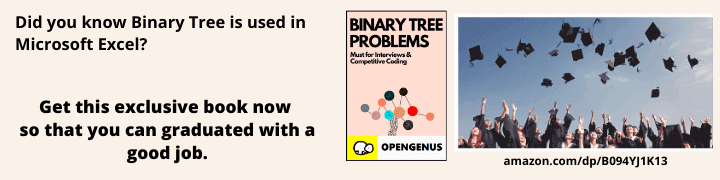
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will see 3 different ways to convert a String to Char Array in Java Programming Language.
Table of contents:
- String in Java
- Char array in Java
- Difference between String and char in Java
- Need of conversion from string to an char array
- String to Char Array
First, we will take a look at basic concepts like String and Char array and also the need to convert String to Char array.
String in Java
String in Java is an object that represent sequence of character. String class is automatically imported as it belongs to java.lang package.
//Using New keyword
String s = new String("OpenGenus");
//Using String Literal
String s = "OpenGenus";
Char array in Java
Char is a primitive datatype in java. Char Array is a data structure which can store a Fixed number of characters.
char carray[] = new char;
Inputs can be taken using index in array
char [] carray = {'A','B','C'};
Array can also be declared and initialized at the same time.
Difference between String and char in Java
String and char array are different on several parameters. Some are Discussed below-
- String in java is a class, whose object is created every time a string is declared whereas char array is a sequential collection of char datatype.
- String in java are immutable i.e whenever a change is applied to a string in java , a new String object is created. Char array are mutable.
- Built-in function like concat, charAt(),equals() can be used on java string. Char being a datatype does not provide built-in function.
Need of conversion from string to an char array
- String manipulation- Manipulation of individual characters in a string like replacing a character in char array is more efficient than in String functions.
String message = "Mello, world!";
char[] chars = message.toCharArray();
chars[0] = 'H';
String modifiedMessage = new String(chars);
System.out.println(modifiedMessage);
- Performance- Several operations over String are more efficiently performed if they were in char array. For Example- Like iterations over large array.
String largeString = "This is a very large string...";
char[] chars = largeString.toCharArray(); //
for (int i = 0; i < chars.length; i++) {
// Perform some operation on the char array
}
- Interoperability- Some APIs and libraries may require char input/output. hence, conversion becomes necessary.For example java.util.Scanner.
String to Char Array
There are 3 different ways to convert a String to a char array:
- Using Built-In function toChharArray()
- Using For loop and charAt()
- Using getBytes method
We will dive into each method.
- Using Built-In function toChharArray()-
The 'toCharArray()' method is provide by the String class in java. It inputs an String object and return a char array representation of the string. This is the simplest way to convert string to char array as it does not involve looping.
String s = "Hello World"
char[] charArray = str.toCharArray();
- Using For loop and charAt()-
Another way to convert a String to a char array is to use a for loop to iterate over all the character in the string using charAt() and add it to a char array. This Approach is usually used when some other operation are to be performed on the string during conversion.
String s = "Hello World";
char[] charArray = new char[str.length()];
for (int i = 0; i < str.length(); i++) {
charArray[i] = str.charAt(i);
}
- Using getBytes method-
This methods is also provided by string class in java. It first converts the string to byte array which can further be converted into char array. This method is rather roundabout and mat not give correct result everytime as the string may contain non-ASCII character sets.
String s = "Hello World"
byte[] byteArray = str.getBytes();
char[] charArray = new String(byteArray).toCharArray();
The first two methods are more commonly used in practice.
With this article at OpenGenus, you must have the complete idea of how to convert a string to char array in Java.