number of correct characters typed by the user by 60 seconds.
private void displayResult() {
// This method is used to display the results of the typing test, including
// typing speed and accuracy.
System.out.println("Typing Speed: " + typingSpeed + " characters per second");
System.out.println("Accuracy: " + accuracy + "%");
}
- displayResult() : The
displayResult()
method is used to display the typing speed and accuracy of the user. The typing speed is displayed in characters per second, and the accuracy is displayed in percentage.
class TimerThread extends Thread {
@Override
public void run() {
// This inner class is used to implement a timer thread that runs for 60 seconds and stops the typing test.
try {
sleep(60000);
System.out.println("\n\nTime is up!\n");
} catch (InterruptedException e) {
// Thread interrupted, do nothing
}
}
}
- TimerThread : The
TimerThread
class is an inner class of theTypingTest
class. This class extends the Thread class and overrides therun()
method. Therun()
method contains the logic to count for 60 seconds and then display a message indicating that the time is up.
Main Class
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// Main method is the entry point of the program and contains the implementation
// of the menu and starts the typing test.
Scanner sc = new Scanner(System.in);
// text is store in Text.java file
String text = Text.text;
System.out.println("Select mode:");
System.out.println("1. Type full text");
System.out.println("2. Type in one minute");
int mode = sc.nextInt();
TypingTest test = new TypingTest(text, mode);
test.startTest();
sc.close();
}
}
In this method, we start by creating a Scanner object to get the input from the user. The text to be typed by the user is defined in the Text.text
variable. The user is prompted to select the mode of the typing test. They can either type the full text or type for one minute. The selected mode is stored in the mode
variable.
Next, we create an object of the TypingTest
class by passing the text and mode as arguments. Finally, we start the typing test by calling the startTest
method on the TypingTest
object. After the typing test is complete, we close the Scanner object to release the system resources.
Example
Let's take a look at the result of the code:
Use of Multithreading
The use of multithreading in the code is to implement the timer feature in the typeinOneMinute mode of the TypingTest. The TimerThread
class extends the java.lang.Thread
class and runs as a separate thread. When the user starts the test, a new instance of TimerThread
is created and its start()
method is called, which starts the timer. The timer runs for 60 seconds and after that, it prints a message saying "Time is up!". During this time, the user can type the given text in the console. The input entered by the user is recorded, and when the timer finishes, the typing speed and accuracy are calculated and displayed to the user.
By using multithreading, the timer and the user input are running concurrently, and the timer does not block the input, so the user can continue typing even if the timer is still running. This makes the typing test more interactive and user-friendly.
Conclusion
In conclusion, typing speed is an essential skill in today's digital world, and being able to measure it is equally important. The above Java console application provides an easy and straightforward way to measure your typing speed and accuracy.
In this article at OpenGenus, we have explained the code step by step and the different parts of the code in detail. We hope that this blog has provided you with all the information you need to start using this application and improving your typing skills.