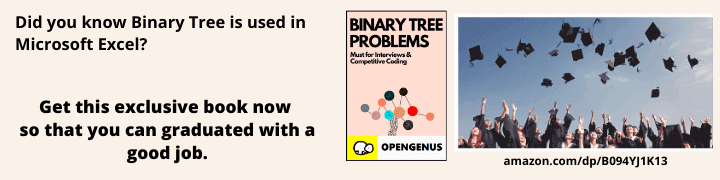
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn how to generate both a hollow and filled square pattern in C++.
Table of contents:
- Introduction to Problem Statement
- Solving the problem
- Implementing the Solution
- Time and Space Complexity
Introduction to Problem Statement
Given the side of a square, write a C++ implementation to generate hollow and filled square pattern.
Solving the problem
A hollow square pattern is one where the border of the square is drawn, but the inside is left empty. On the other hand, a filled square pattern is one where both the border and the inside of the square are filled with a particular character or symbol.
Implementing the Solution
In C++, these patterns can be generated using loops and conditional statements.
To generate a hollow square pattern in C++, we use nested loops to iterate over the rows and columns of the square and print the appropriate characters based on the position of each element. For a filled square pattern, we can modify the logic slightly to fill the entire square with the specified character.
Filled Square Pattern
Following is the complete C++ program to print Filled Square Pattern:
#include <iostream>
int main() {
int n = 5; // Side of the square
for(int i = 0; i < n; ++i){
for(int j = 0; j < n; ++j){
std::cout << "#";
}
std::cout << std::endl;
}
return 0;
}
Output:
#####
#####
#####
#####
#####
Hollow Square Pattern
Following is the complete C++ program to print Hollow Square Pattern:
#include <iostream>
int main() {
int n = 5; // Side of the square
for(int i = 0; i < n; ++i){
if(i == 0 || i == n-1){
for(int j = 0; j < n; ++j){
std::cout << "#";
}
}
else{
for(int j = 0; j < n; ++j){
if(j == 0 || j == n-1){
std::cout << "#";
}
else std::cout << " ";
}
}
std::cout << std::endl;
}
return 0;
}
Output:
#####
# #
# #
# #
#####
Time and Space Complexity
For a square of size n, the time complexity of generating a hollow square pattern using nested loops is O(n^2), as we need to iterate over each element in the square and print the appropriate characters. Similarly, the time complexity of generating a filled square pattern using nested loops is also O(n^2), as we need to fill every element in the square with the specified character.
In terms of space complexity, both hollow and filled square patterns require no space to store the characters that make up the square because we are not storing them in the memory.The space complexity is O(1).
Overall, generating these patterns can be a fun and challenging exercise for C++ programmers, and it can also help improve their problem-solving and logical thinking skills.
In this article at OpenGenus, you must have the complete idea to solve the problem "Hollow and Filled Square pattern in C++".