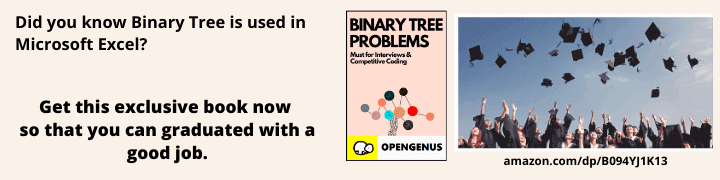
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 18 minutes | Coding time: 22 minutes
Introduction
Scala, short for "Scalable Language," is a modern programming language that blends object-oriented and functional programming paradigms. It was designed to address the limitations and complexities of Java while offering a concise, expressive, and flexible syntax. Developed by Martin Odersky and his team, Scala was first released in 2003 and has since gained popularity in various domains including web development, data analysis, and distributed systems.
Scala runs on top of the Java Virtual Machine (JVM), allowing developers to seamlessly integrate with existing Java libraries and frameworks. Its versatile nature makes it suitable for both small scripts and large-scale applications. Scala's strong static typing, type inference, and compatibility with Java have contributed to its rise in the software development landscape.
Getting Started with Scala
Before diving into the specifics of Scala programming in this OpenGenus article, let's set up the development environment and get a basic understanding of how Scala code is executed.
Installation
To start programming in Scala, you need to have the Java Development Kit (JDK) installed on your system, as Scala runs on the JVM. Once you have JDK installed, follow these steps to install Scala:
- Download Scala: Visit the official Scala website to download the latest version of Scala.
- Installation: Follow the installation instructions for your operating system. Scala usually comes with an easy-to-use installer.
- Environment Setup: After installation, you need to set up environment variables. Add the bin directory of the Scala installation to your system's PATH variable.
Running Scala Code
Scala code can be executed using the Scala interpreter, which allows you to write and execute code interactively. To start the Scala interpreter, open a terminal and simply type scala. This will launch the interpreter, where you can type and execute Scala expressions and statements.
$ scala
Welcome to Scala 2.13.5 (OpenJDK 64-Bit Server VM, Java 11.0.11).
Type in expressions for evaluation. Or try :help.
scala> val greeting = "Hello, Scala!"
greeting: String = Hello, Scala!
scala> println(greeting)
Hello, Scala!
scala> 2 + 3
res2: Int = 5
scala> :q
$
In the above example, we defined a variable greeting and printed its value. We also performed a simple addition using the interpreter.
Variables and Data Types
In Scala, variables can be declared using the var or val keyword. The difference between them lies in mutability. Variables declared with val are immutable, meaning their value cannot be changed once assigned. Variables declared with var are mutable, allowing their value to be changed.
val x = 10 // Immutable variable
var y = 5 // Mutable variable
Scala provides a rich set of data types, both primitive and reference types, similar to Java. Some common data types include Int, Double, String, Boolean, and more. Scala also supports type inference, which allows the compiler to automatically determine the data type based on the assigned value.
val age: Int = 30
val pi: Double = 3.14159
val name: String = "Alice"
val isStudent: Boolean = true
Control Structures
Conditional Statements
Scala supports traditional if, else if, and else statements for conditional branching. The syntax is similar to other programming languages.
val num = 10
if (num > 0) {
println("Positive")
} else if (num < 0) {
println("Negative")
} else {
println("Zero")
}
Loops
Scala provides both imperative and functional approaches to looping. The for loop is widely used and can be used for iteration over ranges, collections, and more.
for (i <- 1 to 5) {
println(i)
}
val numbers = List(1, 2, 3, 4, 5)
for (num <- numbers) {
println(num)
}
Another powerful construct is the while loop:
var i = 0
while (i < 5) {
println(i)
i += 1
}
Functions in Scala
Functions are a fundamental building block of Scala programs. They can be defined using the def keyword. Scala functions can take parameters, return values, and can be used in a functional programming style.
def add(x: Int, y: Int): Int = {
x + y
}
val result = add(3, 5)
println(result) // Output: 8
Functions in Scala are also first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
val multiply = (x: Int, y: Int) => x * y
val product = multiply(4, 6)
println(product) // Output: 24
Object-Oriented Programming in Scala
Scala is a hybrid language that seamlessly integrates object-oriented and functional programming. Everything in Scala is an object, including primitive types.
