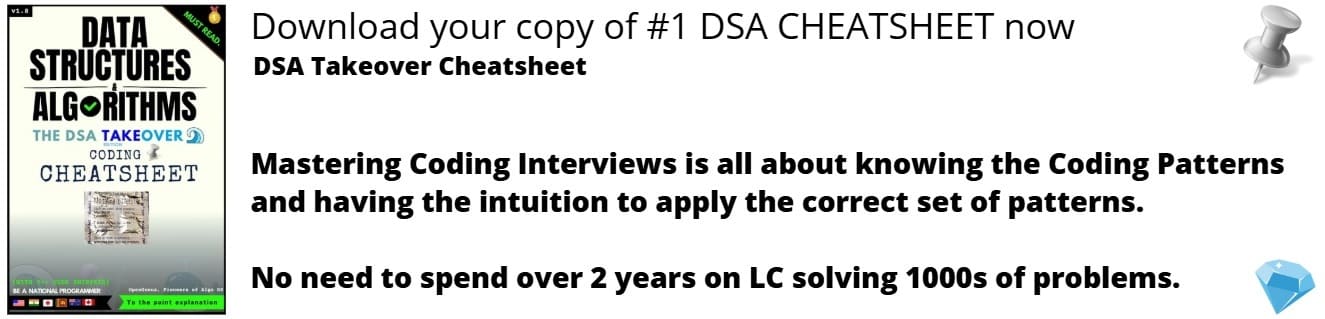
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the fabs and abs functions in C++ Programming Language which are used to get the absolute value of different numeric values. We have compared the differences between fabs and abs as well.
CONTENTS:
- Fabs
- Abs
- Fabs and Abs Parameters
- Syntax of Fabs and Abs
- Example of Fabs and Abs
- Application of fabs and Abs
- Complexity of Fabs and Abs
- Difference between Fabs and Abs
What is Fabs and Abs?
Fabs
The Fabs function in the C++ returns the absolute value of the argument.
The fab() function takes a single argument, a whose absolute value has to be returned.
It is defined in cmath header file.
Mathematically, it is represented as: fabs(num) = |num|
Abs
Abs in C++ used to compute the absolute value of a number.
The function takes a single argument, which is the number for which the absolute value is to be calculated, and it returns the absolute value of that number as its result.
It is defined in cstdlib
The abs() function is declared as:
int abs(int x);
Fabs and Abs Parameters
The fabs() function takes the following parameter:
- num -
a floating point number whose absolute value is returned. It can be of the following types:
- double
- float
- long double
- fabs() Return Value -
the fabs() function returns:
the absolute value of num i.e. |num|
The Abs() function takes the following parameter:
- x: The value whose absolute value is to be determined.
It can be of 3 data types :
- int
- long int
- long long int
- Return value
It returns the absolute value of x.
Syntax of fabs and Abs in C++
Synatx of Fabs:
- double fabs(double x);
- float fabs(float x);
- long double fabs(long double x);
Syntax of Abs:
- abs( int num);
- abs(long int num);
- abs(long long int num);
C++ code example of Fabs and Abs
Fabs()
include <iostream>
include <cmath>
using namespace std;
int main() {
double num = -10.25, result;
result = fabs(num);
cout << "fabs(" << num << ") = |" << num << "| = " <<result;
return 0;
}
Output:
fabs(-10.25) = |-10.25| = 10.25
abs()
include <stdio.h>
include <stdlib.h>
int main ()
{
int n,m;
n=abs(23);
m=abs(-11);
printf ("n=%d\n",n);
printf ("m=%d\n",m);
return 0;
}
Output:
n=23
m=11
Application of Fabs and Abs in C++
fabs and abs functions in C++ are typically used in mathematical and computational applications, such as numerical analysis and computer programming.
The concept of absolute value, which is what these functions calculate, is used in many areas of mathematics and can be applied to various real-life scenarios.
For example, in finance, the absolute value is used to calculate the magnitude of a gain or loss, such as calculating the percentage change in the value of a stock. In physics and engineering, absolute value is used to calculate the magnitude of physical quantities such as displacement, velocity, and acceleration.
In daily life, you might use the concept of absolute value to calculate the difference between two quantities, such as the difference in temperature between two cities.
For example, if the temperature in City A is -5 degrees Celsius, and the temperature in City B is 10 degrees Celsius, the absolute value of the difference between these temperatures would be 15 degrees Celsius.
Complexity of fabs and Abs
The complexity of the fabs and abs functions in C++ depends on the underlying implementation, which can vary between different compilers and platforms.
In general, both fabs and abs have a constant time complexity, which means that the execution time does not depend on the size of the input.
For fabs, the function is applied to a floating-point number and it's just a matter of extracting the bits of the number and returning the same number with the sign bit set to positive.
For abs, the function is applied to an integer, and it's just a matter of checking the sign of the number and returning the same number with the sign bit set to positive if it's negative, or the number itself otherwise.
Therefore, the time complexity of both fabs and abs is considered to be O(1), which is considered to be very efficient.
It is worth noting that, when working with large numbers or arrays, the constant time complexity of fabs and abs functions could lead to a significant performance gain when compared to other algorithms that have a higher time complexity.
Difference between fabs and Abs
Following are the 4 differences between fabs and abs:
- The main difference between fabs and abs in C++ is the type of input they accept and the type of output they return.
- fabs is a function that takes a single floating-point number as input, and returns the absolute value of that number as a floating-point number.
- abs is a function that takes a single integer as input, and returns the absolute value of that number as an integer.
- Abs is a part of the 'cstdlib' library and Fabs is a part of the 'cmath' library.
With this article at OpenGenus, you must have the complete idea of fabs() and abs() is C++ Programming Language.