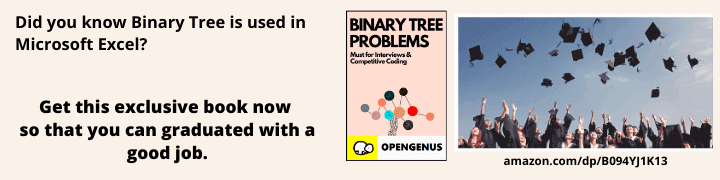
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A constructor is a special method that is used to initialize an object. Constructors are defined inside a class; with the same name as the class in JAVA but in Scala that is not the case. We use this keyword to initialize a constructor in Scala. There are 2 types of classes in Scala, primary constructor and auxiliary constructor. In Scala, we don't have to code getter and setter methods for primary constructors.
Table of contents:
- Introduction to Constructor
- Defining a primary constructor
- Need of Auxiliary Constructor
- Auxiliary Constructor code
Introduction to Constructor
A constructor is a special method that is used to initialize an object. Constructors are defined inside a class; with the same name as the class in JAVA but in Scala that is not the case.
In Scala, a constructor is a special method that is used to initialize the state of an object. There are two types of constructors in Scala: primary constructors and auxiliary constructors.
- Primary Constructors: They are defined in the class definition.
- Auxiliary Constructors: They are defined in the body of the class with the use of this keyword.
Defining a primary constructor
Before understanding the auxiliary constructor, first, we need to understand the primary constructor. It is defined with the class like we define the parameters of a function with the function name. We can also set whether we want a getter and setter method for the values of the class parameters. If we want to get and set the values of the constructor through object then we should define the values of the primary constructor as var. If we want just the getter method but not the setter method then we should use val. If we don't want any getter and setter method then we should just leave it as it is without defining anything before the variable.
- Getter and Setter of the primary constructor
object ExampleForBlog extends App {
val person = new Person("Diwash", "Student") // Creating an object of the class by passing parameters to the constructors
// Getting the value of the class constructors
println(person.name) // Diwash
println(person.occupation) // Student
// Setting the value of the class constructors
person.name = "Mainali"
person.occupation = "Teacher"
println(person.name) // Mainali
println(person.occupation) // Teacher
}
class Person(var name: String, var occupation: String) // Class that takes 2 variable as parameter; i.e constructors of the class
- Getter of the primary constructor
object ExampleForBlog extends App {
val person = new Person("Diwash", "Student")
// Getting the value of the class constructors
println(person.name) // Diwash
println(person.occupation) // Student
// Setting the value of the class constructors
person.name = "Mainali" // Reassignment to val Error
person.occupation = "Teacher" // Reassignment to val Error
}
class Person(val name: String, val occupation: String)
- Default variable
object ExampleForBlog extends App {
val person = new Person("Diwash", "Student")
// Getting the value of the class constructors
println(person.name) // Value name cannot be accessed as a member of the class
println(person.occupation) // Value occupation cannot be accessed as a member of the class
// Setting the value of the class constructors
person.name = "Mainali" // Value name cannot be accessed as a member of the class
person.occupation = "Teacher" // Value occupation cannot be accessed as a member of the class
}
class Person(name: String, occupation: String)
Need of Auxiliary Constructor
Primary constructors are great and powerful but what if we want to create an object that takes some additional parameters or some less parameters? Well, this is where the need for an Auxiliary Constructor comes in.
Auxiliary Constructors AKA Additional Constructors are defined in the class body to allow different ways to instantiate an object of the class. By using auxiliary constructors, we can create instances with different levels of complexity and avoid the need to create multiple classes to handle varying constructor signatures.
Auxiliary Constructor code
class Person(name: String, occupation: String) {
def this(age: Int, dob: Int) = this("defaultValue", "defaultValue")
}
With this article at OpenGenus, you must have a good understanding of Primary and Auxiliary constructors in Scala Programming Language.