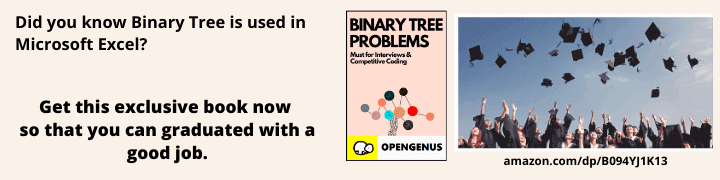
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In C++ programs, we will often have to convert arrays to other data types. One of these examples is converting from an array to a set.
This is used primarily when we want to remove duplicates from an array, but can also be used for a multitude of other reasons, including sorting a list, or generating faster insertion and deletion times using the set data structure.
In this article, we will discuss the three most common ways to convert an array into a set in C++. Lets jump in!
In short, the approach to convert array to set in C++ is:
std::set<int> s(std::begin(array), std::end(array));
Table Of Contents
- Problem Statement
- Naive Solution
- Range Solution
- Iterator Solution
Problem Statement
Suppose we have an array A, where A can be any array. In particular, let A be
int A[] = {4, 11, 5, 3, 1, 6};
The problem we want to solve is to convert this into an (ordered) set s. Once this happens, our set should contain the elements {1, 3, 4, 5, 6, 11}
.
Let's discuss some ways we can do this.
Naive Solution
A naive solution is to iterate through each element of the array, and add it to the set. Then, we can print out the set
#include <iostream>
#include <set>
int main()
{
int a[] = {4, 11, 5, 3, 1, 6};
std::set<int> s;
for (int i : a) {
s.insert(i);
}
for (int i : s) {
std::cout << i << " ";
}
std::cout << "\n";
return 0;
}
Output: 1 3 4 5 6 11
Time Complexity: O(N)
Space Complexity: O(N)
Range Solution
A more efficient solution is touse the range method of the set constructor to convert the array to a set, while specifying that the entire array should be converted. This lowers the space complexity of our solution.
#include <iostream>
#include <set>
int main()
{
int a[] = {4, 11, 5, 3, 1, 6};
int N = sizeof(a) / sizeof(a[0]);
std::set<int> s(A, A + N);
for (int i : s) {
std::cout << i << " ";
}
std::cout << "\n";
return 0;
}
Output: 1 3 4 5 6 11
Time Complexity: O(N)
Space Complexity: O(N)
Iterator Solution
The most efficient solution is to use an iterator to create a set using std::begin
and std::end
functions, which return an iterator to the beginning and end of the array, respectively. This lowers our time complexity, as iterators are faster than for loops, while also keeping the previous space complexity.
#include <iostream>
#include <set>
int main()
{
int a[] = {4, 11, 5, 3, 1, 6};
std::set<int> s(std::begin(a), std::end(a));
for (int i : s) {
std::cout << i << " ";
}
std::cout << "\n";
return 0;
}
Output: 1 3 4 5 6 11
Time Complexity: O(N)
Space Complexity: O(N)
Conclusion
Now that you know 3 of the most common ways (and the most optimal way) to convert an array to a set, you can solve a variety of problems relating to sets! See if you can find other applications of these methods. Thanks for reading the article!