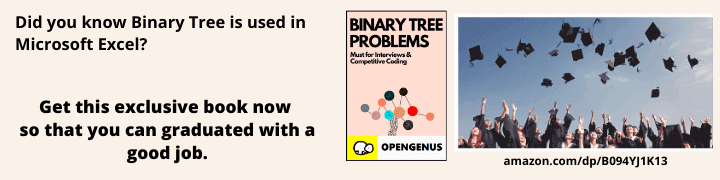
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to develop a Calculator in C++ Programming Language as a console application. This is a strong beginner project for SDE Portfolio.
There are many ways to implement a simple calculator console.
When I first heared about calculator and C++ the first thing in my mind was to use the overloading arithmetic operators using the C++ super strong functionality.
They are basically regular functions with special names: their name begins by the operator keyword followed by the operator sign that is overloaded. The syntax is:
type operator sign (parameters) { /... body .../ }
where sign might be any of the recognized operators +,-,*,/,%,^ etc.
As you already know from mathematic addition and multiplication are commutative so it is not important how you introduce the operands for computation.
But in case of subtraction and division that behavior does not apply.
The order of writting them is very important, and more than this, in case of division a check of the right operand to not be divided to 0 must be taken into consideration.
There are 2 ways to implement that:
-
using one paramenter
return_type operator - (parameter_type r) which in this case r is on the right side -
using two parameters
return_type operator - (paramter_type l, paramter_type r) which in this case l is on the left side and r on the right side
Remember that compiler knows if an operator is unary,binary or ternary so you must implement accordingly. In the example below I overload the bitwise operator ^ and gave it another interpretation of an arithmetic one such as the power of a variable.
Operators in C++ | ||
---|---|---|
Operator | Type | |
Unary | ++ , -- | Increment |
Binary | + , - , * , / , % | Arithmetic |
< , <= , > , >= , != , == | Relational | |
&& , || , ! | Logical | |
& , | , << , >> , ~ , ^ | Bitwise | |
= , += , -= , *= , /= , %= | Assignment | |
Ternary | ?: | Conditional |
You can also declare parameters with const Object_name & to avoid changing them or ovarload the << (shifting) operator to display the result to the console.
A way of implementation
Ver 1 is a simple reading loop operation and return the result each time.
It reads from the console in exactly the same order the variables x, opr and y.
The x and y are of type double and opr is of type char to retain the operation that needs to be done.
After reading the values 2 Operand objects a and b are instantiated with those values and a third one c_out is used for showing the result to the console.
#include <iostream>
using namespace std;
class Operand
{
double a;
public:
Operand()=default;
Operand(double x):a(x) {};
Operand operator + (Operand x) {return Operand(a + x.a);}
Operand operator - (Operand x) {return Operand(a - x.a);}
Operand operator * (Operand x) {return Operand(a * x.a);}
Operand operator / (Operand x) {return Operand((x.a!=0)?a/x.a:0);}
Operand operator % (Operand x) {return Operand((int)a%(int)x.a);}
Operand operator ^ (Operand x)
{
if ((int)x.a == 0) return 1;
double v=a;
for(int i=(int)x.a-1; i>0; i--) a=a*v;
return *this;
}
void operator << (Operand x) {cout<<x.a;}
};
int main()
{
double x,y;
char opr;
while( cin>>x>>opr>>y )
{
Operand a(x),b(y),c_out;
switch(opr)
{
case '+': cout<<"="; c_out<<a+b; cout<<endl; break;
case '-': cout<<"="; c_out<<a-b; cout<<endl; break;
case '*': cout<<"="; c_out<<a*b; cout<<endl; break;
case '/': cout<<"="; c_out<<a/b; cout<<endl; break;
case '%': cout<<"="; c_out<<a%b; cout<<endl; break;
case '^': cout<<"="; c_out<<(a^b); cout<<endl; break;
default: break;
}
};
return 0;
}
Output:
9+6
=15
3.14^2
=9.8596
8/0
=0
3/5
=0.6
15%4
=3
5*5
=25
Ver 2
The downside of the first version is that you cannot use the previos result into the next computation. So, some changes need to be done to achieve that.
First, we need to rethink how we read the variables from the console in order to retain the result each time and also to have the posibility to start over again.
The best way to do so is to read the entire line via the getline function and substring after that the position of the operator and the operand values.
If the operator is on the first position then the first value will retain the previous value computed before that operation.
The stod function will help us to convert a string into a double variable.
The contains function is a programmer defined function that will help us to find the position of one character in a string, i.e the position of the operator in the line to determine after that the operands values.
If we introduce a wrong operator the contains function will return -1 and all variables will be reset and a clear screen will be made.
Depending on the OS you will need to chose the corect argument for the system call.
#include <iostream>
#include <stdlib.h>
#include <string>
#include <cstring>
using namespace std;
class Operand
{
double a;
public:
Operand()=default;
Operand(double x):a(x) {};
Operand operator + (Operand x) {return Operand(a + x.a);}
Operand operator - (Operand x) {return Operand(a - x.a);}
Operand operator * (Operand x) {return Operand(a * x.a);}
Operand operator / (Operand x) {return Operand((x.a!=0)?a/x.a:0);}
Operand operator % (Operand x) {return Operand((int)a%(int)x.a);}
Operand operator ^ (Operand x)
{
if ((int)x.a == 0) return 1;
double v=a;
for(int i=(int)x.a-1; i>0; i--) a=a*v;
return *this;
}
Operand operator = (Operand x)
{
a=x.a;
return *this;
}
void operator << (Operand x) {cout<<x.a;}
void set(double x){a=x;}
double get() {return a;}
};
int contains(string source, string find)
{
int pos, length = find.size();
char *temp = new char[length+1];
strcpy(temp,find.c_str());
for(int i=0;i<length;i++)
if ( (pos = source.find(temp[i])) != string::npos )
return pos;
return -1;
}
int main()
{
string s;
double x,y;
char *opr=new char[1];
int pos;
Operand a,b,c;
while( getline(cin,s) )
{
pos = contains(s,"+-*/%^");
if (pos != -1)
{
if (pos != 0)
x = stod(s.substr(0,pos));
else
x = c.get();
string temp = s.substr(pos,1);
strcpy(opr, temp.c_str());
y = stod(s.substr(pos+1));
a.set(x);
b.set(y);
switch(*opr)
{
case '+': cout<<"="; c<<(c=a+b); cout<<endl; break;
case '-': cout<<"="; c<<(c=a-b); cout<<endl; break;
case '*': cout<<"="; c<<(c=a*b); cout<<endl; break;
case '/': cout<<"="; c<<(c=a/b); cout<<endl; break;
case '%': cout<<"="; c<<(c=a%b); cout<<endl; break;
case '^': cout<<"="; c<<(c=(a^b)); cout<<endl; break;
}
}
else
{
x=0; y=0; c.set(0);
system("cls"); //for windows
system("clear"); //for linux
}
};
return 0;
}
Output:
3.14*2
=6.28
+3
=9.28
5+3
=8
^2
=64
/8
=8
/0
=0
-10
=-10
With this article at OpenGenus, you must have the complete idea of how to develop a Calculator Console application in C++ Programming Language.