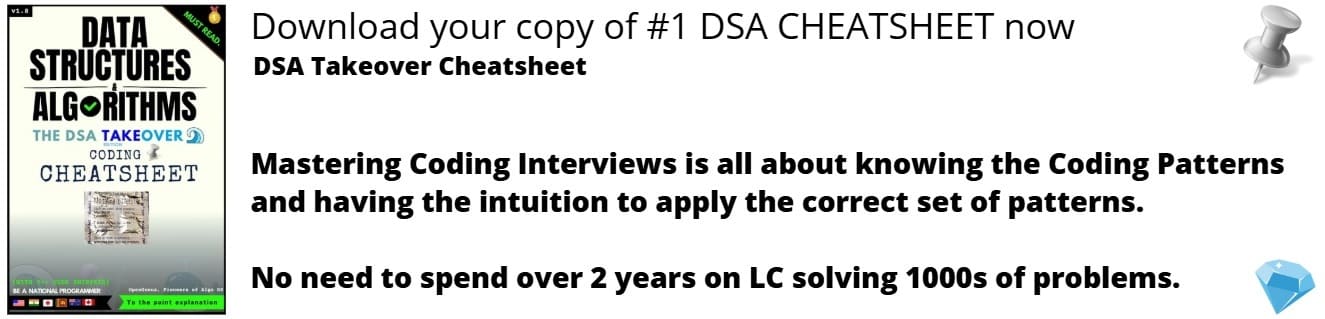
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to use Lambda in Python to replicate the behavior of for loop and how to use for loop within lambda.
Table of contents:
- Use for loop within lambda
- Replace for loop with lambda
- Concluding Note
Let us understand how lambda can be used in this case in Python.
Use for loop within lambda
Lambda can include only one line expression and for loop has multiple lines (at least 3) in Python so at first, it may seem to be difficult to use it in Lambda.
The trick is to write for loop in one line expression which can be done as follows:
[print(x) for x in listx]
As this is a one line expression, we can directly include this in a lambda. Following Python code traverses through a list of integers using lambda which in turn uses for loop:
list = [91, 12, 63, 5]
f = lambda listx: [print(x) for x in listx]
f(list)
Output:
91
12
63
5
The output is as expected.
Replace for loop with lambda
Moving forward, you may want to replace a for loop which a corresponding lambda (without using for loop). This is also possible either using the lambda recursively or using reduce built-in function in Python.
Following Python code uses a lambda along with reduce function to recursively traverse through a list just like a for loop:
from functools import reduce
list = [91, 12, 63, 5]
f = lambda x, y: print(y) if x is None else print(x, y)
a = reduce(f, list)
Output:
91 12
63
5
Another alternative is to use the lambda recursively. This is demonstrated in the following Python code:
list = [59, 47, 7, 17, 45]
f = lambda l: print(l[0]) if len(l) == 1 else print(l[0]) + f(l[1:])
sum = f(list)
Output:
59
47
7
17
45
Go through this Python code to get the sum of all elements of a list using lambda just like for loop:
list = [59, 47, 7, 17, 45]
f = lambda l: l[0] if len(l) == 1 else l[0] + f(l[1:])
sum = f(list)
print(sum)
Output:
175
Concluding Note
With this article at OpenGenus, you must have the complete idea of how to use for loop with lambda and even replace for loop with lambda.
Lambda is a powerful feature in Python. Use it wisely.