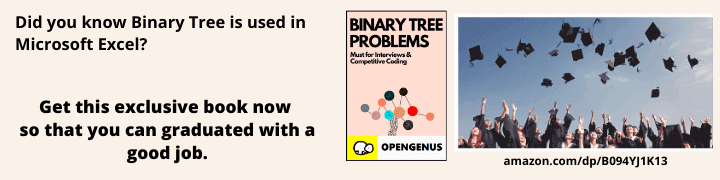
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the reason uint8_t cannot be printed with std::cout and multiple fixes that can be used to print uint8_t values.
Table of contents:
- Problem with uint8_t
- Fix 1: Convert to unsigned
- Fix 2: Using + operator
- Fix 3: operator<<()
- Fix 4: number + 0
- Fix 5: Use static_cast
Problem with uint8_t
uint8_t is a typedef for unsigned char in C++. Due to this, ostream class (used for cout) prints the character having ascii numeric value (in this case 4).
Hence, it is not possible to print the numeric value of a uint8_t variable directly. There are work-arounds which we have presented in this article at OpenGenus.
#include <iostream>
int main() {
uint8_t number = 4;
std::cout << "Number=" << number << std::endl;
return 0;
}
Output:
Number=
The uint8_t number does not get printed or a random ASCII character is printed.
Fix 1: Convert to unsigned
The fix is to convert number of datatype uint8_t to unsigned number using the unsigned() method.
unsigned(number)
The working C++ code is as follows:
#include <iostream>
int main() {
uint8_t number = 4;
std::cout << "Number=" << unsigned(number) << std::endl;
return 0;
}
Output:
Number=4
Fix 2: Using + operator
Adding + operator before any variable (such as of datatype uint8_t) will return the numeric printable value instead of unprinted ASCII character.
+number
The working C++ code is as follows:
#include <iostream>
int main() {
uint8_t number = 4;
std::cout << "Number=" << +number << std::endl;
return 0;
}
Output:
Number=4
Fix 3: operator<<()
operator<<() is an overloaded non-member function of std::ostream which can be called explicitly to consider any character as an integer. As uint8_t is actually a character, this approach works.
std::cout.operator<<(number);
The working C++ code is as follows:
#include <iostream>
int main() {
uint8_t number = 4;
std::cout << "Number="
std::cout.operator<<(number);
return 0;
}
Output:
Number=
4
Fix 4: number + 0
In this fix, we add number 0 to our uint8_t variable at the time of printing. This will convert the result to integer and the value will not change as well. One thing to note is that this will convert uint8_t to int32_t so the memory requirements will increase.
This approach works for other datatypes like int8_t as well.
std::cout << "Number=" << number + 0 << std::endl;
The working C++ code is as follows:
#include <iostream>
int main() {
uint8_t number = 4;
std::cout << "Number=" << number + 0 << std::endl;
return 0;
}
Output:
Number=4
Fix 5: Use static_cast
One can use static_cast<unsigned int>
to convert uint8_t to an unsigned integer which can be printed as usually. We can modify the std namescape to consider all unsigned character as unsigned integer.
static_cast<unsigned int>(number)
The working C++ code with an alternative to std namespace using static_cast is as follows:
#include <cstdint>
#include <iostream>
#include <typeinfo>
namespace fixed_std {
inline std::ostream &operator<<(std::ostream &os, char c) {
return std::is_signed<char>::value ? os << static_cast<int>(c)
: os << static_cast<unsigned int>(c);
}
inline std::ostream &operator<<(std::ostream &os, unsigned char c) {
return os << static_cast<unsigned int>(c);
}
}
int main() {
uint8_t i = 4;
std::cout << "Number=" << number << std::endl;
fixed_std::cout << "Number=" << number << fixed_std::endl;
}
Output:
Number=
Number=4
With this article at OpenGenus, you must have a complete idea of how to print uint8_t correctly.