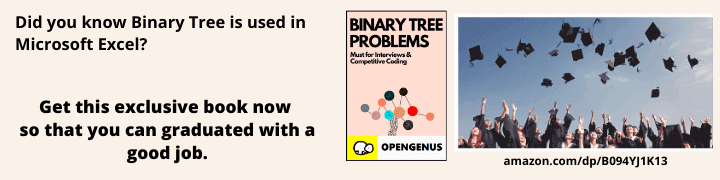
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of contents:
- Introdution for Producer-Consumer
- Basic outline
- How to implement in Java?
- Summary
Introdution for Producer-Consumer
The Producer-Consumer problem is a classic example of a multi-process synchronization problem. The problem describes two processes, the producer and the consumer, who share a common, fixed-size buffer used as a queue. The producer generates data, puts it into the buffer, and starts again. At the same time, the consumer consumes the data (removes it from the buffer), one piece at a time.
there are some problems we should focus on:
- make sure the producer matches the consumer so that we won't lose data and consumer can receive message from producer
- the producer won't try to add data into the buffer if it's full and the consumer won't try to remove data from an empty buffer.
- how to implement using wait() and notify() methods in Java to make sure that multiple threads of producer and consumer can run correctly
Basic outline
-
Shared Resource (Buffer): This is a shared resource between the producer and consumer. Its implementation must ensure it's thread-safe. For this problem, we can use message queue like rabiitMQ or memory-based database like Redis
-
Producer:
Checks if the buffer is full.
If the buffer is full, it goes to the waiting state (wait()).
If the buffer has space, it produces an item and puts it in the buffer.
After producing, it notifies the consumer (notify() or notifyAll()) that it has produced an item. -
Consumer:
Checks if the buffer is empty.
If the buffer is empty, it goes to the waiting state (wait()).
If there are items in the buffer, it consumes an item.
After consuming an item, it notifies the producer (notify() or notifyAll()) that it has consumed an item. -
Synchronization: The wait() and notify() methods should be used within a synchronized block or method to ensure that the buffer is being modified by only one thread at a time.
How to implement in Java?
class Buffer {// this is an example for memory-shared buffer
private final int[] items;
private int count = 0;
private final int capacity;
public Buffer(int capacity) {
this.capacity = capacity;
this.items = new int[capacity];
}
public synchronized void put(int item) throws InterruptedException {
while (count == capacity) {
wait(); // Wait if buffer is full
}
items[count++] = item;
notify(); // Notify a single waiting thread
}
public synchronized int get() throws InterruptedException {
while (count == 0) {
wait(); // Wait if buffer is empty
}
int item = items[--count];
notify(); // Notify a single waiting thread
return item;
}
}
class Producer extends Thread {
private final Buffer buffer;
public Producer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
buffer.put(i);
System.out.println("Produced: " + i);
Thread.sleep(1000); // Simulating work
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
class Consumer extends Thread {
private final Buffer buffer;
public Consumer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
int item = buffer.get();
System.out.println("Consumed: " + item);
Thread.sleep(1000); // Simulating work
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class ProducerConsumerExample {
public static void main(String[] args) {
Buffer buffer = new Buffer(5);
Producer producer = new Producer(buffer);
Consumer consumer = new Consumer(buffer);
producer.start();
consumer.start();
}
}
And then ,I provide another implement using message queue, which is more common in development. The main advantage of using RabbitMQ is that it supports asynchronous processing, allowing producers and consumers to work independently, which is very useful for distributed systems and microservice architectures.
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
class Producer {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
String message = "Hello World!";
channel.basicPublish("", QUEUE_NAME, null, message.getBytes());
System.out.println(" [x] Sent '" + message + "'");
}
}
}
class Consumer {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
Connection connection = factory.newConnection();
Channel channel = connection.createChannel();
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
System.out.println(" [*] Waiting for messages. To exit press CTRL+C");
DeliverCallback deliverCallback = (consumerTag, delivery) -> {
String message = new String(delivery.getBody(), "UTF-8");
System.out.println(" [x] Received '" + message + "'");
};
channel.basicConsume(QUEUE_NAME, true, deliverCallback, consumerTag -> { });
}
}
Summary
-
wait() Method:
When a thread calls the wait() method of an object, it suspends its execution and releases the lock of that object, entering a waiting state. This is typically used to wait for a certain condition to be met.
The thread remains in the waiting state until another thread calls notify() or notifyAll() on the same object, or until it is interrupted.
The wait() method is usually called within a loop to check if the condition is met, to guard against spurious wakeups. -
notify() Method:
When a thread calls the notify() method of an object, it notifies one of the threads waiting on that object (if any) so that the notified thread can attempt to re-acquire the object lock.
The notify() method does not immediately release the lock; it simply marks one waiting thread (if any) for being woken up once the current thread leaves the synchronized block.
notify() wakes up only one waiting thread. If there are multiple threads waiting, which one will be woken up is indeterminate. -
something to keep in mind
- Deadlock Prevention:Ensure that wait() is always called in a loop checking the condition on which it's waiting (while loop is preferred over if to avoid spurious wakeups).Carefully design the logic to avoid situations where all threads end up waiting and none are left to call notify() or notifyAll().
- Object's Lock:Remember that wait(), notify(), and notifyAll() must be called within a synchronized block or method. This synchronization is on the object that threads are calling wait() or notify() on.
- Resource Management:Be mindful of resource usage. A buffer or queue that is too large or too small might affect performance and resource utilization.
- Performance Considerations:Excessive use of wait() and notify() can lead to performance bottlenecks, especially if many threads are contending for the same lock.
- Alternatives:For many use cases, higher-level concurrency constructs like BlockingQueue from java.util.concurrent might be more suitable and easier to manage.