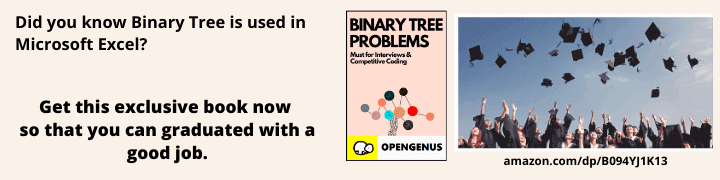
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented how to convert int to uint8_t considering explicit casting and overflow of uint8_t.
Table of contents:
- Convert int to uint8_t
- Consider Overflow
Convert int to uint8_t
One can convert an integer value to uint8_t value by assigning the int value to uint8_t value. One must ensure that the integer value is within the range of uint8_t and will not overflow.
int number1 = 12;
// int converted to uint8_t
uint8_t number2 = number1;
No explicit casting is required as we are downscaling a value to a smaller datatype.
// casting not needed
uint8_t number2 = (uint8_t) number1;
Following is the complete working C++ code:
#include <iostream>
int main() {
int number1 = 4;
uint8_t number2 = number1;
return 0;
}
Consider Overflow
One must ensure there is no overflow. int is of 32 bits while uint8_t is of 8 bits. The range of uint8_t is 0 to 2^8 - 1 (both included).
Range of uint8_t = 0 to 255
So, if the int value is outside this range, the value will overflow. Note, there will not be any compiler or runtime warning or error.
Following is the complete working C++ code considering overflow:
#include <iostream>
int main() {
int number1 = 300;
uint8_t number2 = number1;
if(number1 > 255 || number1 < 0) {
std::cout << "Conversion from int to uint8_t has overflowed" << std::endl;
// Handle it
}
return 0;
}
With this article at OpenGenus, you must have the complete idea of how to convert an integer (int) to uint8_t.