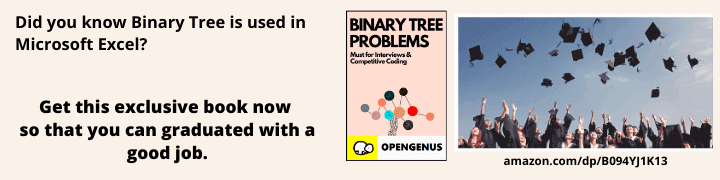
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Problem statement
Print the following pattern and show its implementation in C programming language using escape sequences like horizontal and vertical tab.
abz
pqr
mno
qrs
tvz
Solution
- First we normally print abz then print a vertical tab(\v)
- for example, we print abz, now the cursor is at the position after z. If we print \v then the cursor will go vertically down and the next thing will be printed from there onwards.
- We repeat the same thing for pqr and mno that is add \v at the end of printing it separately.
- Now for qrs, the cursor is vertically below o but one place ahead. To print qrs from the right place we have to come back 6 spaces. For that we use\b 6 times then \n to go to the next line.
- Since we used \n the cursor is now at the beginning of the line so we can now simply print tvz.
Implementation
Following is the implementation of the program in C programming language.
#include <stdio.h>
int main()
{
printf("abz\v");
printf("pqr\v");
printf("mno\b\b\b\b\b\b");
printf("\vqrs\n");
printf("tvz");
return 0;
}
Compile and run the above code as follows:
gcc code.c
./a.out
The output will be as follows:
abz
pqr
mno
qrs
tvz
With this article at OpenGenus, you must be able to print this pattern using escape sequences in C Programming.