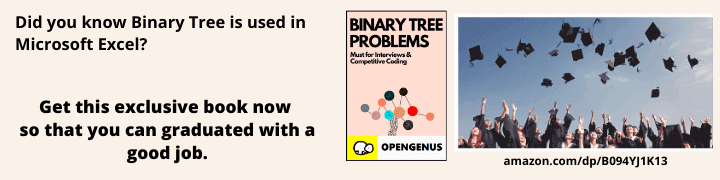
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
In this article, we will go through how to control the program flow in shell scripting using if else and switch case statements.
We will cover the following topics:
- If-Else Statement
- If-Elif-Else Statement
- Switch case
Control structures are structures that are used in decision making.
A condition is checked and different execution paths can be defined based on whether the condition is true or false.
In Shell Scripts, we have two control structures:
- If Elif Else fi
- Switch cases
1. If-Else Statement
If-else Statements in Shell Scripts act as a decision statement defining alternate execution paths based on whether the condition is true or false.
Syntax:
if [ (Condition) ]
then
Statements (executed if condition is true)...
else
Statements...
fi
- If the condition specified is true, statements in if block are executed.
- If the condition specified is false, statements in the else block are executed.
- Unlike programming languages like C++, Java etc, Shell does not use {} to define the scope of the if statement. Shell uses if to begin if else statement and fi to close it.
Code:
#!/bin/bash
read -p "Enter username: " name
if [ $name == "admin" ]
then
echo "Access Granted"
else
echo "Access Denied"
fi
Code in vi-editor:
Output:
Here, the code is executed two times:
- When input is anything other than "admin", code prints "Access Denied" in standard output.
- When input is "admin", code prints "Access Granted" in standard output.
2. If-Elif-Else Statement
When we want to check multiple conditions and define more than two alternate paths of execution based on decision, if elif (else if) and else statements are used.
Syntax:
if [ (Condition) ]
then
Statements...
elif [ (Condition) ]
then
Statements...
elif [ (Condition) ]
then
Statements...
...
else
Statements...
fi
Code:
#!/bin/bash
echo "Enter two numbers"
read num1
read num2
if [ $num1 -gt $num2 ]
then
echo "$num1 is greater than $num2"
elif [ $num1 -eq $num2 ]
then
echo "Both numbers are equal"
else
echo "$num2 is greater than $num1"
fi
- When first input is greater than second input, if statement executes.
- When both inputs are equal, elif statement executes.
- When second input is greater than first input, else statement executes.
Code in vi-editor:
Output:
Here, the code is executed three times:
- If statement executes because num1 > num2.
- Elif statement executes because num1 = num2.
- Else statement executes because num2 > num1.
3. Switch case
Switch case is used to define a set of values which define specific tasks to be performed when the iteration variable matches one of the value.
Syntax:
case {value} in
pattern/value) Statements ;;
pattern/value) Statements ;;
...
*) Default case statements ;;
esac
Here,
- ;; are used as break statement. If they are not used, all following cases will be automatically executed.
*)
is used to define the default case when no pattern/value has match with iteration variable.
Code:
#!/bin/bash
read -p "Enter your name: " name
case $name in
admin) echo "Access Granted! Welcome Admin" ;;
root) echo "Access Granted! Welcome root" ;;
*) echo "Access Denied" ;;
esac
Code in vi-editor:
Output:
Here, the code is executed three times:
- If user input is "admin", Access is granted with message Welcome Admin.
- If user input is "root", Access is granted with message Welcome root.
- For all other inputs, Access is denied.