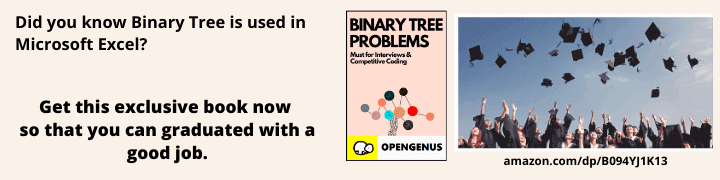
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Why BigInteger
- BigInteger Methods
- BigInteger Key Methods
- Example Question on BigInteger Methods
- How to convert BigInteger to int and vice versa
- Summary
Why BigInteger
BigInteger is one of the useful classes found in java.math library. It is used to represent arbitrary precision integers. It comes in handy when we want to deal with digits that primitive integers can no longer handle, numbers whose capacity is way beyond 32 and 64 bits. BigIntegers allow us to work with virtually unlimited sizes. We can either use them for arithmetic purposes, comparisons or other miscellaneous operations. BigIntegers are immutable, meaning that the value cannot be changed once it has been set.
BigInteger Methods
BigInteger contains numerous methods which can be used depending on what you intent to do. There are methods which can suit the following:
- Arithmetic Operations
- Comparison Operations
- Bitwise operations
- Shift Operations
These are only few of the example methods that can be found in the BigInteger class. We are going to look at a few examples using arithmetic, comparison and other useful operations that can be done using BigInteger.
The BigInteger class can be accessed by using:
import java.math.BigInteger;
After this, we will have a variety of methods at our disposal. For our first examples, let us take a look at the some of the methods that can be used.
- add()
import java.math.BigInteger; public class Add { public static void main(String[] args) { // creating and initializing big integer objects with their values BigInteger num1 = new BigInteger("234998380290487"); BigInteger num2 = new BigInteger("87839837927649"); // using the add method BigInteger sum = num1.add(num2); // printing out the value of adding num1 and num2 System.out.println("Sum of num1 and num2: " + sum); } }
Output:
Sum of num1 and num2: 322838218218136
- multiply()
Output:import java.math.BigInteger; public class Multiply { public static void main(String[] args) { // creating big integer objects with the integer values we want BigInteger num1 = new BigInteger("23478753"); BigInteger num2 = new BigInteger("763482983"); // we create a new big integer variable, which will store the product of the num1 and num2 // multiply method is denoted as bigInteger value.multiply(big integer value) BigInteger product = num1.multiply(num2); // we then print the product System.out.println("Product of num1 and num2: " + product); } }
Product of num1 and num2: 20642219637976403208708975063
- nextProbablePrime()
This method returns the next integer that is greater than the big integer given and the integer will be a prime number:
import java.math.BigInteger;
public class nextProbablePrime {
public static void main(String[] args) {
BigInteger value = new BigInteger("100");
BigInteger probablePrime = value.nextProbablePrime();
System.out.println("Next probable prime: " + probablePrime);
}
}
Output:
Next probable prime: 101
- isProbablePrime()
This method checks if the given BigInteger is a probable prime with a certainity of a given number.
import java.math.BigInteger;
public class isProbablePrime {
public static void main(String[] args) {
BigInteger value = new BigInteger("101");
boolean isPrime = value.isProbablePrime(10);
System.out.println(value + " is a probable prime: " + isPrime);
}
}
Output:
101 is a probable prime: true
- sqrt()
The sqrt() method is used to find the square root of a given number
import java.math.BigInteger;
public class sqrt {
public static void main(String[] args) {
BigInteger value = new BigInteger("25");
BigInteger sqrtValue = value.sqrt();
System.out.println("The square root of " + value + " is " + sqrtValue);
}
}
Output:
The square root of 25 is 5
- xor()
This performs the xor operation on 2 numbers:
import java.math.BigInteger;
public class xor {
public static void main(String[] args) {
BigInteger num1 = new BigInteger("10");
BigInteger num2 = new BigInteger("5");
BigInteger result = num1.xor(num2);
System.out.println("Result of XOR: " + result);
}
}
Output:
Result of XOR: 15
- flipBit()
This method flips the bit of a big integer number at a specified index. Please note that the number will be flipped in binary, not in decimal form.
import java.math.BigInteger;
public class FlipBit {
public static void main(String[] args) {
BigInteger originalValue = new BigInteger("10");
BigInteger flippedValue = originalValue.flipBit(2);
System.out.println("Original Value: " + originalValue);
System.out.println("Value after flipping bit at position 2: " + flippedValue);
}
}
Output:
Original Value: 10
Value after flipping bit at position 2: 14
- bitCount()
This method counts the number of set bits of a given big integer i.e. the number is converted to binary and the bits are then counted in the range of 1 to 8.
