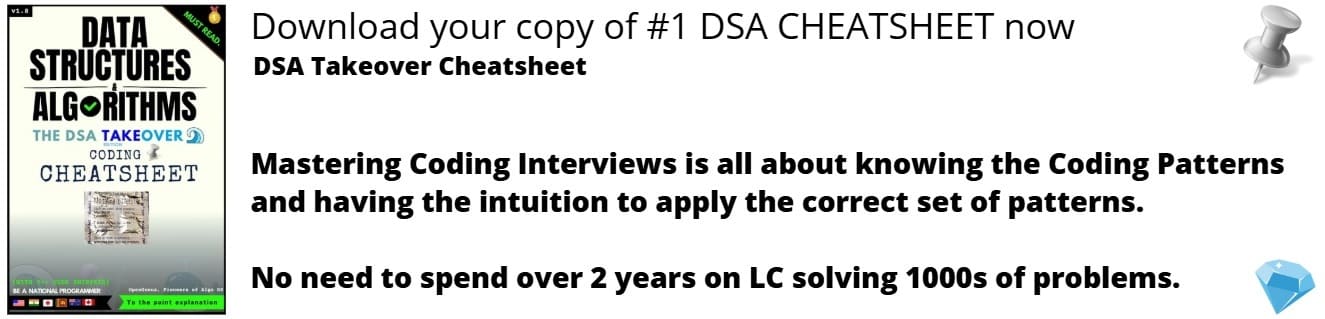
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will discuss about the vector::capacity() function in C++ and why it is essential when working with vectors.
Table of Contents:
- Introduction to vector::capacity() function
- Syntax and Time Complexity of the vector::capacity() function
- Examples
1. Introduction to vector::capacity() function
In C++, the vector::capacity() function is a built in function, which simply returns the maximum capacity that is allocated by the vector.
2. Syntax and Time complexity of the vector::capacity() function
vector_name.capacity()
vector_name
is simply where you put the name of your vector and this function has a time complexity of constant O(1)
. Let's do some examples for when we use the vector::capacity() function.
The return type of vector::capacity() is size_type.
size_type as a typedef to std::allocator<T>::size_type
which is also defined as size_t. This is a generalization so the return value can be stored directly in variable of datatypes int, float and others.
3. Examples
Example 1 (Comparing size and capacity of a vector):
This C++ code compares size and capacity of a vector:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> v;
int size;
cin >> size;
for (int i = 0; i < size; i++) {
v.push_back(i); // Pushes i into v
}
cout << endl << "Current size of v: " << size;
cout << endl << "Maximum size of v: " << v.capacity();
}
Input:
10
Output:
Current Size of v: 10
Maximum size of v: 16
The maximum size will always be bigger than or equal to the current size, depending on the size that is inputted by the user. Let's do another example, but this time, let's look at the capacity of an Auditorium.
Example 2 (Auditorium Capacity)
In this example, we were able to use the vector::capacity() function to find the max capacity of the auditorium and we went an extra step further, where we were able to equate the amount of people until max capacity is reached.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> people;
int auditorium_count;
cout << "How many people are currently in the Auditorium: ";
cin >> auditorium_count;
for (int i = 1; i <= auditorium_count; i++) {
people.push_back(i); // Pushes i into people
}
cout << endl << "Current size of the Auditorium: " << auditorium_count;
cout << endl << "Max capacity of the Auditorium: " << people.capacity();
cout << endl << "Amount of people until max capacity is reached: " << people.capacity() - auditorium_count;
}
Input:
How many people are currently in the Auditorium: 23
Output:
Current size of the Auditorium: 23
Max capacity of the Auditorium: 32
Amount of people until max capacity is reached: 9
With this article at OpenGenus, you must have the complete idea of vector::capacity() function in C++.