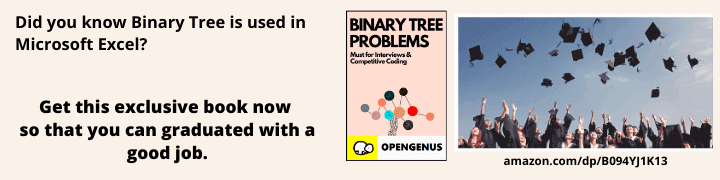
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will convert integer to string in C++ using different methods.
Table of Contents:
- Integer to string conversion by to_string() method.
- Integer to string conversion by stringstream class.
- Integer to string conversion by using boost::lexical_cast.
Method 1. By to_string() method
In C++, the std::string to_string( int value ) function is a built in function, which simply returns the integer value to string after conversion.
It is defined in the header file <string>
We can also use to_string() method for float,long and double conversion.
Syntax
to_string(val)
Parameters
value - a numeric value
Return value
string - holding numeric value as string
Time Complexity
time complexity of to_string() method is O(1)
Example of to_string()
#include <iostream>
#include <string>
int main() {
int num = 10;
//converting to string
std::string str = std::to_string(num);
std::cout << str;
}
Output
10
Method 2. By stringstream class
In this method we use object of input/output class
- istringstream(input string stream)
- ostringstream(output string stream)
The stringstream class is included in the <sstream>
header file.
"<<" this operator is used to send data to stream object.
str() method is used to retrive value from object and assign it to string variable.
Time Complexity
time complexity of to_string() method is O(N)
N is length of string.
Auxiliary Space
time complexity of to_string() method is O(N)
Example of stringstream conversion
#include <iostream>
#include <sstream> //header file for sstream
using namespace std;
int main() {
int num = 1234 ;
ostringstream ostr ; //declaring output stringstream
ostr<<num ; //sending integer as stream into output stringstream
string s = ostr.str(); // using str() method for conversion of integer to string
cout<<"num as a string - "<<s;
}
Output
num as a string - 1234
Method 3. By boost::lexical_cast method
This boost::lexical_cast method is defined in the library boost/lexical_cast.hpp
It can perform interconversion of different data types like int,float,double and string.This is included in<boost/lexical_cast.hpp>
header file.
Note: You need to install the Boost libraries for conversion.
(For more reference visit boost.org)
Syntax
boost::lexical_cast<data-type>(argument)
data-type: This is the type to which argument will be converted. To convert an integer to a string, you should specify the data type as a string.
argument: This is the value that will be converted. To convert an integer to a string, the argument should be an integer value.
Example of boost::lexical_cast conversion
#include <bits/stdc++.h>
#include <boost/lexical_cast.hpp> // for lexical_cast() method
using namespace std;
int main()
{
//initialize the integer
int num = 1234;
// convert int to string
string str = boost::lexical_cast<string>(num);
cout << "num after string conversion : " << str << "\n";
}
Output
num after string conversion : 1234
The simplest ways to convert integer to string is by to_string() method other than that we can also use sstream and boost::lexical_cast method.
With this article at OpenGenus, you must have the complete idea of how to convert integer to string in C++.