Classes and Objects
Classes are the blueprint for creating objects. Scala classes can have fields, methods, constructors, and more. Here's an example of a simple class:
class Person(firstName: String, lastName: String) {
def fullName: String = s"$firstName $lastName"
}
val person = new Person("John", "Doe")
println(person.fullName) // Output: John Doe
Scala also supports case classes, which are commonly used for immutable data structures. They come with automatically generated methods for equality and pattern matching.
case class Point(x: Int, y: Int)
val p1 = Point(2, 3)
val p2 = Point(2, 3)
println(p1 == p2) // Output: true
Inheritance and Polymorphism
Scala supports single-class inheritance as well as the use of traits for multiple inheritance. Traits are similar to interfaces in Java but can also include concrete methods.
class Animal(name: String) {
def speak(): Unit = println(s"$name makes a sound")
}
class Cat(name: String) extends Animal(name) {
override def speak(): Unit = println(s"$name meows")
}
val cat: Animal = new Cat("Whiskers")
cat.speak() // Output: Whiskers meows
Pattern Matching
Pattern matching is a powerful feature in Scala that allows you to match values against patterns and execute different code blocks accordingly. It is often used with case classes.
def matchNumber(num: Int): String = num match {
case 0 => "Zero"
case 1 => "One"
case _ => "Other"
}
println(matchNumber(2)) // Output: Other
Pattern matching can also be used to destructure case class instances:
case class Person(name: String, age: Int)
val person = Person("Alice", 30)
person match {
case Person(name, age) => println(s"$name is $age years old")
}
Collections and Data Structures
Scala provides a rich set of collection types for handling and manipulating data. Some common collections include lists, sets, maps, arrays, and more. Collections can be mutable or immutable.
Lists
Lists are ordered collections that can hold elements of the same type.
val numbers = List(1, 2, 3, 4, 5)
val doubled = numbers.map(_ * 2)
println(doubled) // Output: List(2, 4, 6, 8, 10)
Sets
Sets are collections of distinct elements.
val colors = Set("red", "green", "blue", "green")
println(colors) // Output: Set(red, green, blue)
Maps
Maps are key-value pairs where keys are unique.
val ages = Map("Alice" -> 30, "Bob" -> 25)
println(ages("Alice")) // Output: 30
Concurrency and Parallelism
Scala provides concurrency and parallelism support through the Akka framework and the Future API.
Futures
Futures represent a value that may not be available yet. They are used to perform asynchronous operations and handle results when they become available.
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val futureResult: Future[Int] = Future {
// Perform some time-consuming operation
42
}
futureResult.map(result => println(s"Result: $result"))
Functional Programming
Scala promotes functional programming by providing support for higher-order functions, immutability, and other functional constructs.
Higher-Order Functions
Higher-order functions take one or more functions as arguments or return a function.
val numbers = List(1, 2, 3, 4, 5)
val squared = numbers.map(x => x * x)
println(squared) // Output: List(1, 4, 9, 16, 25)
Immutability
Immutable data structures are a cornerstone of functional programming. Scala encourages immutability to avoid unintended side effects.
Applications of Scala
Scala's versatility has led to its adoption in various domains:
- Web Development: Scala can be used for building web applications using frameworks like Play and Akka HTTP. These frameworks provide features for building scalable and high-performance web services.
- Data Analysis: Scala is gaining popularity in the field of data analysis and data science. Libraries like Apache Spark provide Scala APIs for distributed data processing and machine learning.
- Concurrent and Distributed Systems: Scala's concurrency support through the Akka framework makes it suitable for building highly concurrent and fault-tolerant systems.
- Functional Programming: Scala's strong support for functional programming makes it an excellent choice for projects that require elegant and expressive code.
Conclusion
In this OpenGenus article, we saw Scala is a powerful and expressive programming language that offers the best of both object-oriented and functional programming paradigms. Its compatibility with Java, conciseness, and versatility make it a popular choice for various application domains. By understanding the basics covered in this guide, you've taken the first step toward becoming proficient in Scala programming. From here, you can explore its advanced features, libraries, and frameworks to build sophisticated and robust applications. Happy coding!