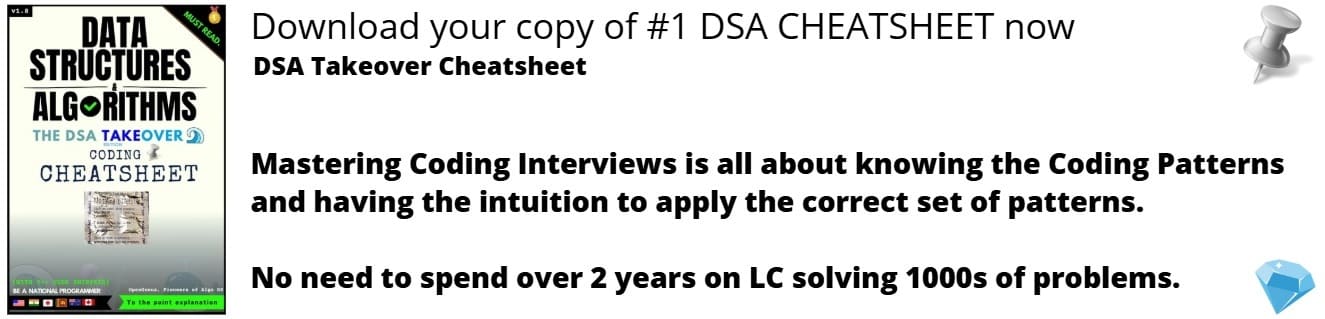
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to find the maximum, minimum and average of the marks of 4 subjects of different students using structure in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to find the maximum, minimum and average of 4 subjects using structures .
We will implement this problem in C Programming Language.
Approach to solve
Following is the approach to solve the problem:
- First we create a structure data type and declase all the variables.
- Next inside the main function we take the input of roll number, age and marks of 4 subjects.
- Then we find out the maximum and minimum marks using if statement.
- We also have to find the average of the marks by adding all the marks and dividing by 4.0.
- Then we print all the values.
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
struct data
{
int rollno;
int age;
int mark[4];
}
s;
int main()
{
double avg,total;
printf("enter roll");
scanf("%d",&s.rollno);
printf("enter age");
scanf("%d",&s.age);
printf("enter the marks");
for(int i=0;i<4;i++)
{
scanf("%d",&s.mark[i]);
}
int max=s.mark[0];
int min=s.mark[0];
for(int i=0;i<4;i++)
{
if(s.mark[i]>max)
max=s.mark[i];
if(s.mark[i]<min)
min=s.mark[i];
total+=s.mark[i];
}
avg=total/4.0;
printf("max is%d",max);
printf("min is %d",min);
printf("total is %lf",total);
return 0;
}
Output
Run the code as follows:
gcc code.c
./a.out
Following is the output of the program:
enter roll7
enter age18
enter the marks98
78
67
99
max is99min is 67total is 342.000000
With this article at OpenGenus, you must have the complete idea of how to find maximum, minimum and average of 4 subjects using structure in C Programming Language.