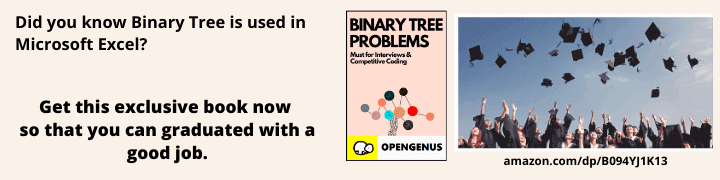
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Ruby is an interpreted, high-level, general-purpose programming language that was designed and developed by Yukihiro "Matz" Matsumoto in the mid-1990s.
Some of it's features include:
- dynamic typing and duck typing
- multiple paradigms support(object-oriented/procedural/functional)
- reflective typing
- garbage collection
- centralized package management through Ruby Gems
- exception handling
The inspirations behind Ruby were Perl, Smalltalk, Ada, BASIC, and Lisp.
Semantics
Ruby has taken it's semantics, as well as language structures, from a bunch of other pre-existing languages.
- Variables in Ruby always refer to their objects.
- Every function is a method, and methods are always called on objects.
- Ruby supports inheritance - features include dynamic dispatch, mixins and singleton methods.
- Ruby does not support multiple inheritance directly - but instead, classes can import modules as mixins. A mixin is a class, that has been mixed with a module.
- Ruby is also a multi-paradigm programming language - it can be written as procedural as well as functional programming language.
Philosophy
Ruby was designed to make programming extremely easy, and readable for better productivity and fun. Usually, programming languages can be quite overwhelming for a beginner, but this is not the case with Ruby.
It follows the principle of least astonishment(POLA), which means that language minimizes the confusion for experienced users. A simple for-loop in C++ looks like this-
#include <iostream>
using namespace std;
int main()
{
for(int i=1;i<=5;i++)
{
cout<<"hello world"<<endl;
}
return 0;
}
whereas, in Ruby, it can be written on a single line-
5.times{puts("hello world")}
Syntax
Ruby has it's syntax similar to Python or Perl. Classes and methods are defined by keywords, whereas code blocks can be defined by either keywords, or braces.
One of the features that makes Ruby different from Python or Perl, is that Ruby keeps it's instance variables completely private to the class and only exposes them through accessor methods(unlike the conventional "getter" and "setter" methods).
Installing Ruby
This article assumes that you have never installed Ruby on you computer before, be it for Rails framework, or Shoes GUI toolkit, etc.
For Windows:
Head to the following link (this version is known as the RubyInstaller), and download current stable version (2.7.2 at the time of writing this article).
Warning!!
Avoid installing this Ruby flavour on your PC. Railsinstaller is outdated, and the development has ceased back in 2017. Also, the Ruby version is old, along with many broken gem dependencies.
You may also see another setup with it, called the Ruby Installer for Windows 2. Just press [ENTER], and it will auto-select the required dependencies.
Now, to see if Ruby has been installed, open the command prompt, and try typing this-
ruby -v
If nothing shows up, it maybe because of the following:
- Improper installation (missing DevKit)
- PATH not set properly
Usually, it is always the later, so copy this location "C:\Ruby27-x64\bin" (this won't be different, unless you've changed the installation location) and type "Environment Variables" on the search bar in start menu. Click on "Environment Variables", and look for "Path" in "User Variables", click on Edit, add the above path, then click on Ok, and try the Ruby command again.
Windows version do not have any version managers (Pik and Uru are discontinued/development has ceased), and this is why, when it comes to frameworks like Ruby on Rails, Linux is recommended. A simple work-around, would be, to install the version you want to work on, and modify the Path that you have added before. So, the new path would be "C:<path-to-Ruby>\bin", instead of "C:\Ruby27-x64\bin".
For UNIX based systems:
For Linux, and Mac, installing a Ruby Version Manager is recommended. This is one of the actual reason, why Ruby is enjoyed a lot on UNIX based platforms, and is thus, superior, over Windows.
Follow the instructions to install RVM here. They have a well-explained guide on how to install Ruby for Linux, as well as switching the versions.
