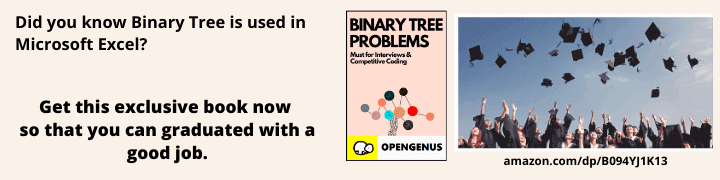
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In Java, arrays and lists are two fundamental data structures. Arrays have a fixed length and are used to store a collection of elements of the same type. Lists, on the other hand, are dynamic and can grow or shrink as needed to store a collection of elements. In Java, it is often necessary to convert an array to a list or vice versa. In this article, we will explore several ways to convert an array to a list in Java.
Pre-requisites:
Table of contents:
- Using the Arrays.asList() method
- Using a for loop to add elements to a new list
- Using the Stream API
- Using the Collection.addAll() method
- Conclusion
Using the Arrays.asList() method
The Arrays.asList() method is a convenient way to convert an array to a list. This method takes an array as an argument and returns a fixed-size list backed by the original array. Here is an example of using this method:
package javaapplication;
import java.util.*;
public class JavaApplication {
public static void main(String args[])
{
String[] array = {"one", "two", "three"};
List<String> list = Arrays.asList(array);
System.out.println(list);
}
}
Output:
[one, two, three]
This code creates a new array containing three elements, and then converts it to a list using the Arrays.asList() method. The resulting list is backed by the original array, which means that any changes to the list will also affect the array, and vice versa. This method is convenient and efficient, but it has one limitation: the resulting list is fixed-size, which means that you cannot add or remove elements from it.
Using a for loop to add elements to a new list
Another way to convert an array to a list is to create a new list and add each element of the array to it using a for loop. Here is an example of using this method:
package javaapplication;
import java.util.*;
public class JavaApplication {
public static void main(String args[])
{
String[] array = {"one", "two", "three"};
List<String> list = new ArrayList<>();
for (String element : array) {
list.add(element);
}
System.out.println(list);
}
}
Output:
[one, two, three]
This code creates a new ArrayList, then iterates over each element of the array using a for loop and adds it to the list. The resulting list is a dynamic list that can grow or shrink as needed. This method is flexible, and you can use it to convert arrays of any type to lists.
Using the Stream API
In Java 8 and later, you can use the Stream API to convert an array to a list. This method is similar to using a for loop, but it is more concise and uses functional programming concepts. Here is an example of using this method:
package javaapplication;
import java.util.*;
import java.util.stream.*;
public class JavaApplication {
public static void main(String args[])
{
String[] array = {"one", "two", "three"};
List<String> list = Arrays.stream(array)
.collect(Collectors.toList());
System.out.println(list);
}
}
Output:
[one, two, three]
This code creates a new Stream from the array using the Arrays.stream() method, then collects the elements of the stream into a list using the Collectors.toList() method. This method is concise and easy to read, and it can be used to convert arrays of any type to lists.
Using the Collection.addAll() method
Finally, you can use the Collection.addAll() method to convert an array to a list. This method is similar to using a for loop, but it is more concise and uses a built-in method to add all the elements of the array to the list. Here is an example of using this method:
package javaapplication;
import java.util.*;
public class JavaApplication {
public static void main(String args[])
{
String[] array = {"one", "two", "three"};
List<String> list = new ArrayList<>();
Collections.addAll(list, array);
System.out.println(list);
}
}
Output:
[one, two, three]
This code creates a new ArrayList, then adds all the elements of the array to the list using the Collections.addAll() method. This method is concise and easy to read, and it can be used to convert arrays of any type to lists.
Conclusion
In this article at OpenGenus, we have explored several ways to convert an array to a list in Java. The Arrays.asList() method is a convenient and efficient way to convert an array to a fixed-size list. Using a for loop or the Stream API, you can create a dynamic list that can grow or shrink as needed.
The Collection.addAll() method is a concise and built-in way to add all the elements of an array to a list. Each method has its advantages and disadvantages, and the choice of method depends on the requirements of your application.
One advantage of using the Arrays.asList() method is that it is a convenient way to create a fixed-size list that is backed by the original array. This method is efficient and avoids the overhead of creating a new list. However, the resulting list cannot be modified, and any attempt to modify it will result in an UnsupportedOperationException.
Using a for loop or the Stream API, you can create a dynamic list that can grow or shrink as needed. This method is more flexible and can be used to convert arrays of any type to lists. However, it requires more code and may be less efficient than using the Arrays.asList() method.
The Collection.addAll() method is a concise and built-in way to add all the elements of an array to a list. This method is efficient and easy to read, and it can be used to convert arrays of any type to lists. However, it requires a new list to be created, and the resulting list is not backed by the original array.
In conclusion, there are several ways to convert an array to a list in Java, and the choice of method depends on the requirements of your application. The Arrays.asList() method is a convenient way to create a fixed-size list, while using a for loop or the Stream API can create a dynamic list that can grow or shrink as needed. The Collection.addAll() method is a concise and built-in way to add all the elements of an array to a list, but it requires a new list to be created.