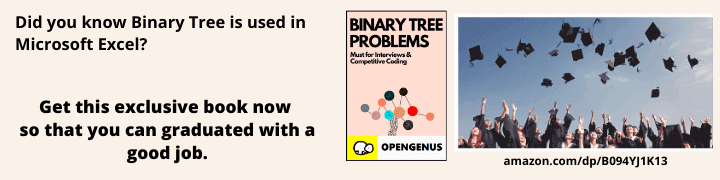
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
There are 9 different error signals in C++ Programming Language such as SIGABRT. Each signal handles a different category of runtime error. We have listed all types of error signals in C++ along with C++ code snippets.
Table of content-
1. Introduction to signals
2. different types of c++ signals.
3. signal handling programs.
Intoduction to signals -
Signals are the Interruptions that come in our program which are delivered to our program (or process) that runs on an operating system and pauses our running program (or process) to terminate prematurely.So signals are interruptions which stops an operating system from attending another task,to solve this problem signals needed to be handled inside our program.
There are many signals which are not caught by our program , The signals are defined in c++ csignal header file .below are the lists of c++ signals along with their description.
Different types of c++ signals-
There are 9 different signals in C++:
- SIGINT (signal interrupt)
- SIGABRT (Abort)
- SIGTERM (signal termination)
- SIGSEGV (signal segmentation violation)
- SIGBUS (signal bus)
- SIGFPE (floating point exception)
- SIGTSTP (Stop temporarily)
- SIGILL (signal illegal instruction)
- SIGALRM (signal alarm)
SIGINT (signal interrupt)
SIGINT is an interrupt signal sent to a program by a user's request, it's default action is to terminate a program but it can be overriden to handle different cases as well . it catches the external interrupt, usually initiated by the user(e.g. ctrl + c).
below is its implementation-
#include<bits/stdc++.h>
using namespace std;
void siginthandler(int param)
{
cout<<"User pressed Ctrl+C \n";
exit(1);
}
int main()
{
signal(SIGINT, siginthandler);
while(1);
return 0;
}
Output:
User pressed Ctrl+C
SIGABRT (Abort)
SIGABRT is generally used to terminate a program in case of errors, termination of the program in case of some critcal errors is handled by this signal,
abort() sends the calling process the SIGABRT signal.
abort() is usually called by library functions libc which detect errors like different types of heap corruptions in a program and more.
Below is its implementation-
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGABRT, signal_handler);
while(++count) {
cout << "Hey there this is SIGABRT error handling..." << endl;
if( count == 16 )
raise(SIGABRT);
}
return 0;
}
}
Output:
Hey there this is SIGABRT error handling...
Hey there this is SIGABRT error handling...
Hey there this is SIGABRT error handling...
Hey there this is SIGABRT error handling...
Hey there this is SIGABRT error handling...
Hey there this is SIGABRT error handling...
Interrupt signal is (6).
SIGTERM (signal termination)
SIGTERM signal is general signal used in termination of a running process or program.This signal can be handled and can be ignored. it sends request to the program for termination of current running process (or program).It is generated by default in shell command kill.
In C++ code, objects with static storage duration are destroyed in a standard program termination. This will not happen in an abnormal program termination caused by raise( SIGTERM ).
Implementation of SIGTERM in c++ -
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGTERM, signal_handler);
while(++count) {
cout << "Hey there this is SIGTERM error handling..." << endl;
if( count == 16 )
raise(SIGTERM);
}
return 0;
}
Output:
Hey there this is SIGTERM error handling...
Hey there this is SIGTERM error handling...
Hey there this is SIGTERM error handling...
Hey there this is SIGTERM error handling...
Hey there this is SIGTERM error handling...
Hey there this is SIGTERM error handling...
Interrupt signal is (15).
SIGSEGV (signal segmentation violation)
SIGSEGV Segmentation fault occurs when our program tries to access a memory location that is not allowed to access.
This signal handles interrupts related to invalid memory access.
this error occurs due to using uninitialized pointer, overflowing buffer,using a null pointer etc.
Its implementation is given below-
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGSEGV, signal_handler);
while(++count) {
cout << "Hey there this is sigsegv error handling..." << endl;
if( count == 17 )
raise(SIGSEGV);
}
return 0;
}
output:
Hey there this is sigsegv error handling...
Hey there this is sigsegv error handling...
Hey there this is sigsegv error handling...
Hey there this is sigsegv error handling...
Hey there this is sigsegv error handling...
Hey there this is sigsegv error handling...
Hey there this is sigsegv error handling...
Interrupt signal is (11).
SIGBUS (signal bus)
Bus error indicates that there is access to an invalid address. This signal notifies that the program tried to access an invalid address and halts the program. bus error is reading data form bad-aligned memory or memory address that doen't exist anymore.
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGBUS, signal_handler);
while(++count) {
cout << "Hey there this is sigbus error handling..." << endl;
if( count == 15 )
raise(SIGBUS);
}
return 0;
}
Output:
Hey there this is sigbus error handling...