Warning!!
If you are using a Mac, remove the in-built Ruby first, and then proceed to install RVM.
Interaction
Similar to Python's shell(iPython), Ruby has it's own REPL called the IRB(Interactive Ruby Shell). This can be accessed by typing the following command in the terminal-
$ irb
irb(main):001:0>
Note:
When we are talking of terminal (also called a shell program), it could be any of the following-
- Command Prompt (Windows)
- Powershell (Windows)
- Bash (Linux)
- Zsh (Linux)
- Terminal (Mac)
It could even include third party consoles too, like Git Bash or MSYS2.
We will recommend you to stick to the platform default terminal. Although in the example, we are using the Command Prompt for Windows, we will very much recommend to stick to Powershell.
Hello World!!
For any beginner or expert wanting to get into a new programming language, we begin with a "Hello World!" example.
It is that simple to print an output in Ruby.
Now, the same can be done without using the IRB shell. I'll be using Visual Studio code, but you can stick with any code editors of your choice. Create a new file called 'helloworld.rb' inside a folder. Open it, and type the same code.
To run the code from the file, change directory to the given location, and then type 'ruby <name_of_file.rb>'.
This is how you can run the code inside the .rb file.
Note:
In case, if you're using a modern editor like VSCode, or Sublime, you can access the integrated terminal from within the interface.
Ruby Style Guide
Note:
This might contain too much information to digest in, but you don't have to remember them all.
Just give a brief read, and refer when required.
What is RuboCop?
RuboCop is a linter/formatter that checks for stylistic errors.
A linter is used to improve the quality of code, and to make it readable to others. It highlights the associated style errors as well as warnings (style is the keyword here - both linters and formatters cannot fix structural or logical errors).
A formatter, on the other hand, tries to fix styling errors that it can detect, be it code spacing, line length, or argument positioning.
To install RuboCop, type in the terminal-
gem install rubocop
To use RuboCop, change the directory to the file location, and simple type 'rubocop'
Note:
If you see a "Style/FrozenStringLiteralComment: Missing frozen string literal comment" error, you may either choose to ignore for now, or add this to the first line of the file, as a good practise.
Although this may look like a comment, it is half-wrong to say so.
Ruby has this feautre known as magic comments.
"# frozen_string_literal: true"
The true purpose of this magic comment is purely for performance. Adding this makes the string in your code immutable, as opposed to mutable.
Warning!!
There is a difference between immutable strings and immutable objects.
Examples of immutable objects include int, float, string, etc.
On the other hand, immutable strings cannot be modified during the execution of the program. Any operation on it will cause it to produce exception.
Naming Conventions
Type | Convention |
---|---|
Class | PascalCase |
Constant | SCREAMING_SNAKE_CASE |
Module | snake_case |
Method | snake_case |
Package | PascalCase |
Variable | snake_case |
Tabs or Spaces?
Use only spaces for indentation. No hard tabs. (A Tab is equal to usually four spaces depending on the code editor used).
For example, this following snippet-
# bad practise - no four spaces
def some_method
....do_something
end
# good practise
def some_other_method
..do_something
end
How many words in a single lines?
Line length should be limited to 80 characters. But the maximum limit is 120.
Semicolons
Semicolons can be used, but they have been discouraged. Usually, this is used only to cram multiple statements onto a single line.
puts 'Hello World!'; puts 'Can you see this!'; puts 'Okay, bye!'
Comments
Comments are the part of code, that are ignored by the interpreter.
In Ruby, a single line comment is added in the following symbol '#'.
# frozen_string_literal: true
# This is a single line comment.
puts "See, comments have been ignored!"
To add a multi-line comment, this was the conventional way-
=begin
Multiple line of comments.
This is the second line.
=end
Block comments are not used anymore, and most Ruby programmers still stick to multiple single line comments because:
- They cannot be preceded by whitespace
- Not as easy to spot as regular comments
- Poor readability
Note:
Linter/formatter plugins like RuboCop may show warnings, if you have chosen to use block comments.