import java.math.BigInteger;
public class BitCount {
public static void main(String[] args) {
BigInteger value = new BigInteger("255");
// Counting the number of set bits
int numberOfSetBits = value.bitCount();
System.out.println("Value: " + value);
System.out.println("Number of set bits: " + numberOfSetBits);
}
}
Output:
Value: 255
Number of set bits: 8
The above code snippets demonstrates how we can use some of the arithmetic methods that BigInteger has to offer. They also illustrate how we can create BigInteger Objects and initialize the objects with respective values. There are other arithmetic methods which are subtract(), divide(), remainder(), nextProbablePrime, probablePrime(), sqrt(), xor(), flipBit() and BitCount().
BigInteger Key Methods
BigInteger class has other key methods such as gcd() that can help us to find the greatest common divisor between numbers. Also we can also utilize some other methods such as max(), min() to find the maximum and minimum between a given list of numbers and abs() to find the absolute value (positive value) of a given number. The following is a list of the key methods of BigInteger:
- add()
- subtract()
- remainder()
- multiply()
- compareTo()
- gcd()
- and()
- or()
- abs()
- negate()
The list contains just a few since there is quite a number of them.
Example Question
Let us consider the following question:
You are given a list of integers, and you need to perform several operations on them. Write a Java program to solve the following problem:
Calculate the Greatest Common Divisor (GCD) of all the integers in the list.
Find the maximum and minimum values in the list.
Ensure that all the numbers in the list are positive.
Program Requirements:
- Create a Java program that takes 2 numbers as input.
- Calculate and display the GCD of the integers.
- Find and display the maximum and minimum values between the 2.
Solution
import java.math.BigInteger;
import java.util.Scanner;
public class GCD {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String userInput;
System.out.println("Enter num1: ");
userInput = scanner.next();
// creating a big integer object and initializing it
BigInteger value1 = new BigInteger(userInput);
System.out.println("Enter num2");
userInput = scanner.next();
BigInteger value2 = new BigInteger(userInput);
// using the gcd() method to find the greatest common divisor between 2 numbers
BigInteger gcdValue = value1.gcd(value2);
System.out.println("GCD: " + gcdValue);
// using the max() method to find the maximum number between the 2
BigInteger maxValue = value1.max(value2);
System.out.println("Max value: " + maxValue);
// using the min() method to find the minimum number between the 2
BigInteger minValue = value1.min(value2);
System.out.println("Min value: " + minValue);
}
}
Output
Enter num1:
8374923802085
Enter num2
90384853245
GCD: 5
Max value: 8374923802085
Min value: 90384853245
The program prompts the user to enter the first number, the input is read as a string because BigInteger accepts strings as inputs. Then the user input for num1 is converted to a BigInteger object called value1. The same steps are done for num2 as well. Then the gcd() method is used to calculate the Greatest Common Divisor (GCD) of value1 and value2 with the result being stored in gcdValue and the printed to the screen. After finding the GCD, the max() and min() methods are used to find the maximum and minimum values between value one and 2, then the results are also printed.
How to convert BigInteger to int and vice versa?
Coverting a big integer to an integer is quite an easy task. There is one issue though, we have to be careful on the value of the big integer that we want to convert into an integer. If it is way too big to be handled by an integer, that may cause an overflow. To convert a big integer to an integer we can do this:
import java.math.BigInteger;
public class BigIntegerToInt {
public static void main(String[] args) {
BigInteger bigIntegerValue = new BigInteger("123456789");
// Converting BigInteger to int
int intValue = bigIntegerValue.intValueExact();
System.out.println("Converted int value: " + intValue);
}
}
As we can see in the above example, we used a method called intValueExact which converts the given bigInteger to an integer provided that the BigInteger value is not out of range of the supported int values.
How to convert int to BigInteger?
Converting an integer to a BigInteger is also as simple as the above method, but this time instead of using intValueExact, we use the valueOf method. It is used like this:
import java.math.BigInteger;
public class IntToBigInteger {
public static void main(String[] args) {
// Converting int to BigInteger
int intValue = 123456;
BigInteger bigIntValue = BigInteger.valueOf(intValue);
// Displaying the results
System.out.println("Original int value: " + intValue);
System.out.println("Converted BigInteger value: " + bigIntValue);
}
}
The BigInteger valueOf method takes a single int parameter, and then converts it to a BigInteger value.
Summary
To summarize in short, we looked at the BigInteger class, discussed about it's immutability meaning that once values have been set, they cannot be changed. We also looked at some methods that are found in the BigInteger class such as add(), multiply(), gcd(), max() and min. We also looked at how we can initialize the BigInteger objects with string only values.