Hey there this is sigbus error handling...
Hey there this is sigbus error handling...
Hey there this is sigbus error handling...
Hey there this is sigbus error handling...
Interrupt signal is (7).
SIGFPE (floating point exception)
The SIGFPE signal handles floating point exceptions. SIGFPE signal is raised by a program whenever a floation point exception occurs and calls the signal handler suroutione.some floating point examples that signaled by a program :
- Invalid Operation
- Division by Zero
- Overflow
- Underflow
Below is its Implementation-
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGFPE, signal_handler);
while(++count) {
cout << "Hey there this is SIGFPE error handling..." << endl;
if( count == 16 )
raise(SIGFPE);
}
return 0;
}
Output:
Hey there this is SIGFPE error handling...
Hey there this is SIGFPE error handling...
Hey there this is SIGFPE error handling...
Hey there this is SIGFPE error handling...
Hey there this is SIGFPE error handling...
Hey there this is SIGFPE error handling...
Interrupt signal is (8).
SIGTSTP (Stop temporarily)
The SIGTSTP signal is an interactive stop signal.This signal can be handled and ignored.It is also known as terminal stop. This signal generated when the user types the character normally (ctrl-z) on terminal.The SIGTSTP signal is sent to a process to request it to stop temporarily.
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGTSTP, signal_handler);
while(++count) {
cout << "Hey there this is SIGTSTP error handling..." << endl;
if( count == 14 )
raise(SIGTSTP);
}
return 0;
}
Output:
Hey there this is SIGTSTP error handling...
Hey there this is SIGTSTP error handling...
Hey there this is SIGTSTP error handling...
Hey there this is SIGTSTP error handling...
Interrupt signal is (20).
SIGILL (signal illegal instruction)
This signal is used to detect an illegal command or instruction.It can not be Ignored.SIGILL signal is raised when there is an attempt to execute an illegal, unknown instruction and causes program termination.It may cause by calling a function which is not in program load module.
below is its implementation-
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGILL, signal_handler);
while(++count) {
cout << "Hey there this is SIGILL error handling..." << endl;
if( count == 14 )
raise(SIGILL);
}
return 0;
}
Output:
Hey there this is SIGILL error handling...
Hey there this is SIGILL error handling...
Hey there this is SIGILL error handling...
Hey there this is SIGILL error handling...
Interrupt signal is (4).
SIGALRM (signal alarm)
This process uses the alarm() function to generate SIGALRM signal.It can be defined as a wake up call by a process from operating system at some time in near future using alarm() function,After the no. of seconds ended specified in seconds parameter ,alarm() function generate SIGALRM signals.
Below is its implementation-
#include <bits/stdc++.h>
using namespace std;
void signal_handler( int num ) {
cout << "Interrupt signal is (" << num << ").\n";
exit(num);
}
int main () {
int count = 10;
signal(SIGALRM, signal_handler);
while(++count) {
cout << "Hey there this is SIGALRM error handling..." << endl;
if( count == 16 )
raise(SIGALRM);
}
return 0;
}
Output:
Hey there this is SIGALRM error handling...
Hey there this is SIGALRM error handling...
Hey there this is SIGALRM error handling...
Hey there this is SIGALRM error handling...
Hey there this is SIGALRM error handling...
Hey there this is SIGALRM error handling...
Interrupt signal is (14).
Examples of Signal handling programs
The Signal() function-
Syntax:
void (*signal (int sig, void (*func)(int)))(int);
The signal-handling library of C++ provides a function signal to trap interrupts in our program.
The first argument is an integer, representing the signal number and second is the pointer to a signal handling function. The signal handling function is of the void type.
#include<bits/stdc++.h>
using namespace std;
void signalHandler( int signum ) {
cout << "Interrupt signal (" << signum << ") received by program.\n";
exit(signum);
}
int main () {
signal(SIGINT, signalHandler);
while(1) {
cout << "hey there this is signal error program" << endl;
}
return 0;
}
Output:
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
hey there this is signal error program
Interrupt signal (2) received by program.
The implementation for different signal errors like SIGINT, SIGABRT, SIGFPE, SIGILL etc. are handle by below raise() function.
The raise() Function
We can generate signals by function raise(), which takes an integer signal number as an argument and has the following syntax.
int raise (signal sig);
Here, sig is the signal sig can send any of the signals: SIGINT, SIGABRT, SIGFPE, SIGILL etc.
Following is an example-
#include<bits/stdc++.h>
using namespace std;
void signalHandler( int signum ) {
cout << "Interrupt signal (" << signum << ") received.\n";
exit(signum);
}
int main () {
int i = 0;
signal(SIGINT, signalHandler);
while(++i) {
cout << "hey there " << endl;
if( i == 5 ) {
raise( SIGINT);
}
}return 0;
}
Output:
hey there
hey there
hey there
hey there
hey there
Interrupt signal (2) received.
With this article at OpenGenus, you must have the complete idea of different signal errors in C++.